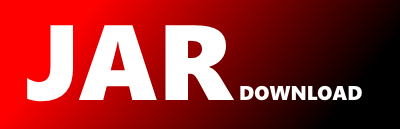
de.codecamp.messages.shared.conf.BundleMapping Maven / Gradle / Ivy
package de.codecamp.messages.shared.conf;
import java.util.Objects;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang3.StringUtils;
public class BundleMapping
{
public static final BundleMapping IMPORTS_PLACEHOLDER = new BundleMapping(null, null);
private static final Pattern MATCH_ALL = Pattern.compile(".*");
private final String messageKeyPattern;
// should not be serialized into message key index
private transient Pattern messageKeyPatternRegex;
private final String bundleNamePattern;
public BundleMapping(String messageKeyPattern, String bundleNamePattern)
{
this.messageKeyPattern = messageKeyPattern;
this.bundleNamePattern = bundleNamePattern;
}
/**
* Return the message key pattern.
*
* @return the message key pattern
*/
public String getMessageKeyPattern()
{
return messageKeyPattern;
}
public static void main(String[] args)
{
Pattern regexPattern = toRegexPattern("de.codecamp");
Matcher matcher = regexPattern.matcher("de.codecamp.app.model.Type");
System.out.println(matcher.matches());
System.out.println(matcher.groupCount());
if (matcher.groupCount() >= 1)
{
String bla = matcher.group(1);
bla = StringUtils.removeStart(bla, ".");
System.out.println(bla);
}
}
public Pattern getMessageKeyPatternAsRegex()
{
if (messageKeyPatternRegex == null)
messageKeyPatternRegex = toRegexPattern(messageKeyPattern);
return messageKeyPatternRegex;
}
/**
* Return the target bundle name.
*
* @return the target bundle name
*/
public String getBundleNamePattern()
{
return bundleNamePattern;
}
@Override
public int hashCode()
{
final int prime = 31;
int result = 1;
result = prime * result + ((messageKeyPattern == null) ? 0 : messageKeyPattern.hashCode());
result = prime * result + ((bundleNamePattern == null) ? 0 : bundleNamePattern.hashCode());
return result;
}
@Override
public boolean equals(Object obj)
{
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
BundleMapping other = (BundleMapping) obj;
if (!Objects.equals(messageKeyPattern, other.messageKeyPattern))
return false;
if (!Objects.equals(bundleNamePattern, other.bundleNamePattern))
return false;
return true;
}
private static Pattern toRegexPattern(String messageKeyPattern)
{
if (messageKeyPattern.isEmpty())
return MATCH_ALL;
StringBuilder patternBuilder = new StringBuilder();
patternBuilder.append("^");
boolean firstSegment = true;
for (String segment : messageKeyPattern.split("\\."))
{
if (firstSegment)
firstSegment = false;
else
patternBuilder.append(Pattern.quote("."));
// split segments around any number of "*", while also keeping the delimiter
for (String token : segment.split("(?<=\\*+)(?!\\*)|(?
© 2015 - 2025 Weber Informatics LLC | Privacy Policy