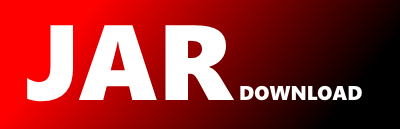
de.codecamp.messages.shared.bundle.NioFileSystemAdapter Maven / Gradle / Ivy
package de.codecamp.messages.shared.bundle;
import static java.util.stream.Collectors.toList;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.NoSuchFileException;
import java.nio.file.NotDirectoryException;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.util.Collections;
import java.util.List;
import java.util.stream.Stream;
public class NioFileSystemAdapter
implements
FileSystemAdapter
{
@Override
public Path getDirectory(String directoryPath)
{
return Paths.get(directoryPath);
}
@Override
public List listFiles(Path dir, boolean recursive)
throws IOException
{
try (Stream pathStream = (recursive ? Files.walk(dir) : Files.list(dir)))
{
return pathStream.filter(Files::isRegularFile).collect(toList());
}
catch (NoSuchFileException | NotDirectoryException ex)
{
return Collections.emptyList();
}
}
@Override
public Path getFile(Path dir, String fileName)
{
return dir.resolve(fileName);
}
@Override
public String getFileName(Path file)
{
return file.getFileName().toString();
}
@Override
public String getRelativeFilePath(Path dir, Path file)
{
return dir.relativize(file).toString();
}
@Override
public String getDisplayPath(Path dir, Path file)
{
return dir.relativize(file).toString();
}
@Override
public boolean exists(Path file)
{
return Files.exists(file);
}
@Override
public void createParentDirectories(Path file)
throws IOException
{
Path bundleDir = file.getParent();
Files.createDirectories(bundleDir);
}
@Override
public void deleteIfExists(Path file)
throws IOException
{
Files.deleteIfExists(file);
}
@Override
public InputStream newInputStream(Path file)
throws IOException
{
return Files.newInputStream(file);
}
@Override
public OutputStream newOutputStream(Path file)
throws IOException
{
return Files.newOutputStream(file, StandardOpenOption.CREATE,
StandardOpenOption.TRUNCATE_EXISTING);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy