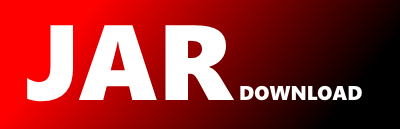
org.dummycreator.ConstructorCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dummy-creator Show documentation
Show all versions of dummy-creator Show documentation
A small tool for generating populated dummy objects
/*
* The contents of this file are subject to the terms of the Common Development
* and Distribution License (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the License at http://www.opensource.org/licenses/cddl1.php
* or http://www.opensource.org/licenses/cddl1.txt.
*
* When distributing Covered Code, include this CDDL Header Notice in each file
* and include the License file at http://www.opensource.org/licenses/cddl1.php.
* If applicable, add the following below the CDDL Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* The Original Software is dummyCreator. The Initial Developer of the Original
* Software is Alexander Muthmann .
*/
package org.dummycreator;
import java.lang.reflect.Constructor;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
*
* @author Alexander Muthmann
* @version 05/2010
*/
class ConstructorCache {
private final Map, List>> cache = new HashMap, List>>();
private final Map, Constructor>> preferedConstructors = new HashMap, Constructor>>();
public List> getCachedConstructors(final Class> clazz) {
List> cs = cache.get(clazz);
return cs == null ? null : Collections.unmodifiableList(cs);
}
public void addConstructor(final Class> clazz, final Constructor> cons) {
List> cs = cache.get(clazz);
if (cs == null) {
cs = new ArrayList>();
cache.put(clazz, cs);
}
cs.add(cons);
}
public void addConstructors(final Class> clazz, final List> cons) {
List> cs = cache.get(clazz);
if (cs == null) {
cs = new ArrayList>();
cache.put(clazz, cs);
}
cs.addAll(cons);
}
public Constructor> getPreferedConstructor(final Class> clazz) {
return preferedConstructors.get(clazz);
}
public void setPreferedConstructor(final Class> clazz, final Constructor> cons) {
preferedConstructors.put(clazz, cons);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy