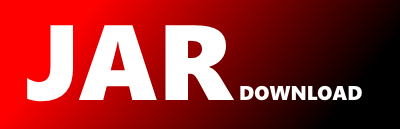
de.bigmichi1.appengine.appcfg.AppCfgUpdateMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appengine-maven-plugin Show documentation
Show all versions of appengine-maven-plugin Show documentation
A Maven Plugin for managing the Google App Engine or running the development server.
The newest version!
/*
* #%L
* A Maven Plugin for the Google App Engine
* %%
* Copyright (C) 2013 None
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package de.bigmichi1.appengine.appcfg;
import com.google.common.base.Joiner;
import org.apache.maven.plugins.annotations.Execute;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.List;
/**
* AppCfg "update" Mojo.
* Installs a new version of the application onto the server, as the default version for end users.
*
* @author Michael Cramer
* @version 1.7.5
* @since 1.7.5
*/
@Mojo(name = "update")
@Execute(phase = LifecyclePhase.PACKAGE)
public class AppCfgUpdateMojo extends AbstractBaseAppCfgMojo {
/**
* Split large jar files (> 10M) into smaller fragments.
*/
@Parameter(property = "appengine.enableJarSplitting", defaultValue = "true")
private boolean enableJarSplitting;
/**
* When {@link #enableJarSplitting} is set, files that match the list of comma separated SUFFIXES will be excluded
* from all jars.
*/
@Parameter(property = "appengine.jarSplittingExcludes")
private List jarSplittingExcludes;
/**
* Do not delete temporary (staging) directory used in uploading.
*/
@Parameter(property = "appengine.retainUploadDir", defaultValue = "false")
private boolean retainUploadDir;
/**
* The character encoding to use when compiling JSPs.
*/
@Parameter(property = "appengine.compileEncoding", defaultValue = "false")
private boolean compileEncoding;
/**
* Do not jar the classes generated from JSPs.
*/
@Parameter(property = "appengine.disableJarJsps", defaultValue = "false")
private boolean disableJarJsps;
/**
* Delete the JSP source files after compilation.
*/
@Parameter(property = "appengine.deleteJsps", defaultValue = "true")
private boolean deleteJsps;
/**
* Jar the WEB-INF/classes content.
*/
@Parameter(property = "appengine.enableJarClasses", defaultValue = "true")
private boolean enableJarClasses;
/**
* {@inheritDoc}
*/
@Nonnull
@Override
protected final String getActionName() {
return "update";
}
/**
* {@inheritDoc}
*/
@Override
protected final void addLogMessages() {
getLog().info("");
getLog().info("Google App Engine Java SDK - Create or update an app version");
getLog().info("");
}
/**
* {@inheritDoc}
*/
@Nonnull
@Override
protected final List collectActionParams() {
final List actionParams = new ArrayList(7);
if (enableJarSplitting) {
actionParams.add("--enable_jar_splitting");
}
if (enableJarSplitting && jarSplittingExcludes != null && !jarSplittingExcludes.isEmpty()) {
final String excludes = Joiner.on(',').join(jarSplittingExcludes);
actionParams.add(String.format("--jar_splitting_excludes=%s", excludes));
}
if (retainUploadDir) {
actionParams.add("--retain_upload_dir");
}
if (compileEncoding) {
actionParams.add("--compile_encoding");
}
if (disableJarJsps) {
actionParams.add("--disable_jar_jsps");
}
if (deleteJsps) {
actionParams.add("--delete_jsps");
}
if (enableJarClasses) {
actionParams.add("--enable_jar_classes");
}
return actionParams;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy