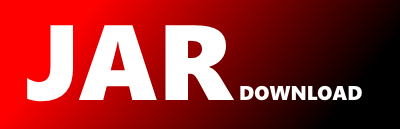
org.artofsolving.jodconverter.office.OfficeUtils Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2010 Daniel Manzke
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
//
// JODConverter - Java OpenDocument Converter
// Copyright 2009 Art of Solving Ltd
// Copyright 2004-2009 Mirko Nasato
//
// JODConverter is free software: you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public License
// as published by the Free Software Foundation, either version 3 of
// the License, or (at your option) any later version.
//
// JODConverter is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General
// Public License along with JODConverter. If not, see
// .
//
package org.artofsolving.jodconverter.office;
import java.io.File;
import java.util.Map;
import org.artofsolving.jodconverter.util.PlatformUtils;
import com.sun.star.beans.PropertyValue;
import com.sun.star.uno.UnoRuntime;
public class OfficeUtils {
public static final String SERVICE_DESKTOP = "com.sun.star.frame.Desktop";
private OfficeUtils() {
throw new AssertionError("utility class must not be instantiated");
}
public static T cast(Class type, Object object) {
return (T) UnoRuntime.queryInterface(type, object);
}
public static PropertyValue property(String name, Object value) {
PropertyValue propertyValue = new PropertyValue();
propertyValue.Name = name;
propertyValue.Value = value;
return propertyValue;
}
@SuppressWarnings("unchecked")
public static PropertyValue[] toUnoProperties(Map properties) {
PropertyValue[] propertyValues = new PropertyValue[properties.size()];
int i = 0;
for (Map.Entry entry : properties.entrySet()) {
Object value = entry.getValue();
if (value instanceof Map) {
Map subProperties = (Map) value;
value = toUnoProperties(subProperties);
}
propertyValues[i++] = property((String) entry.getKey(), value);
}
return propertyValues;
}
public static String toUrl(File file) {
String path = file.toURI().getRawPath();
String url = path.startsWith("//") ? "file:" + path : "file://" + path;
return url.endsWith("/") ? url.substring(0, url.length() - 1) : url;
}
public static File getDefaultOfficeHome() {
if (System.getProperty("office.home") != null) {
return new File(System.getProperty("office.home"));
}
if (PlatformUtils.isWindows()) {
return new File(System.getenv("ProgramFiles"), "OpenOffice.org 3");
} else if (PlatformUtils.isMac()) {
return new File("/Applications/OpenOffice.org.app/Contents");
} else {
// Linux or Solaris
return new File("/opt/openoffice.org3");
}
}
public static File getDefaultProfileDir() {
if (System.getProperty("office.profile") != null) {
return new File(System.getProperty("office.profile"));
}
if (PlatformUtils.isWindows()) {
return new File(System.getenv("APPDATA"), "OpenOffice.org/3");
} else if (PlatformUtils.isMac()) {
return new File(System.getProperty("user.home"), "Library/Application Support/OpenOffice.org/3");
} else {
// Linux or Solaris
return new File(System.getProperty("user.home"), ".openoffice.org/3");
}
}
public static File getOfficeExecutable(File officeHome) {
if (PlatformUtils.isMac()) {
return new File(officeHome, "MacOS/soffice.bin");
} else {
return new File(officeHome, "program/soffice.bin");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy