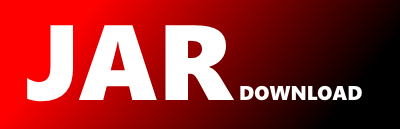
de.comhix.twitch.api.oauth.TwitchAuthenticator Maven / Gradle / Ivy
The newest version!
package de.comhix.twitch.api.oauth;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.gson.GsonBuilder;
import io.reactivex.Observable;
import io.reactivex.schedulers.Schedulers;
import okhttp3.Call;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.RequestBody;
import org.apache.commons.lang3.RandomStringUtils;
import java.io.InputStreamReader;
import java.util.Map;
import static com.google.common.collect.Maps.newHashMap;
/**
* @author Benjamin Beeker
*/
public class TwitchAuthenticator {
private static final ImmutableList SCOPES = ImmutableList.of("chat_login",
"user_blocks_edit",
"channel_editor",
"channel_feed_edit",
"user_read");
private final ClientInformation clientInformation;
public TwitchAuthenticator(ClientInformation clientInformation) {
this.clientInformation = Preconditions.checkNotNull(clientInformation, "clientInformation may not be null");
Preconditions.checkNotNull(clientInformation.getCallbackUrl(), "callbackUrl may not be null");
}
public TwitchAuthenticator(ClientInformation clientInformation, String callbackUrl) {
this(new ClientInformation(clientInformation.getClientId(), clientInformation.getClientSecret(), callbackUrl));
}
public SecondStep doAuth() {
String state = RandomStringUtils.randomAlphabetic(8);
String targetUrl = "https://api.twitch.tv/kraken/oauth2/authorize" +
"?response_type=code" +
"&client_id=" + clientInformation.getClientId() +
"&redirect_uri=" + clientInformation.getCallbackUrl() +
"&scope=" + Joiner.on("+").join(SCOPES) +
"&state=" + state;
return new SecondStep(state, targetUrl);
}
public class SecondStep {
private final String state;
private final String targetUrl;
SecondStep(String state, String targetUrl) {
this.state = state;
this.targetUrl = targetUrl;
}
public String getState() {
return state;
}
public String getTargetUrl() {
return targetUrl;
}
public Observable getOAuthToken(String authCode) {
OkHttpClient client = new OkHttpClient.Builder().build();
Map postData = newHashMap();
postData.put("client_id", clientInformation.getClientId());
postData.put("client_secret", clientInformation.getClientSecret());
postData.put("grant_type", "authorization_code");
postData.put("redirect_uri", clientInformation.getCallbackUrl());
postData.put("code", authCode);
postData.put("state", state);
String postString = Joiner.on("&").withKeyValueSeparator("=").join(postData);
okhttp3.Request request = new okhttp3.Request.Builder()
.post(RequestBody.create(MediaType.parse("application/x-www-form-urlencoded"), postString))
.url("https://api.twitch.tv/kraken/oauth2/token").build();
return Observable.just(request)
.map(client::newCall)
.subscribeOn(Schedulers.io())
.map(Call::execute)
.subscribeOn(Schedulers.io())
.map(response -> new GsonBuilder().create().fromJson(new InputStreamReader(response.body().byteStream()), OAuthResponse.class));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy