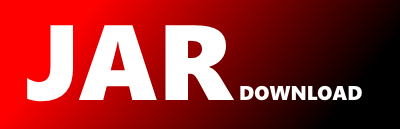
de.citec.tcs.alignment.ParallelProcessingEngine Maven / Gradle / Ivy
/*
* TCS Alignment Toolbox
*
* Copyright (C) 2013-2015
* Benjamin Paaßen, Georg Zentgraf
* AG Theoretical Computer Science
* Centre of Excellence Cognitive Interaction Technology (CITEC)
* University of Bielefeld
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package de.citec.tcs.alignment;
import de.citec.tcs.alignment.parallel.MatrixEngine;
import de.citec.tcs.alignment.sequence.Sequence;
import java.util.concurrent.Callable;
/**
* This allows parallel processing of alignment calculations.
*
* @param The result type of the alignment algorithm.
*
* @author Benjamin Paassen - [email protected]
*/
public class ParallelProcessingEngine extends MatrixEngine {
private final AlignmentAlgorithm< R> algorithm;
private final Sequence[] sequences;
/**
* This sets up a ParallelProcessingEngine for the given AlignmentAlgorithm
* and the given Sequences.
*
* @param algorithm an AlignmentAlgorithm of your choice.
* @param sequences an array of Sequences. Per default the
* ParallelProcessingEngine will calculate all pairwise alignments between
* them.
*/
public ParallelProcessingEngine(AlignmentAlgorithm< R> algorithm, Sequence[] sequences) {
super(sequences.length, sequences.length, algorithm.getResultClass());
this.algorithm = algorithm;
this.sequences = sequences;
}
/**
*
* @return The AlignmentAlgorithm that was given in the constructor.
*/
public AlignmentAlgorithm< R> getAlgorithm() {
return algorithm;
}
/**
*
* @return The Sequence instances that were given in the constructor.
*/
public Sequence[] getSequences() {
return sequences;
}
/**
* {@inheritDoc }
*/
@Override
public Callable createCallable(MatrixCoordinate ident) {
return new AlignmentCallable(ident.i, ident.j);
}
private class AlignmentCallable implements Callable {
private final int leftIdx;
private final int rightIdx;
public AlignmentCallable(int leftIdx, int rightIdx) {
this.leftIdx = leftIdx;
this.rightIdx = rightIdx;
}
@Override
public R call() throws Exception {
return algorithm.calculateAlignment(sequences[leftIdx], sequences[rightIdx]);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy