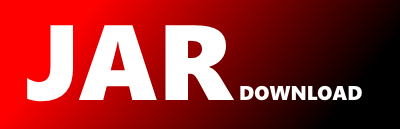
de.dnb.oai.harvester.task.InMemoryOaiTaskDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oaiharvester Show documentation
Show all versions of oaiharvester Show documentation
A Java implementation of OAI-PMH 2.0 specification.
See http://www.openarchives.org/pmh/
The newest version!
/**********************************************************************
* Class InMemoryOaiTaskDao
*
* Copyright (c) 2008-2012, German National Library / Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.oai.harvester.task;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import de.dnb.stm.manager.ManagerConstants;
import de.dnb.stm.task.Task;
import de.dnb.stm.task.TaskConstants;
/** ********************************************************************
* Class implementing an In-Memory implementation of TaskDao.
* Mostly for testing purposes. In production must the Database version
* be used.
*
* @author Kadir Karaca Kocer, German National Library
* @version 20090220
* @see de.dnb.oai.harvester.Harvester
* @see de.dnb.oai.harvester.task.OaiTask
* @see de.dnb.oai.harvester.task.Request
* @see de.dnb.stm.task.TaskDao
* @since 20080715
**********************************************************************/
public class InMemoryOaiTaskDao implements OaiTaskDao
{
/**
* Logger for this class
*/
private static final Log LOGGER = LogFactory.getLog(InMemoryOaiTaskDao.class);
private Map taskMap = new LinkedHashMap();
/**
* {@inheritDoc}
*/
@Override
public List getTaskList() {
if (this.taskMap == null) return new ArrayList();
if (this.taskMap.isEmpty()) return new ArrayList();
return new ArrayList(this.taskMap.values());
}
/**
* Sets the OaiTask-List.
*
* @param taskList OaiTask list.
* @author kocer, German National Library
*/
public void setTaskList(List taskList) {
for (OaiTask task : taskList) {
this.taskMap.put(new Long(task.getTaskId()), task);
}
}
/**
* {@inheritDoc}
*/
@Override
public void saveTask(Task task) {
if (task == null) {
throw new IllegalArgumentException("In MemoryTaskDao.saveTask(): Illegal Argument: OaiTask can not be NULL!");
}
if (task.getTaskId() == 0){
// 0 -> First initialisation! We have to do some initialisation work:
// try to generate a unique id for this OaiTask
long num = createUniqueNumber();
System.out.println("In MemoryTaskDao.saveTask(): new task. Setting its id to " + num);
((OaiTask)task).setTaskId(num);
num = createUniqueNumber();
((OaiTask)task).getRequest().setRequestId(num);
//create a new list for the already processed requests
((OaiTask)task).setProcessedRequests(new ArrayList());
}
this.taskMap.put(new Long(task.getTaskId()), ((OaiTask)task));
}
/**
* {@inheritDoc}
*/
@Override
public OaiTask getTask(Long taskId) {
if(LOGGER.isDebugEnabled()){LOGGER.debug("In MemoryTaskDao.getTask(): searching the task with id " + taskId);}
return this.taskMap.get(taskId);
}
/**
* {@inheritDoc}
*/
@Override
public void deleteTask(Task task) {
this.taskMap.remove(new Long(task.getTaskId()));
}
/**
* {@inheritDoc}
*/
@Override
public int deleteOldRequest(Task task, long RequestId) {
for (int i = 0; i < ((OaiTask)task).getProcessedRequests().size(); i++) {
if (RequestId == ((OaiTask)task).getProcessedRequests().get(i).getRequestId()) {
//found the searched request. remove it
((OaiTask)task).getProcessedRequests().remove(i);
return 0; // success
}
}
return 1; //no such ID
}
/**
* {@inheritDoc}
*/
@Override
public Request getNextRequest(Task task) {
//if this is not a repeating task there will be no next request!
long runInterval = task.getRunInterval();
if (runInterval == 0) {return null;}
//get the already terminated request
Request request = ((OaiTask)task).getRequest();
if (request == null) {
//actual request is NULL becouse the task was a one-time task. get the last request instead.
List l = ((OaiTask)task).getProcessedRequests();
request = l.get(l.size() -1);
}
//there will be a subsequent request. calculate the parameters
Request tempRequest = new Request();
//set some important values with the actual values
tempRequest.setRequestId(createUniqueNumber());
//calculate the new dates
if (request.getStatus() == 0) {
//Terminated successfully --> former until date is now from Date + 1 second
//TODO: use the OAI-PMH Response Date instead
tempRequest.setFromDate(new java.util.Date(request.getUntilDate().getTime() + 1000));
} else {
//there was an error --> fromDate must be the same
tempRequest.setFromDate(request.getFromDate());
}
if (request.getUntilDate() == null) {
//previous until date is not set. Should not occur! Set it.
request.setUntilDate(Calendar.getInstance(ManagerConstants.LOCALE).getTime());
}
//until date is + RunInterval
java.util.Date untilDate = new java.util.Date(request.getUntilDate().getTime() + (runInterval * 1000));
tempRequest.setUntilDate(untilDate);
return tempRequest;
}
/**
* {@inheritDoc}
*/
@Override
public void garbageCollector(long timeLimitTask, long timeLimitRequest) {
long now = System.currentTimeMillis();
synchronized(this.taskMap) {
java.util.Collection taskList = this.taskMap.values();
//first delete all the not repeating tasks, which are terminated and are older than given timestamp
for (OaiTask task : taskList) {
if ((timeLimitTask > 0) //0 means no tasks shall be erased
//remove only if its not repeating
&& (task.getRunInterval() == 0)
//remove only if its terminated
&& (task.getStatus() <= TaskConstants.TASK_STATUS_SUCCESSFULL)
//older than given timestamp
&& (task.getRequest().getFinishedAt().getTime() < (now - (timeLimitTask * 60000)) )) {
//all conditions are fullfilled. remove this task
taskList.remove(task);
if(LOGGER.isDebugEnabled()){LOGGER.debug("OaiTask removed!");}
} else { //its a repeating task
if (timeLimitRequest > 0) { //0 means no requests shall be erased
//remove all the requests which are older than given timestamp
for (int i = 0; i < task.getProcessedRequests().size(); i++) {
if (task.getProcessedRequests().get(i).getFinishedAt().getTime() < (now - (timeLimitTask * 60000))) {
//older than timestamp. remove it
task.getProcessedRequests().remove(i);
if(LOGGER.isDebugEnabled()){LOGGER.debug("Request erased!");}
} //if
} //for
} // if
} // else
} //for
}
}
private static long createUniqueNumber() {
// miliseconds + random number must be enough for our purpose
long ms = System.currentTimeMillis() - 1000000000000L;
java.util.Random rnd = new java.util.Random();
int r = (int) (rnd.nextFloat() * 10000);
return ((ms * 10000) + r);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy