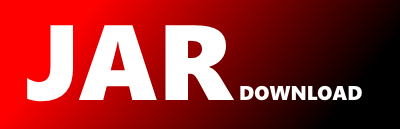
de.captaingoldfish.scim.sdk.client.http.ProxyHelper Maven / Gradle / Ivy
// Generated by delombok at Sat Oct 07 17:30:34 CEST 2023
package de.captaingoldfish.scim.sdk.client.http;
import org.apache.commons.lang3.StringUtils;
import org.apache.http.HttpHost;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.CredentialsProvider;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.impl.client.BasicCredentialsProvider;
/**
* author: Pascal Knueppel
* created at: 09.12.2019 - 12:17
*
* this helper can be used with the apache http-client to create an http client that will use a proxy.
*
* @see ScimHttpClient
*/
public class ProxyHelper
{
@java.lang.SuppressWarnings("all")
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(ProxyHelper.class);
/**
* the host under which the proxy can be reached
*/
private String systemProxyHost;
/**
* the port on the host under which the proxy can be reached
*/
private int systemProxyPort;
/**
* optional proxy username in case of proxy authentication
*/
private String systemProxyUsername;
/**
* optional proxy password in case of proxy authentication
*/
private String systemProxyPassword;
/**
* @return true if a proxy configuration is present, false else
*/
public boolean isProxySet()
{
return StringUtils.isNotBlank(systemProxyHost) && systemProxyPort != 0;
}
/**
* @return a basic credentials provider that will be used for proxy authentication.
*/
public CredentialsProvider getProxyCredentials()
{
if (StringUtils.isBlank(getSystemProxyUsername()))
{
log.trace("Proxy username is empty cannot create client credentials");
return null;
}
if (getSystemProxyPassword() == null)
{
log.debug("Proxy password is null cannot create client credentials");
return null;
}
CredentialsProvider credentialsProvider = new BasicCredentialsProvider();
credentialsProvider.setCredentials(new AuthScope(getSystemProxyHost(), systemProxyPort),
new UsernamePasswordCredentials(getSystemProxyUsername(),
getSystemProxyPassword()));
return credentialsProvider;
}
/**
* will give back a request-config with the proxy settings based on the configuration-poperties
*
* @return a new config with the configured proxy or the default-config
*/
public RequestConfig getProxyConfig()
{
if (StringUtils.isNotBlank(systemProxyHost))
{
HttpHost systemProxy = new HttpHost(systemProxyHost, systemProxyPort);
log.debug("Using proxy configuration: {}", systemProxy);
return RequestConfig.custom().setProxy(systemProxy).build();
}
return RequestConfig.DEFAULT;
}
/**
* @return the currently configured proxy settings as string in the form "localhost:8888"
*/
public String getProxyAddress()
{
return getSystemProxyHost() + ":" + getSystemProxyPort();
}
/**
* overriding lombok builder class
*/
public static class ProxyHelperBuilder
{
@java.lang.SuppressWarnings("all")
private String systemProxyHost;
@java.lang.SuppressWarnings("all")
private int systemProxyPort;
@java.lang.SuppressWarnings("all")
private String systemProxyUsername;
@java.lang.SuppressWarnings("all")
private String systemProxyPassword;
/**
* public default constructor to enable builder-inheritance
*/
public ProxyHelperBuilder()
{
super();
}
/**
* sets the proxy port
*/
public ProxyHelperBuilder systemProxyPort(int systemProxyPort)
{
this.systemProxyPort = systemProxyPort; // NOPMD
return this;
}
/**
* will parse the given string to the desired port
*
* @param systemProxyPort the port as string
* @throws IllegalArgumentException if the port is empty or does not match a valid number
*/
public ProxyHelperBuilder systemProxyPort(String systemProxyPort)
{
if (StringUtils.isBlank(systemProxyPort) || !systemProxyPort.matches("\\d+"))
{
throw new IllegalArgumentException("Port must not be empty and must contain only numbers but is: "
+ systemProxyPort + "\n Set Port to \'0\' if proxy is not required.");
}
this.systemProxyPort = Integer.parseInt(systemProxyPort); // NOPMD
return this;
}
/**
* the host under which the proxy can be reached
*
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProxyHelper.ProxyHelperBuilder systemProxyHost(final String systemProxyHost)
{
this.systemProxyHost = systemProxyHost;
return this;
}
/**
* optional proxy username in case of proxy authentication
*
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProxyHelper.ProxyHelperBuilder systemProxyUsername(final String systemProxyUsername)
{
this.systemProxyUsername = systemProxyUsername;
return this;
}
/**
* optional proxy password in case of proxy authentication
*
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProxyHelper.ProxyHelperBuilder systemProxyPassword(final String systemProxyPassword)
{
this.systemProxyPassword = systemProxyPassword;
return this;
}
@java.lang.SuppressWarnings("all")
public ProxyHelper build()
{
return new ProxyHelper(this.systemProxyHost, this.systemProxyPort, this.systemProxyUsername,
this.systemProxyPassword);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString()
{
return "ProxyHelper.ProxyHelperBuilder(systemProxyHost=" + this.systemProxyHost + ", systemProxyPort="
+ this.systemProxyPort + ", systemProxyUsername=" + this.systemProxyUsername + ", systemProxyPassword="
+ this.systemProxyPassword + ")";
}
}
@java.lang.SuppressWarnings("all")
public static ProxyHelper.ProxyHelperBuilder builder()
{
return new ProxyHelper.ProxyHelperBuilder();
}
/**
* the host under which the proxy can be reached
*/
@java.lang.SuppressWarnings("all")
public String getSystemProxyHost()
{
return this.systemProxyHost;
}
/**
* the port on the host under which the proxy can be reached
*/
@java.lang.SuppressWarnings("all")
public int getSystemProxyPort()
{
return this.systemProxyPort;
}
/**
* optional proxy username in case of proxy authentication
*/
@java.lang.SuppressWarnings("all")
public String getSystemProxyUsername()
{
return this.systemProxyUsername;
}
/**
* optional proxy password in case of proxy authentication
*/
@java.lang.SuppressWarnings("all")
public String getSystemProxyPassword()
{
return this.systemProxyPassword;
}
/**
* the host under which the proxy can be reached
*/
@java.lang.SuppressWarnings("all")
public void setSystemProxyHost(final String systemProxyHost)
{
this.systemProxyHost = systemProxyHost;
}
/**
* the port on the host under which the proxy can be reached
*/
@java.lang.SuppressWarnings("all")
public void setSystemProxyPort(final int systemProxyPort)
{
this.systemProxyPort = systemProxyPort;
}
/**
* optional proxy username in case of proxy authentication
*/
@java.lang.SuppressWarnings("all")
public void setSystemProxyUsername(final String systemProxyUsername)
{
this.systemProxyUsername = systemProxyUsername;
}
/**
* optional proxy password in case of proxy authentication
*/
@java.lang.SuppressWarnings("all")
public void setSystemProxyPassword(final String systemProxyPassword)
{
this.systemProxyPassword = systemProxyPassword;
}
@java.lang.SuppressWarnings("all")
public ProxyHelper(final String systemProxyHost,
final int systemProxyPort,
final String systemProxyUsername,
final String systemProxyPassword)
{
this.systemProxyHost = systemProxyHost;
this.systemProxyPort = systemProxyPort;
this.systemProxyUsername = systemProxyUsername;
this.systemProxyPassword = systemProxyPassword;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy