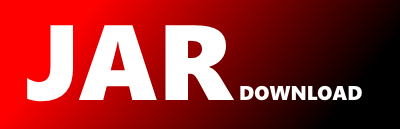
spoon.reflect.eval.SymbolicHeap Maven / Gradle / Ivy
/*
* Spoon - http://spoon.gforge.inria.fr/
* Copyright (C) 2006 INRIA Futurs
*
* This software is governed by the CeCILL-C License under French law and
* abiding by the rules of distribution of free software. You can use, modify
* and/or redistribute the software under the terms of the CeCILL-C license as
* circulated by CEA, CNRS and INRIA at http://www.cecill.info.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the CeCILL-C License for more details.
*
* The fact that you are presently reading this means that you have had
* knowledge of the CeCILL-C license and that you accept its terms.
*/
package spoon.reflect.eval;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import spoon.reflect.reference.CtTypeReference;
/**
* This class defines the heap for {@link spoon.reflect.eval.SymbolicEvaluator}.
*/
public class SymbolicHeap {
/**
* Creates an empty heap.
*/
public SymbolicHeap() {
}
/**
* Copies the given heap.
*/
@SuppressWarnings("unchecked")
public SymbolicHeap(SymbolicHeap heap) {
statelessAbstractInstances.putAll(heap.statelessAbstractInstances);
for (Entry> e : heap.statefullAbstractInstances
.entrySet()) {
statefullAbstractInstances.put(
e.getKey(),
new SymbolicInstance
© 2015 - 2025 Weber Informatics LLC | Privacy Policy