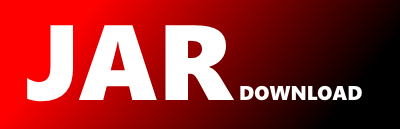
de.datasecs.hydra.shared.protocol.packets.Packet Maven / Gradle / Ivy
package de.datasecs.hydra.shared.protocol.packets;
import de.datasecs.hydra.shared.serialization.IgnoreSerialization;
import io.netty.buffer.ByteBuf;
import java.io.*;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Created with love by DataSecs on 29.09.2017.
*/
public abstract class Packet {
private ByteBuf byteBuf;
private Object objectToSerialize;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy