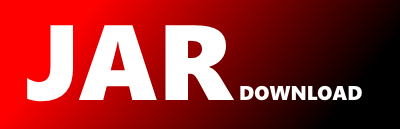
de.captaingoldfish.scim.sdk.client.builder.config.MetaConfigRequestDetails Maven / Gradle / Ivy
// Generated by delombok at Tue Oct 15 15:19:09 CEST 2024
package de.captaingoldfish.scim.sdk.client.builder.config;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import de.captaingoldfish.scim.sdk.common.constants.EndpointPaths;
import de.captaingoldfish.scim.sdk.common.constants.ResourceTypeNames;
import de.captaingoldfish.scim.sdk.common.constants.SchemaUris;
/**
* @author Pascal Knueppel
* @since 14.10.2023
*/
public class MetaConfigRequestDetails
{
public static final List DEFAULT_META_SCHEMA_URIS = Arrays.asList(SchemaUris.META,
SchemaUris.SERVICE_PROVIDER_CONFIG_URI,
SchemaUris.RESOURCE_TYPE_URI,
SchemaUris.RESOURCE_TYPE_FEATURE_EXTENSION_URI,
SchemaUris.SCHEMA_URI);
public static final List DEFAULT_META_RESOURCE_TYPES_NAMES = Arrays.asList(ResourceTypeNames.SERVICE_PROVIDER_CONFIG,
ResourceTypeNames.RESOURCE_TYPE,
ResourceTypeNames.SCHEMA);
/**
* the endpoint where the ServiceProviderConfig can be found
*/
private final String serviceProviderEndpoint;
/**
* the endpoint where the ResourceTypes can be found
*/
private final String resourceTypeEndpoint;
/**
* the endpoint where the Schemas can be found
*/
private final String schemasEndpoint;
/**
* if the meta-schemas should be excluded
*/
private final boolean excludeMetaSchemas;
/**
* if the meta-ResourceTypes should be excluded
*/
private final boolean excludeMetaResourceTypes;
/**
* the meta-ResourceTypes. This field is only used if the field {@link #excludeMetaResourceTypes} is true
*/
private final List metaResourceTypeNames;
/**
* the meta-Schemas. This field is only used if the field {@link #excludeMetaSchemas} is true
*/
private final List metaSchemaUris;
public MetaConfigRequestDetails()
{
this.serviceProviderEndpoint = EndpointPaths.SERVICE_PROVIDER_CONFIG;
this.resourceTypeEndpoint = EndpointPaths.RESOURCE_TYPES;
this.schemasEndpoint = EndpointPaths.SCHEMAS;
this.excludeMetaSchemas = false;
this.excludeMetaResourceTypes = false;
this.metaResourceTypeNames = DEFAULT_META_RESOURCE_TYPES_NAMES;
this.metaSchemaUris = DEFAULT_META_SCHEMA_URIS;
}
public MetaConfigRequestDetails(String serviceProviderEndpoint,
String resourceTypeEndpoint,
String schemasEndpoint,
boolean excludeMetaSchemas,
boolean excludeMetaResourceTypes,
List metaSchemaUris,
List metaResourceTypeNames)
{
this.serviceProviderEndpoint = Optional.ofNullable(serviceProviderEndpoint)
.orElse(EndpointPaths.SERVICE_PROVIDER_CONFIG);
this.resourceTypeEndpoint = Optional.ofNullable(resourceTypeEndpoint).orElse(EndpointPaths.RESOURCE_TYPES);
this.schemasEndpoint = Optional.ofNullable(schemasEndpoint).orElse(EndpointPaths.SCHEMAS);
this.excludeMetaSchemas = excludeMetaSchemas;
this.excludeMetaResourceTypes = excludeMetaResourceTypes;
this.metaSchemaUris = Optional.ofNullable(metaSchemaUris).orElse(DEFAULT_META_SCHEMA_URIS);
this.metaResourceTypeNames = Optional.ofNullable(metaResourceTypeNames).orElse(DEFAULT_META_RESOURCE_TYPES_NAMES);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static class MetaConfigRequestDetailsBuilder
{
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String serviceProviderEndpoint;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String resourceTypeEndpoint;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private String schemasEndpoint;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private boolean excludeMetaSchemas;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private boolean excludeMetaResourceTypes;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List metaSchemaUris;
@java.lang.SuppressWarnings("all")
@lombok.Generated
private List metaResourceTypeNames;
@java.lang.SuppressWarnings("all")
@lombok.Generated
MetaConfigRequestDetailsBuilder()
{}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder serviceProviderEndpoint(final String serviceProviderEndpoint)
{
this.serviceProviderEndpoint = serviceProviderEndpoint;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder resourceTypeEndpoint(final String resourceTypeEndpoint)
{
this.resourceTypeEndpoint = resourceTypeEndpoint;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder schemasEndpoint(final String schemasEndpoint)
{
this.schemasEndpoint = schemasEndpoint;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder excludeMetaSchemas(final boolean excludeMetaSchemas)
{
this.excludeMetaSchemas = excludeMetaSchemas;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder excludeMetaResourceTypes(final boolean excludeMetaResourceTypes)
{
this.excludeMetaResourceTypes = excludeMetaResourceTypes;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder metaSchemaUris(final List metaSchemaUris)
{
this.metaSchemaUris = metaSchemaUris;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder metaResourceTypeNames(final List metaResourceTypeNames)
{
this.metaResourceTypeNames = metaResourceTypeNames;
return this;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public MetaConfigRequestDetails build()
{
return new MetaConfigRequestDetails(this.serviceProviderEndpoint, this.resourceTypeEndpoint, this.schemasEndpoint,
this.excludeMetaSchemas, this.excludeMetaResourceTypes, this.metaSchemaUris,
this.metaResourceTypeNames);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public java.lang.String toString()
{
return "MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder(serviceProviderEndpoint="
+ this.serviceProviderEndpoint + ", resourceTypeEndpoint=" + this.resourceTypeEndpoint
+ ", schemasEndpoint=" + this.schemasEndpoint + ", excludeMetaSchemas=" + this.excludeMetaSchemas
+ ", excludeMetaResourceTypes=" + this.excludeMetaResourceTypes + ", metaSchemaUris=" + this.metaSchemaUris
+ ", metaResourceTypeNames=" + this.metaResourceTypeNames + ")";
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public static MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder builder()
{
return new MetaConfigRequestDetails.MetaConfigRequestDetailsBuilder();
}
/**
* the endpoint where the ServiceProviderConfig can be found
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getServiceProviderEndpoint()
{
return this.serviceProviderEndpoint;
}
/**
* the endpoint where the ResourceTypes can be found
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getResourceTypeEndpoint()
{
return this.resourceTypeEndpoint;
}
/**
* the endpoint where the Schemas can be found
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSchemasEndpoint()
{
return this.schemasEndpoint;
}
/**
* if the meta-schemas should be excluded
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean isExcludeMetaSchemas()
{
return this.excludeMetaSchemas;
}
/**
* if the meta-ResourceTypes should be excluded
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean isExcludeMetaResourceTypes()
{
return this.excludeMetaResourceTypes;
}
/**
* the meta-ResourceTypes. This field is only used if the field {@link #excludeMetaResourceTypes} is true
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getMetaResourceTypeNames()
{
return this.metaResourceTypeNames;
}
/**
* the meta-Schemas. This field is only used if the field {@link #excludeMetaSchemas} is true
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getMetaSchemaUris()
{
return this.metaSchemaUris;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy