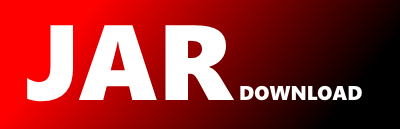
de.placeblock.commandapi.core.SuggestionBuilder Maven / Gradle / Ivy
package de.placeblock.commandapi.core;
import de.placeblock.commandapi.core.parser.ParameterHolder;
import de.placeblock.commandapi.core.tree.ParameterTreeCommand;
import de.placeblock.commandapi.core.tree.TreeCommand;
import lombok.Getter;
import lombok.RequiredArgsConstructor;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
@Getter
@RequiredArgsConstructor
@SuppressWarnings("unused")
public class SuggestionBuilder extends ParameterHolder {
private final TreeCommand treeCommand;
private final String remaining;
private final S source;
private final List suggestions = new ArrayList<>();
public SuggestionBuilder(TreeCommand treeCommand, String remaining, S source, Map, Object> parsedParameters) {
super(parsedParameters);
this.treeCommand = treeCommand;
this.remaining = remaining;
this.source = source;
}
@SuppressWarnings("UnusedReturnValue")
public SuggestionBuilder withSuggestion(String suggestion) {
this.suggestions.add(suggestion);
return this;
}
public void withSuggestions(List suggestions) {
this.suggestions.addAll(suggestions);
}
public void withSuggestions(String[] suggestions) {
this.suggestions.addAll(List.of(suggestions));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy