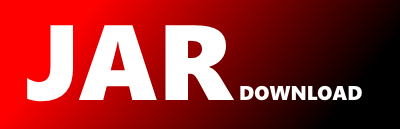
de.beyondjava.angularFaces.components.puiModelSync.PuiScriptRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of angularFaces-core Show documentation
Show all versions of angularFaces-core Show documentation
AngularFaces makes JSF programming simpler. In particular, it adds AngularJS to JSF.
/**
* (C) 2013-2014 Stephan Rauh http://www.beyondjava.net
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.beyondjava.angularFaces.components.puiModelSync;
import java.io.IOException;
import java.util.Map;
import javax.faces.application.FacesMessage;
import javax.faces.application.ProjectStage;
import javax.faces.application.Resource;
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import javax.faces.context.ResponseWriter;
import de.beyondjava.angularFaces.core.ELTools;
import de.beyondjava.angularFaces.core.i18n.I18n;
/**
* Generates a script tag that loads a script from the JSF resource folders.
* Originally copied from the Mojarra libraries.
*/
public class PuiScriptRenderer {
public void encodeScript(FacesContext context, UIComponent component, String name, String library)
throws IOException {
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy