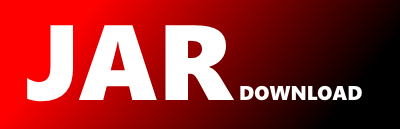
de.dnb.oai.repository.InMemoryRepositoryDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oaiharvester Show documentation
Show all versions of oaiharvester Show documentation
A Java implementation of OAI-PMH 2.0 specification.
See http://www.openarchives.org/pmh/
The newest version!
/**********************************************************************
* Class InMemoryRepositoryDao
*
* Copyright (c) 2005-2012, German National Library / Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.oai.repository;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/** ********************************************************************
* Class implementing an In-Memory implementation of RepositoryDao.
* Mostly for testing purposes. In production must the Database version
* be used.
*
* @author Kadir Karaca Kocer, German National Library
* @version 20090220
* @see de.dnb.oai.repository.RepositoryDao
* @see de.dnb.oai.repository.Repository
* @see de.dnb.oai.harvester.Harvester
* @see de.dnb.oai.harvester.task.OaiTask
* @since 20081120
**********************************************************************/
public class InMemoryRepositoryDao implements RepositoryDao
{
private static final Log LOGGER = LogFactory.getLog(InMemoryRepositoryDao.class);
private Map repositoryMap = new LinkedHashMap();
InMemoryRepositoryDao() {
LOGGER.debug("InMemoryRepositoryDao Initialised!");
}
/**
* Returns the list of registered OAI-Repositories.
*
* @return The list of registered OAI-Repositories.
*/
@Override
public List getRepositories() {
if (this.repositoryMap == null) return new ArrayList();
if (this.repositoryMap.isEmpty()) return new ArrayList();
return new ArrayList(this.repositoryMap.values());
}
/**
* Returns the OAI-Repository with the given unique id.
*
* @param id Id of the repository.
* @return The repository with the given id.
*/
@Override
public Repository getRepositoryById(Long id) {
return this.repositoryMap.get(id);
}
/**
* Saves the given the OAI-Repository.
*
* @param rep Repository to save.
*/
@Override
public void saveRepository(Repository rep) {
if (rep == null) {
throw new IllegalArgumentException("InMemoryRepositoryDao.saveRepository(): " +
"Illegal Argument: OAI-Repository can not be NULL!");
}
if (rep.getRepositoryId() == null){
//0 -> first initialisation
rep.setRepositoryId(new Long(System.currentTimeMillis())); //pseudo-unique-id!!
}
this.repositoryMap.put(rep.getRepositoryId(), rep);
LOGGER.debug("Repository saved.");
}
/**
* Deletes the given the OAI-Repository.
*
* @param rep The repository to delete.
*/
@Override
public void deleteRepository(Repository rep) {
//TODO: fix this!
/* Deactivated after refactoring!! We can not check if its referenced any more.
*
//first check if this OAI Repository is referenced from a OaiTask
boolean referenced = false;
long id = rep.getRepositoryId();
java.util.Collection taskList = this.taskMap.values();
for (OaiTask task : taskList) {
if (task.getRepository().getRepositoryId() == id) referenced = true;
}
//if not referenced remove it from the map
if (!referenced) this.repositoryMap.remove(new Long(rep.getRepositoryId()));
*/
LOGGER.debug("deleteRepository() is inactive.");
}
@Override
public List getRepositoriesSortedByName() {
//no sorting here, but not that bad
return getRepositories();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy