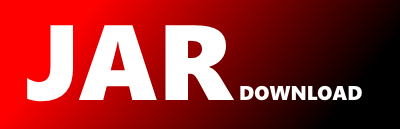
de.comhix.twitch.api.TwitchApi Maven / Gradle / Ivy
The newest version!
package de.comhix.twitch.api;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import com.google.gson.Gson;
import de.comhix.twitch.api.data.DetailedUser;
import de.comhix.twitch.api.data.User;
import de.comhix.twitch.api.data.UsersResponse;
import de.comhix.twitch.api.oauth.ClientInformation;
import de.comhix.twitch.api.oauth.OAuthResponse;
import de.comhix.twitch.api.oauth.SelfhostedTwitchAuthenticator;
import de.comhix.twitch.api.oauth.TwitchAuthenticator;
import io.reactivex.Observable;
import io.reactivex.schedulers.Schedulers;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.List;
import java.util.Map;
import static com.google.common.collect.Maps.newHashMap;
/**
* Main entry-point to use the Twitch-Api
*/
public class TwitchApi {
private static final Logger log = LoggerFactory.getLogger(TwitchApi.class);
private final ClientInformation clientInformation;
private final String oauthToken;
private static OkHttpClient client = new OkHttpClient.Builder().build();
private boolean closed = false;
private final Map chatProviders = newHashMap();
/**
* Creates a new instance for already connected user
*/
public TwitchApi(ClientInformation clientInformation, String oauthToken) {
this.clientInformation = Preconditions.checkNotNull(clientInformation);
this.oauthToken = Preconditions.checkNotNull(oauthToken);
}
/**
* Starts the oAuth process. Best for Website-based bots with own callback-url.
* {@link TwitchAuthenticator.SecondStep#getTargetUrl()} provides the URL the user should be redirected to for the oAuth process.
* You should keep the instance of {@link de.comhix.twitch.api.oauth.TwitchAuthenticator.SecondStep} somewhere cached to enter the auth-code.
*/
public static TwitchAuthenticator.SecondStep startOAuth(ClientInformation clientInformation, String callbackUrl) {
return new TwitchAuthenticator(clientInformation, callbackUrl).doAuth();
}
/**
* Starts the oAuth process. Best for Website-based bots with own callback-url.
* {@link TwitchAuthenticator.SecondStep#getTargetUrl()} provides the URL the user should be redirected to for the oAuth process.
* You should keep the instance of {@link de.comhix.twitch.api.oauth.TwitchAuthenticator.SecondStep} somewhere cached to enter the auth-code.
*/
public static TwitchAuthenticator.SecondStep startOAuth(ClientInformation clientInformation) {
return new TwitchAuthenticator(clientInformation).doAuth();
}
/**
* Does the whole oAuth process with starting a http server. This is for local executed Bots which run on the Desktop pc
*/
public static Observable doListeningOAuth(ClientInformation clientInformation, String landingHTML, int port) {
return new SelfhostedTwitchAuthenticator(clientInformation, landingHTML, port).doOAuth();
}
/**
* @see #doListeningOAuth(ClientInformation, String, int)
*/
public static Observable doListeningOAuth(ClientInformation clientInformation) {
return doListeningOAuth(clientInformation, SelfhostedTwitchAuthenticator.RESPONSE_HTML, SelfhostedTwitchAuthenticator.DEFAULT_PORT);
}
public void close() {
closed = true;
chatProviders.values().forEach(ChatProvider::close);
}
private void checkClosed() {
if (closed) {
throw new IllegalStateException("this TwitchApi instance is closed");
}
}
/**
* Provides the {@link ChatProvider} where you can send messages or register for incoming messages and events
*/
public Observable getChatProvider(String channel) {
checkClosed();
ChatProvider chatProvider = chatProviders.get(channel);
if (chatProvider == null) {
return getOwnInfo().map(ownInfo -> {
synchronized (chatProviders) {
return chatProviders.computeIfAbsent(channel, ignored -> new ChatProvider(oauthToken, ownInfo.getName(), channel));
}
});
}
return Observable.just(chatProvider);
}
/**
* @return The User information of the user identified by the oAuth credentials
*/
public Observable getOwnInfo() {
checkClosed();
return Observable.fromCallable(() -> {
Request.Builder request = new Request.Builder().url("https://api.twitch.tv/kraken/user")
.addHeader("Client-ID", clientInformation.getClientId())
.addHeader("Authorization", "OAuth " + oauthToken)
.addHeader("Accept", "application/vnd.twitchtv.v5+json");
InputStream body = client.newCall(request.build()).execute().body().byteStream();
return new Gson().fromJson(new InputStreamReader(body), DetailedUser.class);
}).observeOn(Schedulers.io());
}
/**
* @return The user information of the users given by loginNames
*/
public static Observable> getUsers(ClientInformation clientInformation, List loginNames) {
return Observable.fromCallable(() -> {
String url = "https://api.twitch.tv/kraken/users?login=" + Joiner.on(",").join(loginNames);
log.debug("calling {}", url);
Request.Builder request = new Request.Builder().url(url)
.addHeader("Client-ID", clientInformation.getClientId())
.addHeader("Accept", "application/vnd.twitchtv.v5+json");
String json = client.newCall(request.build()).execute().body().string();
log.debug("response json: {}", json);
return new Gson().fromJson(json, UsersResponse.class).users;
}).observeOn(Schedulers.io());
}
public Observable> getUsers(List loginNames) {
checkClosed();
return getUsers(clientInformation, loginNames);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy