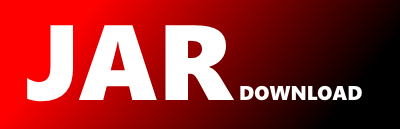
de.bwaldvogel.mongo.entity.Person Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongo-java-server-test-common Show documentation
Show all versions of mongo-java-server-test-common Show documentation
Fake implementation of MongoDB in Java that speaks the wire protocol
package de.bwaldvogel.mongo.entity;
import java.util.ArrayList;
import java.util.List;
import org.bson.types.ObjectId;
import org.springframework.data.annotation.Id;
import org.springframework.data.annotation.TypeAlias;
import org.springframework.data.mongodb.core.index.Indexed;
import org.springframework.data.mongodb.core.mapping.DBRef;
import org.springframework.data.mongodb.core.mapping.Document;
import org.springframework.data.mongodb.core.mapping.Field;
@Document
@TypeAlias("person")
public class Person {
@Id
private ObjectId id;
private String name;
@Indexed(unique = true)
@Field("ssn")
private int socialSecurityNumber;
@DBRef
private List accounts;
protected Person() {
}
public Person(String name, int socialSecurityNumber) {
this.name = name;
this.socialSecurityNumber = socialSecurityNumber;
}
public ObjectId getId() {
return id;
}
public String getName() {
return name;
}
public int getSocialSecurityNumber() {
return socialSecurityNumber;
}
public List getAccounts() {
return accounts;
}
public void addAccount(Account account) {
if (accounts == null) {
accounts = new ArrayList<>();
}
accounts.add(account);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy