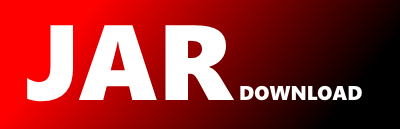
de.dnb.oai.harvester.task.InMemoryOaiTaskStopRequestDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oaiharvester Show documentation
Show all versions of oaiharvester Show documentation
A Java implementation of OAI-PMH 2.0 specification.
See http://www.openarchives.org/pmh/
The newest version!
/**********************************************************************
* Class InMemoryOaiTaskStopRequestDao
*
* Copyright (c) 2008-2012, German National Library / Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.oai.harvester.task;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import de.dnb.stm.task.TaskStopRequest;
import de.dnb.stm.task.TaskStopRequestDao;
/**
*
* @author Matthias Neubauer
*/
public class InMemoryOaiTaskStopRequestDao implements TaskStopRequestDao
{
private Map stopRequests = new LinkedHashMap();
@Override
public void deleteTaskStopRequest(TaskStopRequest taskStopRequest) {
this.stopRequests.remove(new Long(taskStopRequest.getTaskStopRequestId()));
}
@Override
public List findTaskStopRequestsByTaskId(
Long taskId) {
List tl = new ArrayList();
for (TaskStopRequest taskStopRequest : this.stopRequests.values()) {
if(new Long(taskStopRequest.getTaskId()).equals(taskId)){
tl.add(taskStopRequest);
}
}
return tl;
}
@Override
public TaskStopRequest getTaskStopRequest(Long taskStopRequestId) {
return this.stopRequests.get(taskStopRequestId);
}
@Override
public List getTaskStopRequestList() {
if (this.stopRequests == null) return new ArrayList();
if (this.stopRequests.isEmpty()) return new ArrayList();
return new ArrayList(this.stopRequests.values());
}
@Override
public void saveTaskStopRequest(TaskStopRequest taskStopRequest) {
if (taskStopRequest == null) {
throw new IllegalArgumentException("In InMemoryOaiTaskStopRequestDao.saveTaskStopRequest(): Illegal Argument: TaskStopRequest can not be NULL!");
}
if (taskStopRequest.getTaskStopRequestId() == 0){
// 0 -> First initialisation! We have to do some initialisation work:
// try to generate a unique id for this TaskStopRequest
((OaiTaskStopRequest)taskStopRequest).setTaskStopRequestId(createUniqueNumber());
}
this.stopRequests.put(new Long(taskStopRequest.getTaskStopRequestId()), taskStopRequest);
}
private static long createUniqueNumber() {
// miliseconds + random number must be enough for our purpose
long ms = System.currentTimeMillis();
java.util.Random rnd = new java.util.Random();
int r = (int) (rnd.nextFloat() * 10000);
return ((ms * 10000) + r);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy