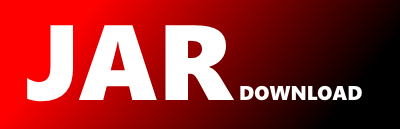
spoon.reflect.factory.FieldFactory Maven / Gradle / Ivy
/*
* Spoon - http://spoon.gforge.inria.fr/
* Copyright (C) 2006 INRIA Futurs
*
* This software is governed by the CeCILL-C License under French law and
* abiding by the rules of distribution of free software. You can use, modify
* and/or redistribute the software under the terms of the CeCILL-C license as
* circulated by CEA, CNRS and INRIA at http://www.cecill.info.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the CeCILL-C License for more details.
*
* The fact that you are presently reading this means that you have had
* knowledge of the CeCILL-C license and that you accept its terms.
*/
package spoon.reflect.factory;
import java.lang.reflect.Field;
import java.util.Set;
import spoon.reflect.code.CtExpression;
import spoon.reflect.declaration.CtField;
import spoon.reflect.declaration.CtSimpleType;
import spoon.reflect.declaration.CtType;
import spoon.reflect.declaration.ModifierKind;
import spoon.reflect.reference.CtFieldReference;
import spoon.reflect.reference.CtTypeReference;
/**
* The {@link CtField} sub-factory.
*/
public class FieldFactory extends SubFactory {
private static final long serialVersionUID = 1L;
/**
* Creates a new field sub-factory.
*
* @param factory
* the parent factory
*/
public FieldFactory(Factory factory) {
super(factory);
}
/**
* Creates a field.
*
* @param target
* the target type to which the field is added
* @param modifiers
* the modifiers
* @param type
* the field's type
* @param name
* the field's name
*/
public CtField create(CtSimpleType> target,
Set modifiers, CtTypeReference type, String name) {
CtField field = factory.Core().createField();
field.setModifiers(modifiers);
field.setType(type);
field.setSimpleName(name);
if (target != null)
target.getFields().add(field);
return field;
}
/**
* Creates a field.
*
* @param target
* the target type to which the field is added
* @param modifiers
* the modifiers
* @param type
* the field's type
* @param name
* the field's name
* @param defaultExpression
* the initializing expression
*/
public CtField create(CtSimpleType> target,
Set modifiers, CtTypeReference type, String name,
CtExpression defaultExpression) {
CtField field = create(target, modifiers, type, name);
field.setDefaultExpression(defaultExpression);
return field;
}
/**
* Creates a field by copying an existing field.
*
* @param
* the type of the field
* @param target
* the target type where the new field has to be inserted to
* @param source
* the source field to be copied
* @return the newly created field
*/
public CtField create(CtType> target, CtField source) {
CtField newField = factory.Core().clone(source);
if (target != null)
target.getFields().add(newField);
return newField;
}
/**
* Creates a field reference from an existing field.
*/
public CtFieldReference createReference(CtField field) {
return createReference(
factory.Type().createReference(field.getDeclaringType()),
field.getType(), field.getSimpleName());
}
/**
* Creates a field reference.
*/
public CtFieldReference createReference(
CtTypeReference> declaringType, CtTypeReference type,
String fieldName) {
CtFieldReference fieldRef = factory.Core().createFieldReference();
fieldRef.setSimpleName(fieldName);
fieldRef.setDeclaringType(declaringType);
fieldRef.setType(type);
return fieldRef;
}
/**
* Creates a field reference from a java.lang.reflect
field.
*/
@SuppressWarnings("unchecked")
public CtFieldReference createReference(Field field) {
CtFieldReference fieldRef = factory.Core().createFieldReference();
fieldRef.setSimpleName(field.getName());
fieldRef.setDeclaringType(factory.Type().createReference(
field.getDeclaringClass()));
CtTypeReference t = factory.Type().createReference(
(Class) field.getType());
fieldRef.setType(t);
return fieldRef;
}
/**
* Creates a field reference from its signature, as defined by the field
* reference's toString.
*/
public CtFieldReference createReference(String signature) {
CtFieldReference fieldRef = factory.Core().createFieldReference();
String type = signature.substring(0, signature.indexOf(" "));
String declaringType = signature.substring(signature.indexOf(" ") + 1,
signature.indexOf(CtField.FIELD_SEPARATOR));
String fieldName = signature.substring(signature
.indexOf(CtField.FIELD_SEPARATOR) + 1);
fieldRef.setSimpleName(fieldName);
fieldRef.setDeclaringType(factory.Type().createReference(declaringType));
CtTypeReference typeRef = factory.Type().createReference(type);
fieldRef.setType(typeRef);
return fieldRef;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy