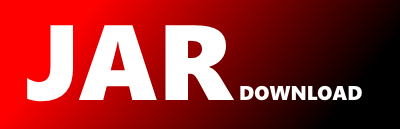
de.codecamp.messages.spring.ExtendedMessageSource Maven / Gradle / Ivy
package de.codecamp.messages.spring;
import de.codecamp.messages.ResolvableMessage;
import de.codecamp.messages.spring.impl.TimeZonePassThrough;
import java.time.ZoneId;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import org.springframework.context.MessageSource;
import org.springframework.context.NoSuchMessageException;
import org.springframework.lang.Nullable;
/**
* An extension of {@link MessageSource} that adds the following:
*
* - A list of all available locales actually provided by the application (explicitly configured
* or autodiscovered).
* - An explicit default locale for the application (explicitly configured or automatically chosen
* from the available locales) as replacement to using the system locale.
* - Support for named arguments in messages.
*
*/
public interface ExtendedMessageSource
extends
MessageSource
{
/**
* Returns all locales available in the application.
*
* @return all locales available in the application
*/
Set getAvailableLocales();
/**
* Returns the default locale of the application that will be used when none of the requested
* locales can be found.
*
* @return the default locale of the application
*/
Locale getDefaultLocale();
/**
* Returns the default time zone of the application that will be used when no other time zone is
* specified.
*
* @return the default time zone of the application
*/
ZoneId getDefaultTimeZone();
/**
* Sets the default time zone of the application that will be used when no other time zone is
* specified.
*
* @param defaultTimeZone
* the default time zone of the application
*/
void setDefaultTimeZone(ZoneId defaultTimeZone);
/**
* Try to resolve the message.
*
* @param code
* the code of the message key to look up
* @param args
* the arguments to format the message with
* @param locale
* the locale in which to do the lookup
* @param timeZone
* the time zone used for formatting
* @return the resolved message (never {@code null})
* @throws NoSuchMessageException
* if no corresponding message was found
*/
default String getMessage(String code, @Nullable Object[] args, Locale locale, ZoneId timeZone)
{
return TimeZonePassThrough.withTimeZone(timeZone, () -> getMessage(code, args, locale));
}
/**
* Try to resolve the message.
*
* @param code
* the code of the message key to look up
* @param args
* the arguments to format the message with
* @param locale
* the locale in which to do the lookup
* @param timeZone
* the time zone used for formatting
* @return the resolved message (never {@code null})
* @throws NoSuchMessageException
* if no corresponding message was found
*/
default String getMessage(String code, @Nullable Map args,
@Nullable Locale locale, @Nullable ZoneId timeZone)
{
return TimeZonePassThrough.withTimeZone(timeZone,
() -> getMessage(code, new Object[] {args}, locale));
}
/**
* Try to resolve the message.
*
* @param code
* the code of the message key to look up
* @param args
* the arguments to format the message with
* @param defaultMessage
* a default message to return if the lookup fails
* @param locale
* the locale in which to do the lookup
* @param timeZone
* the time zone used for formatting
* @return the resolved message if the lookup was successful, otherwise the default message passed
* as a parameter (which may be {@code null})
*/
@Nullable
default String getMessage(String code, @Nullable Map args,
@Nullable String defaultMessage, @Nullable Locale locale, @Nullable ZoneId timeZone)
{
return TimeZonePassThrough.withTimeZone(timeZone,
() -> getMessage(code, new Object[] {args}, defaultMessage, locale));
}
/**
* Try to resolve the message.
*
* @param resolvable
* the resolvable message
* @param locale
* the locale in which to do the lookup
* @param timeZone
* the time zone used for formatting
* @return the resolved message (never {@code null})
* @throws NoSuchMessageException
* if no corresponding message was found
*/
default String getMessage(ResolvableMessage resolvable, @Nullable Locale locale,
@Nullable ZoneId timeZone)
{
return TimeZonePassThrough.withTimeZone(timeZone,
() -> getMessage(resolvable.getCode(), new Object[] {resolvable}, locale));
}
/**
* Try to resolve the message.
*
* @param resolvable
* the resolvable message
* @param defaultMessage
* a default message to return if the lookup fails
* @param locale
* the locale in which to do the lookup
* @param timeZone
* the time zone used for formatting
* @return the resolved message if the lookup was successful, otherwise the default message passed
* as a parameter (which may be {@code null})
*/
@Nullable
default String getMessage(ResolvableMessage resolvable, @Nullable String defaultMessage,
@Nullable Locale locale, @Nullable ZoneId timeZone)
{
return TimeZonePassThrough.withTimeZone(timeZone,
() -> getMessage(resolvable.getCode(), new Object[] {resolvable}, defaultMessage, locale));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy