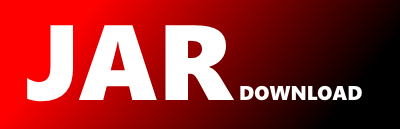
de.dnb.oai.harvester.manager.OaiHarvesterManagerImpl Maven / Gradle / Ivy
/**********************************************************************
* Class OaiHarvesterManagerImpl
*
* Copyright (c) 2004-2012, German National Library / Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.oai.harvester.manager;
import java.util.Calendar;
import java.util.List;
import java.util.Locale;
import java.util.concurrent.TimeUnit;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import de.dnb.oai.harvester.Harvester;
import de.dnb.oai.repository.Repository;
import de.dnb.oai.repository.RepositoryDao;
import de.dnb.stm.manager.AbstractScheduledManager;
import de.dnb.stm.manager.ManagerConstants;
import de.dnb.stm.manager.SchedulerException;
import de.dnb.stm.task.Task;
/** ********************************************************************
* OaiHarvesterManagerImpl manages all active and terminated
* harvesting activities.
*
* @author Kadir Karaca Kocer, German National Library
* @author Jürgen Kett, German National Library
* @version 20090220
* @see de.dnb.oai.harvester.Harvester
* @see de.dnb.oai.harvester.task.OaiTask
* @see java.util.concurrent.ScheduledThreadPoolExecutor
* @see org.springframework.beans.factory.InitializingBean
* @since ??.??.2004
******************************************************************** */
/* ******************************************************************
* CHANGELOG:
*
* 20120626 Maven Port
* 20090303 fixed scheduledTasks(): zombie Tasks filtered
* 20081201 repositoryDao with all related methods added
* Refactored 05.08.2008 by Kadir Karaca Kocer, German National Library
* Created on 10.09.2005 by Kett / Slotta, German National Library
******************************************************************** */
public class OaiHarvesterManagerImpl extends AbstractScheduledManager implements OaiHarvesterManager
{
private static final Log LOGGER = LogFactory.getLog(OaiHarvesterManagerImpl.class);
private RepositoryDao repositoryDao;
/**
* Constructor
*
* @param corePoolSize Initial Core Pool Size for ScheduledThreadPoolExecutor
* @throws IllegalAccessException
* @throws InstantiationException
* @see java.util.concurrent.ScheduledThreadPoolExecutor
* @author Kadir Karaca Kocer, German National Library
*/
public OaiHarvesterManagerImpl(int corePoolSize) throws InstantiationException, IllegalAccessException {
super(corePoolSize);
Locale.setDefault(Locale.GERMANY);
LOGGER.info("[OaiHarvesterManagerImpl] OaiHarvesterManagerImpl is successfully initialised. corePoolSize is " + corePoolSize);
}
// Repository Management ---------------------------
/**
* @return The Repository DAO
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public RepositoryDao getRepositoryDao() {
return this.repositoryDao;
}
/**
* @param repositoryDao The Repository DAO to set
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void setRepositoryDao(RepositoryDao repositoryDao) {
this.repositoryDao = repositoryDao;
}
/**
* @param rep Repository to add.
* @throws InstantiationException
* @throws IllegalAccessException
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void addRepository(Repository rep) throws InstantiationException, IllegalAccessException {
if (rep == null) {
throw new IllegalArgumentException("[OaiHarvesterManagerImpl] Repositoy can not be NULL!");
}
this.repositoryDao.saveRepository(rep);
}
/**
* @return Returns the list of all registered Repositories.
* @since 20.06.2008
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public List getRepositoryList() {
return this.repositoryDao.getRepositoriesSortedByName();
}
/**
* Returns the Repository with the given id.
*
* @param id Id of the Repository
* @return The Repository with the given id. NULL if no such Repository exists.
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public Repository getRepositoryById(Long id) {
return this.repositoryDao.getRepositoryById(id);
}
/**
* Schedules a OaiTask in the thread pool.
*
* @param task OaiTask to schedule
* @throws InstantiationException
* @throws IllegalAccessException
* @see java.util.concurrent.ScheduledThreadPoolExecutor
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void scheduleTask(Task task) throws InstantiationException, IllegalAccessException {
Harvester harvester = (Harvester)this.newProcessor();
harvester.init(this.context);
if (LOGGER.isDebugEnabled()) LOGGER.debug("scheduleTask: TaskDao hash code: " + this.taskDao.hashCode());
harvester.setTaskId(task.getTaskId());
long period = task.getRunInterval();
long initialDelay = task.getStartDate().getTime()- System.currentTimeMillis();
// correct timestamps if they are in the past. there were problems with minus values
if (initialDelay < 100) {
initialDelay = 100;
task.setStartDate(Calendar.getInstance(ManagerConstants.LOCALE).getTime());
}
if (LOGGER.isDebugEnabled()) LOGGER.debug("[OaiHarvesterManagerImpl] Scheduling OaiTask with id: " + task.getTaskId() +
", period: " + period + ", initialDelay: " + initialDelay);
if (period < 1) {
if (LOGGER.isDebugEnabled()) LOGGER.debug("[OaiHarvesterManagerImpl] Starting schedule: OaiTask will run only once.");
schedule(harvester, initialDelay, TimeUnit.MILLISECONDS);
} else {
if (LOGGER.isDebugEnabled()) LOGGER.debug("[OaiHarvesterManagerImpl] Scheduling at fixed rate:" +
" OaiTask will repeat every " + period + " seconds.");
scheduleAtFixedRate(harvester, initialDelay, (period * 1000), TimeUnit.MILLISECONDS);
}
this.taskDao.saveTask(task);
}
@Override
public void removeTask(Task arg0) throws InstantiationException, IllegalAccessException, SchedulerException {
throw new UnsupportedOperationException("Method not implemented yet!");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy