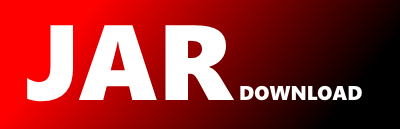
de.dagere.kopeme.kieker.writer.ChangeableFolderWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kopeme-core Show documentation
Show all versions of kopeme-core Show documentation
KoPeMe performance testing core
package de.dagere.kopeme.kieker.writer;
import java.io.File;
import java.io.IOException;
import java.nio.BufferUnderflowException;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map.Entry;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import kieker.common.configuration.Configuration;
import kieker.common.record.IMonitoringRecord;
import kieker.common.record.misc.KiekerMetadataRecord;
import kieker.common.record.misc.RegistryRecord;
import kieker.monitoring.writer.AbstractMonitoringWriter;
import kieker.monitoring.writer.filesystem.FileWriter;
/**
* This class enables Kieker writing in different folders for KoPeMe purposes. It does so by creating a new {@link SyncFsWriter} with every new folder that is set to the
* {@link ChangeableFolderWriter}. For storing all mapping data that is produced, every {@link RegistryRecord} that is measured is saved to a List and written to every new
* {@link SyncFsWriter} that is created with a new folder.
*
* @author reichelt
*
*/
public class ChangeableFolderWriter extends AbstractMonitoringWriter implements ChangeableFolder {
public static final String PREFIX = ChangeableFolderWriter.class.getName() + ".";
public static final String CONFIG_PATH = PREFIX + "customStoragePath";
public static final String CONFIG_MAXENTRIESINFILE = PREFIX + "maxEntriesInFile";
public static final String CONFIG_MAXLOGSIZE = PREFIX + "maxLogSize";
public static final String REAL_WRITER = PREFIX + "realwriter";
public static final String CONFIG_MAXLOGFILES = PREFIX + "maxLogFiles";
public static final String CONFIG_FLUSH = PREFIX + "flush";
public static final String CONFIG_BUFFER = PREFIX + "bufferSize";
private static ChangeableFolderWriter instance;
public static synchronized ChangeableFolderWriter getInstance() {
return instance;
}
private static final Logger LOG = LogManager.getLogger(ChangeableFolderWriter.class);
private final static List mappingRecords = new LinkedList<>();
private static boolean full = false;
private final Configuration configuration;
private AbstractMonitoringWriter currentWriter = null; // no writer is needed, until data is saved to where it belongs
public ChangeableFolderWriter(final Configuration configuration) {
super(configuration);
LOG.info("Init..");
this.configuration = configuration;
currentWriter = createWriter(configuration);
instance = this;
LOG.info("Writer: " + currentWriter.getClass());
}
private AbstractMonitoringWriter createWriter(final Configuration configuration) {
final String writerName = configuration.getStringProperty(REAL_WRITER);
try {
if (writerName.equals(FileWriter.class.getSimpleName())) {
final Configuration newConfig = toWriterConfiguration(configuration, FileWriter.class);
FileWriter fsWriter = new FileWriter(newConfig);
return fsWriter;
} else {
LOG.warn("Defined writer " + writerName + " not found - using default " + FileWriter.class.getSimpleName());
final Configuration newConfig = toWriterConfiguration(configuration, FileWriter.class);
final FileWriter syncFsWriter = new FileWriter(newConfig);
return syncFsWriter;
}
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
Configuration toWriterConfiguration(final Configuration configuration, final Class> writerClass) {
final Configuration returnable = new Configuration();
for (final Iterator> iterator = configuration.entrySet().iterator(); iterator.hasNext();) {
final Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy