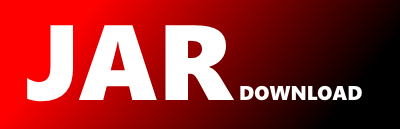
de.comhix.commons.updater.Updater Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of updater Show documentation
Show all versions of updater Show documentation
Base for updating applications
package de.comhix.commons.updater;
import com.google.gson.GsonBuilder;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import okio.BufferedSink;
import okio.Okio;
import java.io.*;
import java.util.function.Function;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
/**
* @author Benjamin Beeker
*/
public class Updater> {
private final String url;
private final Function updateUrlProvider;
private final Function versionProvider;
private final Info currentVersion;
private Info info;
private Updater(String url,
Info currentVersion,
Function updateUrlProvider,
Function versionProvider) {
this.url = url;
this.updateUrlProvider = updateUrlProvider;
this.versionProvider = versionProvider;
this.currentVersion = currentVersion;
}
public static > Updater create(String url,
Info currentVersion,
Function updateUrlProvider,
Function versionProvider) {
return new Updater<>(url, currentVersion, updateUrlProvider, versionProvider);
}
InputStream loadInfo() throws IOException {
OkHttpClient client = new OkHttpClient.Builder().build();
Response response = client.newCall(new Request.Builder().url(url).build()).execute();
return response.body().byteStream();
}
private Info getInfo() throws IOException {
if (info == null) {
info = new GsonBuilder().create().fromJson(new InputStreamReader(loadInfo()),
(Class) currentVersion.getClass());
}
return info;
}
public boolean hasUpdate() throws IOException {
Info info = getInfo();
return versionProvider.apply(currentVersion)
.compareTo(versionProvider.apply(info)) < 0;
}
public void doUpdate() throws IOException {
OkHttpClient client = new OkHttpClient.Builder().build();
Response response = client.newCall(new Request.Builder().url(updateUrlProvider.apply(info)).build()).execute();
try (BufferedSink output = Okio.buffer(Okio.sink(new File("update.zip")))) {
output.writeAll(Okio.source(response.body().byteStream()));
}
byte[] buffer = new byte[1024];
ZipInputStream zis = new ZipInputStream(new FileInputStream("update.zip"));
ZipEntry zipEntry = zis.getNextEntry();
while (zipEntry != null) {
String fileName = zipEntry.getName();
File newFile = new File(fileName);
FileOutputStream fos = new FileOutputStream(newFile);
int len;
while ((len = zis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
zipEntry = zis.getNextEntry();
}
zis.closeEntry();
zis.close();
new File("update.zip").deleteOnExit();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy