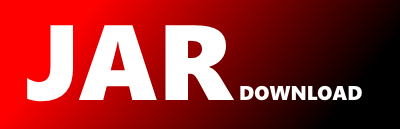
de.dnb.oai.repository.Repository Maven / Gradle / Ivy
/**********************************************************************
* Class Repository
*
* Copyright (c) 2005-2012, German National Library / Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.oai.repository;
import java.util.Date;
/** ********************************************************************
* Bean representing a OAI-PMH Repository
*
* @author Kadir Karaca Kocer, German National Library
* @version 20090220
* @see de.dnb.oai.harvester.task.OaiTask
* @see de.dnb.oai.harvester.task.Request
* @see de.dnb.oai.harvester.Harvester
* @since 20081119
**********************************************************************/
/* *******************************************************************
* CHANGELOG:
*
* Created on 19.11.2008 by Kadir Karaca Kocer, German National Library
**********************************************************************/
public class Repository
{
private Long repositoryId; //Unique IDS of this repository, maschine generated
private String repositoryName = "New OAI-Repository"; //Name of the OAI-Repository (free text for humans)
private String baseUrl = ""; //URI of the OAI-Repository
private int connectionDelay = 120; //How many seconds between two harvests? (Default 2 Minutes)
private int socketTimeout = 300000; //How many milliseconds to socket timeout? (Default 5 Minutes)
private int maxNumberOfRetries = 5; //How many times shall this request maximum run?
private boolean hasFineGranularity = false;//the datetime granularity; true for fine; false otherwise
private boolean strictHTTP = false; //
private int numberOfErrors = 0;
private Date lastKnownGood;
@SuppressWarnings("unused")
private Date lastModified; //for Hibernate
/**
* @return the repositoryId
* @author Kadir Karaca Kocer, German National Library
*/
public Long getRepositoryId() {
return this.repositoryId;
}
/**
* @param repositoryId the repositoryId to set
* @author Kadir Karaca Kocer, German National Library
*/
public void setRepositoryId(Long repositoryId) {
this.repositoryId = repositoryId;
}
/**
* @return Returns the repositoryName.
* @author Kadir Karaca Kocer, German National Library
*/
public String getRepositoryName() {
return this.repositoryName;
}
/**
* @param newRepositoryName The description to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setRepositoryName(String newRepositoryName) {
this.repositoryName = newRepositoryName;
}
/**
* @return Returns the baseURL.
* @author Kadir Karaca Kocer, German National Library
*/
public String getBaseUrl() {
return this.baseUrl;
}
/**
* @param newBaseURL The baseUrl to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setBaseUrl(String newBaseURL) {
this.baseUrl = newBaseURL.trim();
}
/**
* @return Maximun number of retries
* @author Kadir Karaca Kocer, German National Library
*/
public int getMaxNumberOfRetries() {
return this.maxNumberOfRetries;
}
/**
* @param number Maximun number of retries
* @author Kadir Karaca Kocer, German National Library
*/
public void setMaxNumberOfRetries(int number){
this.maxNumberOfRetries = number;
}
/**
* @return Returns the hasFineGranularity.
* @author Kadir Karaca Kocer, German National Library
*/
public boolean isHasFineGranularity() {
return this.hasFineGranularity;
}
/**
* @param isFine The hasFineGranularity to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setHasFineGranularity(boolean isFine) {
this.hasFineGranularity = isFine;
}
/**
* @return Returns the numberOfErrors.
*@author Kadir Karaca Kocer, German National Library
*/
public int getNumberOfErrors() {
return this.numberOfErrors;
}
/**
* Increase the number of errors if an error occurs.
*
* @author Kadir Karaca Kocer, German National Library
*/
public void increaseNumberOfErrors() {
this.numberOfErrors = this.numberOfErrors + 1;
}
/**
* Set the number of errors.
*
* @param number Number of errors.
* @author Kadir Karaca Kocer, German National Library
*/
public void setNumberOfErrors(int number) {
this.numberOfErrors = number;
}
/**
* @return Returns the lastKnownGood.
* @author Kadir Karaca Kocer, German National Library
*/
public Date getLastKnownGood() {
return this.lastKnownGood;
}
/**
* @param lastTime The lastKnownGood to set.
* @author Kadir Karaca Kocer, German National Library
*/
public void setLastKnownGood(Date lastTime) {
this.lastKnownGood = lastTime;
}
/**
* @return the connectionDelay
* @author Kadir Karaca Kocer, German National Library
*/
public int getConnectionDelay() {
return this.connectionDelay;
}
/**
* @param delay the connection_delay to set
* @author Kadir Karaca Kocer, German National Library
*/
public void setConnectionDelay(int delay) {
this.connectionDelay = delay;
}
/**
* @return the socketTimeout
* @author Kadir Karaca Kocer, German National Library
*/
public int getSocketTimeout() {
return this.socketTimeout;
}
/**
* @param st the socket_timeout to set
* @author Kadir Karaca Kocer, German National Library
*/
public void setSocketTimeout(int st) {
this.socketTimeout = st;
}
/**
* See Apache Commons HTTP Client documentation.
*
* @param strictHTTP the strictHTTP to set
* @author Kadir Karaca Kocer, German National Library
*/
public void setStrictHTTP(boolean strictHTTP) {
this.strictHTTP = strictHTTP;
}
/**
* @return the strictHTTP
* @author Kadir Karaca Kocer, German National Library
*/
public boolean isStrictHTTP() {
return this.strictHTTP;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy