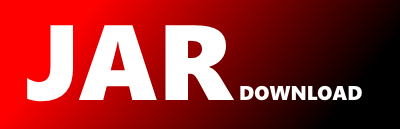
de.dnb.oai.harvester.task.Request Maven / Gradle / Ivy
/**********************************************************************
* Class Request
*
* Copyright (c) 2008-2012, German National Library / Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.oai.harvester.task;
import java.util.Date;
import de.dnb.stm.task.TaskConstants;
/** ********************************************************************
* Class representing a single OAI-PMH Request.
* Actually a request and result object together.
*
* @author Kadir Karaca Kocer, German National Library
* @version 20090220
* @see de.dnb.oai.harvester.Harvester
* @see de.dnb.oai.harvester.task.OaiTask
* {@link "http://www.openarchives.org/pmh/"}
* {@link "http://www.w3.org/TR/html40/appendix/notes.html"}
* @since 20080715
**********************************************************************/
/* ********************************************************************
* CHANGELOG:
*
* 05.08.2011, neubauer: Added ResponseDate of Response to adjust next from date if necessary
* Created on 15.07.2008 by Kadir Karaca Kocer, German National Library
**********************************************************************/
public class Request
{
// STATE (Instance variables) *****************************************
private long requestId; //unique ID of this request
private Date lastModified; //timestamp of last modification
private Date startedAt; //timestamp to start this request.
private Date finishedAt; //timestamp of the end of this request
private Date fromDate; //start harvesting records from this date
private Date untilDate; //harvest until this date
private Date responseDate = null; //responseDate of the servers response
private int status = TaskConstants.STATUS_NOT_DEFINED; //status of this request (yet not defined)
private String requestAsString = ""; //This request as text send to OAI-server
private int numberOfRuns = 0; //How many times is this request already run?
private int totalRecords = TaskConstants.STATUS_NOT_DEFINED;//How many Records total?
private int recordsHarvested = 0; //how many of them harvested?
//save the server response & exception info for later error analysis (if one occurs)
private String serverResponse = "";
private String exceptionMessage = "";
// GET & SET METHODS **************************************************
/**
* @return Returns the requestId.
* @author Kadir Karaca Kocer, German National Library
*/
public long getRequestId() {
return this.requestId;
}
/**
* @param id The requestId to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setRequestId(long id) {
this.requestId = id;
}
/**
* @return Returns the serverResponse.
* @author Kadir Karaca Kocer, German National Library
*/
public String getServerResponse() {
return this.serverResponse;
}
/**
* @param response The serverResponse to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setServerResponse(String response) {
this.serverResponse = response;
}
/**
* @return Returns the timestamp lastModified.
* @author Kadir Karaca Kocer, German National Library
*/
public Date getLastModified() {
return this.lastModified;
}
/**
* @param newLastModified The lastModified to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setLastModified(Date newLastModified) {
this.lastModified = newLastModified;
}
/**
* @return Returns the Date of start.
* @author Kadir Karaca Kocer, German National Library
*/
public Date getStartedAt() {
return this.startedAt;
}
/**
* @param newStartedAt The startDate to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setStartedAt(Date newStartedAt) {
this.startedAt = newStartedAt;
}
/**
* @return Returns the finishedAt.
* @author Kadir Karaca Kocer, German National Library
*/
public Date getFinishedAt() {
return this.finishedAt;
}
/**
* @param newFinishedAt The finishedAt to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setFinishedAt(Date newFinishedAt) {
this.finishedAt = newFinishedAt;
}
/**
* @return Returns the status.
* @author Kadir Karaca Kocer, German National Library
*/
public int getStatus() {
return this.status;
}
/**
* @param newStatus The status to set.
* @author Kadir Karaca Kocer, German National Library
*/
public synchronized void setStatus(int newStatus) {
this.status = newStatus;
}
/**
* @return Returns the requestAsString.
* @author Kadir Karaca Kocer, German National Library
*/
public String getRequestAsString() {
return this.requestAsString;
}
/**
* @param request The requestAsString to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setRequestAsString(String request) {
this.requestAsString = request;
}
/**
* @return Returns the numberOfRuns.
* @author Kadir Karaca Kocer, German National Library
*/
public int getNumberOfRuns() {
return this.numberOfRuns;
}
/**
* @param num The numberOfRuns to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setNumberOfRuns(int num) {
this.numberOfRuns = num;
}
/**
* @return Returns the totalRecords.
* @author Kadir Karaca Kocer, German National Library
*/
public int getTotalRecords() {
return this.totalRecords;
}
/**
* @param total The totalRecords to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setTotalRecords(int total) {
this.totalRecords = total;
}
/**
* @return Returns the recordHarvested.
* @author Kadir Karaca Kocer, German National Library
*/
public int getRecordsHarvested() {
return this.recordsHarvested;
}
/**
* @param harvested The recordHarvested to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setRecordsHarvested(int harvested) {
this.recordsHarvested = harvested;
}
/**
* @return Returns the fromDate.
* @author Kadir Karaca Kocer, German National Library
*/
public Date getFromDate() {
return this.fromDate;
}
/**
* @param newFromDate The fromDate to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setFromDate(Date newFromDate) {
this.fromDate = newFromDate;
}
/**
* @return Returns the untilDate.
* @author Kadir Karaca Kocer, German National Library
*/
public Date getUntilDate() {
return this.untilDate;
}
/**
* @param newUntilDate The untilDate to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setUntilDate(Date newUntilDate) {
this.untilDate = newUntilDate;
}
/**
* @param message The exception message to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setExceptionMessage(String message) {
this.exceptionMessage = message;
}
/**
* @return Returns the exception message.
* @author Kadir Karaca Kocer, German National Library
*/
public String getExceptionMessage() {
return this.exceptionMessage;
}
/**
* @return Response date.
*/
public Date getResponseDate() {
return this.responseDate;
}
/**
* @param responseDate Date to set.
*/
public void setResponseDate(Date responseDate) {
this.responseDate = responseDate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy