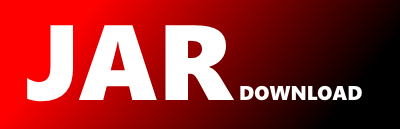
de.cuioss.tools.reflect.FieldWrapper Maven / Gradle / Ivy
/*
* Copyright 2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.cuioss.tools.reflect;
import java.lang.reflect.Field;
import java.lang.reflect.Member;
import java.util.Optional;
import de.cuioss.tools.logging.CuiLogger;
import de.cuioss.tools.string.MoreStrings;
import lombok.Getter;
import lombok.NonNull;
/**
* Wrapper around a {@link Field} that handles implicitly the accessible flag
* for access.
*
* @author Oliver Wolff
*
*/
@SuppressWarnings("java:S3011") // owolff: The warning is "Reflection should not be used to
// increase accessibility of classes, methods, or fields "
// What is actually the use-case of this type, therefore there
// is nothing we can do
public class FieldWrapper {
private static final CuiLogger log = new CuiLogger(FieldWrapper.class);
@Getter
@NonNull
private final Field field;
private final Class> declaringClass;
/**
* @param field must not be null
*/
public FieldWrapper(Field field) {
this.field = field;
declaringClass = ((Member) field).getDeclaringClass();
}
/**
* Reads from the field determined by {@link #getField()}. It implicitly sets
* and resets the {@link Field#isAccessible()} flag.
*
* @param object to be read from
* @return an {@link Optional} on the given field value if applicable. May
* return {@link Optional#empty()} for cases where:
*
* - Field value is {@code null}
* - Given Object is {@code null}
* - Given Object is improper type
* - an {@link IllegalAccessException} occurred while accessing
*
*/
public Optional