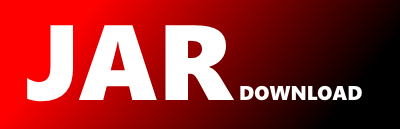
de.citec.scie.util.ResourceFinder Maven / Gradle / Ivy
/*
* SCIE -- Spinal Cord Injury Information Extraction
* Copyright (C) 2013, 2014
* Raphael Dickfelder, Jan Göpfert, Benjamin Paaßen, Andreas Stöckel
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package de.citec.scie.util;
import java.io.File;
import java.io.IOException;
/**
* The ResourceFinder class is responsible for finding certain resources. This
* class is mainly needed because we want the scie-core subproject to run from
* its own sub folder yet allow to store the data in the top-level project. To
* do so, the ResourceFinder traverses upwards the complete directory tree. If
* you run in an environment where this might be a security threat or you don't
* want this application to search for files in locations it shouldn't simply
* provide the scie.noascend property to true, e.g. via the
* -Dscie.noascend="true" Java CLI switch.
*
* @author Andreas Stöckel -- [email protected]
*/
public class ResourceFinder {
/**
* If true, ascends the complete directory tree upwards to search for the
* specified files.
*/
private static final boolean ASCEND
= !System.getProperty("scie.noascend", "false").equalsIgnoreCase("true");
/**
* Exception thrown whenever a requested file is not found.
*/
public static class ResourceFinderException extends RuntimeException {
/**
* Constructor of the ResourceFinderException class.
*
* @param msg message describing the exception.
* @param cause cause of the exception or null.
*/
public ResourceFinderException(String msg, Throwable cause) {
super(msg, cause);
}
}
/**
* Tries to find a file with the given filename. Throws a
* ResourceFinderException if the file is not found. This function ascends
* the directory tree until the file is found (unless -Dscie.noascend="true"
* is set).
*
* @param name is the name of the file to find. The filename should not
* start with a "/".
* @param path is an array of subdirectories/prefixes in which the given
* file should be searched. The paths should end with a "/". If null or an
* empty array is given, the prefix "./" is used. Paths and filename are
* joined using the "parent", "child" version of the File constructor.
* @return a File instance pointing at the found file.
*/
public static File find(String name, String[] path) {
// Search at least in the current directory
if (path == null || path.length == 0) {
path = new String[]{"./"};
}
try {
final String wd = (new File(".")).getCanonicalPath();
File curDir = new File(wd);
do {
for (String p : path) {
final File f = new File(curDir, (new File(p, name)).getPath());
if (f.exists()) {
final String fp = f.getCanonicalPath();
//System.err.println("Found " + name + " at " + fp);
return new File(fp);
}
}
curDir = curDir.getParentFile();
} while (ASCEND && curDir != null && !curDir.getPath().equals("/"));
} catch (IOException ex) {
throw new ResourceFinderException(ex.getMessage(), ex);
}
throw new RuntimeException("File " + name
+ " not found in this or the parent directories!", null);
}
/**
* Tries to find a file with the given filename. Throws a
* ResourceFinderException if the file is not found. This function ascends
* the directory tree until the file is found (unless -Dscie.noascend="true"
* is set).
*
* @param name is the name of the file to find. The filename should not
* start with a "/".
* @return a file
*/
public static File find(String name) {
return find(name, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy