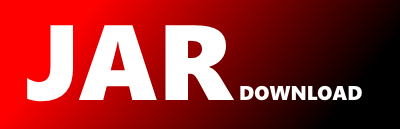
org.mule.modules.ftpclient.generated.sources.PollWithArchivingByRenamingMessageSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ftp-client-connector Show documentation
Show all versions of ftp-client-connector Show documentation
A Mule connector for ftp/sftp.
package org.mule.modules.ftpclient.generated.sources;
import java.util.List;
import javax.annotation.Generated;
import org.mule.api.MuleEvent;
import org.mule.api.MuleException;
import org.mule.api.callback.SourceCallback;
import org.mule.api.construct.FlowConstructAware;
import org.mule.api.context.MuleContextAware;
import org.mule.api.devkit.ProcessAdapter;
import org.mule.api.devkit.ProcessTemplate;
import org.mule.api.lifecycle.InitialisationException;
import org.mule.api.lifecycle.Startable;
import org.mule.api.lifecycle.Stoppable;
import org.mule.api.source.ClusterizableMessageSource;
import org.mule.modules.ftpclient.FtpClientConnector;
import org.mule.security.oauth.callback.ProcessCallback;
import org.mule.security.oauth.processor.AbstractListeningMessageProcessor;
/**
* PollWithArchivingByRenamingMessageSource wraps {@link org.mule.modules.ftpclient.FtpClientConnector#pollWithArchivingByRenaming(java.lang.String, java.lang.String, java.lang.String, java.lang.String, java.lang.String, boolean, org.mule.api.callback.SourceCallback)} method in {@link FtpClientConnector } as a message source capable of generating Mule events. The POJO's method is invoked in its own thread.
*
*/
@SuppressWarnings("all")
@Generated(value = "Mule DevKit Version 3.9.0", date = "2018-04-30T04:51:01+02:00", comments = "Build UNNAMED.2793.f49b6c7")
public class PollWithArchivingByRenamingMessageSource
extends AbstractListeningMessageProcessor
implements Runnable, FlowConstructAware, MuleContextAware, Startable, Stoppable, ClusterizableMessageSource
{
protected Object directory;
protected String _directoryType;
protected Object filename;
protected String _filenameType;
protected Object translatedNameExpression;
protected String _translatedNameExpressionType;
protected Object filenameExpression;
protected String _filenameExpressionType;
protected Object originalFilenameExpression;
protected String _originalFilenameExpressionType;
protected Object streaming;
protected boolean _streamingType;
/**
* Thread under which this message source will execute
*
*/
private java.lang.Thread thread;
/**
* Polling Period for SourceStrategy.POLLING
*
*/
static Long pollingPeriod;
public PollWithArchivingByRenamingMessageSource(String operationName) {
super(operationName);
}
/**
* Sets pollingPeriod
*
* @param value Value to set
*/
public void setPollingPeriod(Long value) {
this.pollingPeriod = value;
}
/**
* Obtains the expression manager from the Mule context and initialises the connector. If a target object has not been set already it will search the Mule registry for a default one.
*
* @throws InitialisationException
*/
public void initialise()
throws InitialisationException
{
}
/**
* Sets streaming
*
* @param value Value to set
*/
public void setStreaming(Object value) {
this.streaming = value;
}
/**
* Sets filename
*
* @param value Value to set
*/
public void setFilename(Object value) {
this.filename = value;
}
/**
* Sets translatedNameExpression
*
* @param value Value to set
*/
public void setTranslatedNameExpression(Object value) {
this.translatedNameExpression = value;
}
/**
* Sets originalFilenameExpression
*
* @param value Value to set
*/
public void setOriginalFilenameExpression(Object value) {
this.originalFilenameExpression = value;
}
/**
* Sets filenameExpression
*
* @param value Value to set
*/
public void setFilenameExpression(Object value) {
this.filenameExpression = value;
}
/**
* Sets directory
*
* @param value Value to set
*/
public void setDirectory(Object value) {
this.directory = value;
}
/**
* Method to be called when Mule instance gets started.
*
*/
public void start()
throws MuleException
{
if (thread == null) {
thread = new java.lang.Thread(this, "Receiving Thread");
}
thread.start();
}
/**
* Method to be called when Mule instance gets stopped.
*
*/
public void stop()
throws MuleException
{
thread.interrupt();
}
/**
* Implementation {@link Runnable#run()} that will invoke the method on the pojo that this message source wraps.
*
*/
public void run() {
Object moduleObject = null;
try {
moduleObject = findOrCreate(null, false, null);
final ProcessTemplate
© 2015 - 2024 Weber Informatics LLC | Privacy Policy