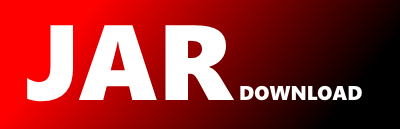
de.audioattack.io.ConsolePrintDecorator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of console Show documentation
Show all versions of console Show documentation
A replacement for java.io.Console with a System.in and System.out based fallback
/*
* SPDX-FileCopyrightText: 2021 Marc Nause
*
* SPDX-License-Identifier: Apache-2.0
*/
package de.audioattack.io;
import java.io.Flushable;
import java.io.PrintWriter;
import java.io.Reader;
import java.util.Locale;
import java.util.Objects;
/**
* Contains convenience methods which make usage of {@link Console de.audioattack.io.Console} as easy as using
* {@link java.io.PrintStream}.
*
* @since 1.1.0
*/
public class ConsolePrintDecorator implements Console, Flushable, Appendable {
private final Console console;
private final PrintWriter writer;
/**
* Constructor.
*
* @param console the console to which data will be written
*/
public ConsolePrintDecorator(final Console console) {
this.console = Objects.requireNonNull(console, "console must not be ");
this.writer = console.writer();
}
/**
* {@inheritDoc}
*/
@Override
public Reader reader() {
return console.reader();
}
/**
* {@inheritDoc}
*/
@Override
public PrintWriter writer() {
return writer;
}
/**
* Writes formatted String.
*
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return this ConsolePrintDecorator
*/
@Override
public ConsolePrintDecorator format(final String fmt, final Object... args) {
console.format(fmt, args);
return this;
}
/**
* Writes formatted String.
*
* @param locale The locale to apply during formatting. If it is {@code null} then no localization is applied.
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return this ConsolePrintDecorator
*/
public ConsolePrintDecorator format(final Locale locale, final String fmt, final Object... args) {
writer.format(locale, fmt, args);
return this;
}
/**
* Prints a boolean value.
*
* @param b boolean to be printed
*/
public void print(final boolean b) {
print(Boolean.toString(b));
}
/**
* Prints a character.
*
* @param c character to be printed
*/
public void print(final char c) {
print(Character.toString(c));
}
/**
* Prints a character array.
*
* @param s character array to be printed
*/
public void print(final char[] s) {
print(new String(s));
}
/**
* Prints a double-precision floating-point number.
*
* @param d double-precision floating-point number to be printed
*/
public void print(final double d) {
print(Double.toString(d));
}
/**
* Prints a floating-point number.
*
* @param f floating-point number to be printed
*/
public void print(final float f) {
print(Float.toString(f));
}
/**
* Prints an integer.
*
* @param i the integer to be printed
*/
public void print(final int i) {
print(Integer.toString(i));
}
/**
* Prints a long integer.
*
* @param l the long integer to be printed
*/
public void print(final long l) {
print(Long.toString(l));
}
/**
* Prints an object.
*
* @param o the object to be printed
*/
public void print(final Object o) {
print(Objects.toString(o));
}
/**
* Prints a string.
*
* @param s the string to be printed
*/
public void print(final String s) {
writer.print(s);
writer.flush();
}
/**
* Convenience method which writes formatted String.
*
* @param locale The locale to apply during formatting. If it is {@code null} then no localization is applied.
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return this ConsolePrintDecorator
*/
public ConsolePrintDecorator printf(final Locale locale, final String fmt, final Object... args) {
format(locale, fmt, args);
return this;
}
/**
* Convenience method which writes formatted String.
*
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return this ConsolePrintDecorator
*/
@Override
public ConsolePrintDecorator printf(final String fmt, final Object... args) {
console.printf(fmt, args);
return this;
}
/**
* Terminates the current line by writing the line separator string.
*/
public void println() {
writer.println();
}
/**
* Prints a boolean value and then terminates the line.
*
* @param b boolean to be printed
*/
public void println(final boolean b) {
println(Boolean.toString(b));
}
/**
* Prints a character and then terminates the line.
*
* @param c character to be printed
*/
public void println(final char c) {
println(Character.toString(c));
}
/**
* Prints a character array and then terminates the line.
*
* @param s character array to be printed
*/
public void println(final char[] s) {
println(new String(s));
}
/**
* Prints a double-precision floating-point number and then terminates the line.
*
* @param d double-precision floating-point number to be printed
*/
public void println(final double d) {
println(Double.toString(d));
}
/**
* Prints a floating-point number and then terminates the line.
*
* @param f floating-point number to be printed
*/
public void println(final float f) {
println(Float.toString(f));
}
/**
* Prints an integer and then terminates the line.
*
* @param i the integer to be printed
*/
public void println(final int i) {
println(Integer.toString(i));
}
/**
* Prints a long integer and then terminates the line.
*
* @param l the long integer to be printed
*/
public void println(final long l) {
println(Long.toString(l));
}
/**
* Prints an object and then terminates the line.
*
* @param o the object to be printed
*/
public void println(final Object o) {
println(Objects.toString(o));
}
/**
* Prints a string and then terminates the line.
*
* @param s the string to be printed
*/
public void println(final String s) {
writer.println(s);
}
/**
* {@inheritDoc}
*/
@Override
public String readLine(final String fmt, final Object... args) {
return console.readLine(fmt, args);
}
/**
* {@inheritDoc}
*/
@Override
public String readLine() {
return console.readLine();
}
/**
* {@inheritDoc}
*/
@Override
public char[] readPassword(final String fmt, final Object... args) {
return console.readPassword(fmt, args);
}
/**
* {@inheritDoc}
*/
@Override
public char[] readPassword() {
return console.readPassword();
}
/**
* Flushes this ConsoleStringDecorator by writing any buffered output.
*/
@Override
public void flush() {
writer.flush();
}
/**
* Appends the specified character sequence.
*
* @param charSequence the character sequence to append.
* If {@code charSequence} is {@code null}, then the four characters "null" are appended
*/
@Override
public ConsolePrintDecorator append(final CharSequence charSequence) {
writer.append(charSequence);
return this;
}
/**
* Appends a subsequence of the specified character sequence.
*
* @param charSequence the character sequence to append. If {@code charSequence} is {@code null}, then characters
* will be appended as if {@code charSequence} contained the four characters "null".
* @param start index of the first character in the subsequence
* @param end index of the character following the last character in the subsequence
*/
@Override
public ConsolePrintDecorator append(final CharSequence charSequence, final int start, final int end) {
writer.append(charSequence, start, end);
return this;
}
/**
* Appends the specified character.
*
* @param c the character to append
*/
@Override
public ConsolePrintDecorator append(final char c) {
writer.append(c);
return this;
}
/**
* Flushes the underlying stream and checks its error state. Error state is set to {@code true} if underlying stream
* has encountered an {@code IOException} other than .{@code InterruptedIOException}
*
* @return {@code true} if underlying stream has encountered an {@code IOException}, else {@code false}
*/
public boolean checkError() {
return writer.checkError();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy