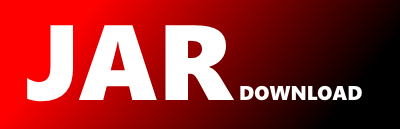
spoon.reflect.declaration.CtExecutable Maven / Gradle / Ivy
/*
* Spoon - http://spoon.gforge.inria.fr/
* Copyright (C) 2006 INRIA Futurs
*
* This software is governed by the CeCILL-C License under French law and
* abiding by the rules of distribution of free software. You can use, modify
* and/or redistribute the software under the terms of the CeCILL-C license as
* circulated by CEA, CNRS and INRIA at http://www.cecill.info.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the CeCILL-C License for more details.
*
* The fact that you are presently reading this means that you have had
* knowledge of the CeCILL-C license and that you accept its terms.
*/
package spoon.reflect.declaration;
import java.util.List;
import java.util.Set;
import spoon.reflect.code.CtBlock;
import spoon.reflect.reference.CtExecutableReference;
import spoon.reflect.reference.CtTypeReference;
/**
* This element represents an executable element such as a method, a
* constructor, or an anonymous block.
*/
public interface CtExecutable extends CtNamedElement, CtGenericElement,
CtTypedElement {
/**
* The separator for a string representation of an executable.
*/
public static final String EXECUTABLE_SEPARATOR = "#";
/**
* Gets the body expression.
*/
CtBlock getBody();
/**
* Gets the declaring type
*/
CtType> getDeclaringType();
/**
* Gets the parameters list.
*/
List> getParameters();
/*
* (non-Javadoc)
*
* @see spoon.reflect.declaration.CtNamedElement#getReference()
*/
CtExecutableReference getReference();
/**
* Returns the exceptions and other throwables listed in this method or
* constructor's throws clause.
*/
Set> getThrownTypes();
/**
* Sets the body expression.
*/
void setBody(CtBlock body);
/**
* Sets the parameters.
*/
void setParameters(List> parameters);
/**
* Add a parameter for this executable
*
* @param parameter
* @return true if this element changed as a result of the call
*/
boolean addParameter(CtParameter> parameter);
/**
* Remove a parameter for this executable
*
* @param parameter
* @return true if this element changed as a result of the call
*/
boolean removeParameter(CtParameter> parameter);
/**
* Sets the thrown types.
*/
void setThrownTypes(Set> thrownTypes);
/**
* add a thrown type.
*
* @param throwType
* @return true if this element changed as a result of the call
*/
boolean addThrownType(CtTypeReference extends Throwable> throwType);
/**
* remove a thrown type.
*
* @param throwType
* @return true if this element changed as a result of the call
*/
boolean removeThrownType(CtTypeReference extends Throwable> throwType);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy