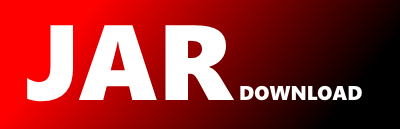
de.codecamp.messages.shared.messageformat.MessageFormatSupport Maven / Gradle / Ivy
package de.codecamp.messages.shared.messageformat;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.time.OffsetTime;
import java.time.ZonedDateTime;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import com.ibm.icu.util.CurrencyAmount;
import de.codecamp.messages.MessageKeyWithArgs;
import de.codecamp.messages.shared.conf.ProjectConf;
public interface MessageFormatSupport
{
boolean supportsFormat(String otherMessageFormat);
boolean hasArgNameSupport();
/**
* Formats the given Java-type of a message argument to something less technical where possible.
*
* @param argType
* the Java-type of the message argument
* @return the formatted message argument type
*/
default String formatArgType(String argType)
{
if (String.class.getName().equals(argType))
{
return "text";
}
else if (byte.class.getName().equals(argType) || Byte.class.getName().equals(argType)
|| short.class.getName().equals(argType) || Short.class.getName().equals(argType)
|| int.class.getName().equals(argType) || Integer.class.getName().equals(argType)
|| long.class.getName().equals(argType) || Long.class.getName().equals(argType)
|| BigInteger.class.getName().equals(argType))
{
return "integer";
}
else if (float.class.getName().equals(argType) || Float.class.getName().equals(argType)
|| double.class.getName().equals(argType) || Double.class.getName().equals(argType)
|| BigDecimal.class.getName().equals(argType))
{
return "decimal";
}
else if (Date.class.getName().equals(argType) || Calendar.class.getName().equals(argType)
|| LocalDateTime.class.getName().equals(argType)
|| ZonedDateTime.class.getName().equals(argType)
|| OffsetDateTime.class.getName().equals(argType)
|| Instant.class.getName().equals(argType))
{
return "date/time";
}
else if (LocalDate.class.getName().equals(argType))
{
return "date";
}
else if (LocalTime.class.getName().equals(argType)
|| OffsetTime.class.getName().equals(argType))
{
return "time";
}
else if (CurrencyAmount.class.getName().equals(argType))
{
return "currency";
}
else
{
return StringUtils.removeStart(argType, "java.lang.");
}
}
List getArgInsertOptions(MessageKeyWithArgs key);
String createMessageBundleComment(MessageKeyWithArgs key);
List checkMessage(String message, String[] argTypes, String[] argNames,
TypeChecker argTypeChecker);
interface TypeChecker
{
boolean isCompatibleWith(String typeName, Set allowedTypeNames);
}
static MessageFormatSupport getSupport(ProjectConf projectConf)
{
MessageFormatSupport argsSupport;
if (projectConf.getMessageFormat().equals(IcuMessageFormatSupport.ID))
{
argsSupport = new IcuMessageFormatSupport();
}
else if (projectConf.getMessageFormat().equals(DefaultMessageFormatSupport.ID))
{
argsSupport = new DefaultMessageFormatSupport();
}
else
{
throw new RuntimeException(
String.format("Unknown message formatter: %s", projectConf.getMessageFormat()));
}
return argsSupport;
}
public static class ArgInsert
{
private final String label;
private final String reference;
public ArgInsert(String label, String reference)
{
this.label = label;
this.reference = reference;
}
public String getLabel()
{
return label;
}
public String getReference()
{
return reference;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy