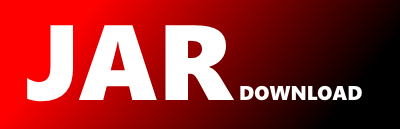
marytts.tools.install.ShortDescriptionPanel Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2009 DFKI GmbH.
* All Rights Reserved. Use is subject to license terms.
*
* This file is part of MARY TTS.
*
* MARY TTS is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
*/
/*
* ShortDescriptionPanel.java
*
* Created on 21. September 2009, 09:33
*/
package marytts.tools.install;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.event.ItemEvent;
import java.util.Observable;
import java.util.Observer;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
/**
*
* @author marc
*/
public class ShortDescriptionPanel extends javax.swing.JPanel implements Observer {
private ComponentDescription desc;
private VoiceUpdateListener voiceUpdate;
private Color selectedColor = new Color(102, 153, 255);
private Color unselectedColor;
private boolean isSelected = false;
/**
* Creates new form ShortDescriptionPanel
*
* @param desc
* desc
* @param voiceUpdate
* voiceUpdate
*/
public ShortDescriptionPanel(ComponentDescription desc, VoiceUpdateListener voiceUpdate) {
this.desc = desc;
desc.addObserver(this);
this.voiceUpdate = voiceUpdate;
initComponents();
this.unselectedColor = this.getBackground();
this.setMaximumSize(new Dimension(32767, (int) this.getPreferredSize().getHeight()));
verifyDisplay();
}
/**
* This method is called from within the constructor to initialize the form. WARNING: Do NOT modify this code. The content of
* this method is always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
cbSelected = new javax.swing.JCheckBox();
bDetails = new javax.swing.JButton();
lStatus = new javax.swing.JLabel();
setLayout(new java.awt.GridBagLayout());
setBorder(javax.swing.BorderFactory.createBevelBorder(javax.swing.border.BevelBorder.RAISED));
addFocusListener(new java.awt.event.FocusAdapter() {
public void focusGained(java.awt.event.FocusEvent evt) {
formFocusGained(evt);
}
public void focusLost(java.awt.event.FocusEvent evt) {
formFocusLost(evt);
}
});
addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
formMouseClicked(evt);
}
});
cbSelected.setText(desc.getName());
cbSelected.setBorder(javax.swing.BorderFactory.createEmptyBorder(0, 0, 0, 0));
cbSelected.setMargin(new java.awt.Insets(0, 0, 0, 0));
cbSelected.setPreferredSize(new java.awt.Dimension(200, 18));
cbSelected.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
cbSelectedItemStateChanged(evt);
}
});
cbSelected.addFocusListener(new java.awt.event.FocusAdapter() {
public void focusGained(java.awt.event.FocusEvent evt) {
formFocusGained(evt);
}
public void focusLost(java.awt.event.FocusEvent evt) {
formFocusLost(evt);
}
});
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridheight = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 0.5;
gridBagConstraints.insets = new java.awt.Insets(0, 5, 0, 0);
add(cbSelected, gridBagConstraints);
bDetails.setText("Details");
bDetails.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
bDetailsActionPerformed(evt);
}
});
bDetails.addFocusListener(new java.awt.event.FocusAdapter() {
public void focusGained(java.awt.event.FocusEvent evt) {
formFocusGained(evt);
}
public void focusLost(java.awt.event.FocusEvent evt) {
formFocusLost(evt);
}
});
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 1;
gridBagConstraints.weighty = 0.2;
gridBagConstraints.insets = new java.awt.Insets(0, 0, 5, 5);
add(bDetails, gridBagConstraints);
lStatus.setFont(new java.awt.Font("Lucida Grande", 0, 10));
lStatus.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
lStatus.setText((desc.isUpdateAvailable() ? "Update available" : desc.getStatus().toString()));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.weighty = 0.2;
gridBagConstraints.insets = new java.awt.Insets(5, 0, 0, 5);
add(lStatus, gridBagConstraints);
}// //GEN-END:initComponents
private void cbSelectedItemStateChanged(java.awt.event.ItemEvent evt) {// GEN-FIRST:event_cbSelectedItemStateChanged
if (evt.getStateChange() == ItemEvent.SELECTED) {
desc.setSelected(true);
} else if (evt.getStateChange() == ItemEvent.DESELECTED) {
desc.setSelected(false);
}
}// GEN-LAST:event_cbSelectedItemStateChanged
private void formMouseClicked(java.awt.event.MouseEvent evt) {// GEN-FIRST:event_formMouseClicked
this.requestFocusInWindow();
}// GEN-LAST:event_formMouseClicked
private void formFocusLost(java.awt.event.FocusEvent evt) {// GEN-FIRST:event_formFocusLost
setSelected(false);
}// GEN-LAST:event_formFocusLost
private void formFocusGained(java.awt.event.FocusEvent evt) {// GEN-FIRST:event_formFocusGained
setSelected(true);
}// GEN-LAST:event_formFocusGained
private void bDetailsActionPerformed(java.awt.event.ActionEvent evt) {// GEN-FIRST:event_bDetailsActionPerformed
showComponentDetails(desc);
}// GEN-LAST:event_bDetailsActionPerformed
private void setSelected(boolean newSelected) {
if (!isSelected && newSelected) {
isSelected = true;
this.setBackground(selectedColor);
cbSelected.setBackground(selectedColor);
if (voiceUpdate != null && desc instanceof LanguageComponentDescription) {
voiceUpdate.updateVoices((LanguageComponentDescription) desc, false);
}
} else if (isSelected && !newSelected) {
isSelected = false;
this.setBackground(unselectedColor);
cbSelected.setBackground(unselectedColor);
}
}
private void showComponentDetails(ComponentDescription aDesc) {
JPanel p;
if (aDesc instanceof LanguageComponentDescription) {
p = new LanguagePanel((LanguageComponentDescription) aDesc);
} else {
p = new VoicePanel((VoiceComponentDescription) aDesc);
}
JOptionPane.showMessageDialog(null, p, "Component details", JOptionPane.PLAIN_MESSAGE);
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton bDetails;
private javax.swing.JCheckBox cbSelected;
private javax.swing.JLabel lStatus;
// End of variables declaration//GEN-END:variables
public void update(Observable o, Object arg) {
verifyDisplay();
}
private void verifyDisplay() {
cbSelected.setSelected(desc.isSelected());
lStatus.setText((desc.isUpdateAvailable() ? "Update available" : desc.getStatus().toString()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy