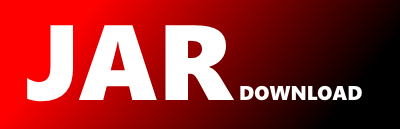
de.bytefish.pgbulkinsert.pgsql.handlers.HstoreValueHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pgbulkinsert Show documentation
Show all versions of pgbulkinsert Show documentation
PgBulkInsert is a Java library for Bulk Inserts with PostgreSQL.
The newest version!
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
package de.bytefish.pgbulkinsert.pgsql.handlers;
import de.bytefish.pgbulkinsert.util.StringUtils;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.util.Map;
public class HstoreValueHandler extends BaseValueHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy