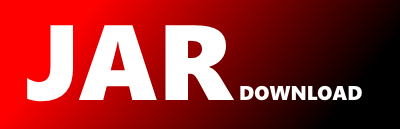
de.dnb.stm.manager.AbstractScheduledManager Maven / Gradle / Ivy
/**********************************************************************
* Class AbstractScheduledManager
*
* Copyright (c) 2005-2012, German National Library/Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
package de.dnb.stm.manager;
import java.util.Calendar;
import java.util.List;
import java.util.Locale;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import de.dnb.stm.handler.export.ExportHandlerDao;
import de.dnb.stm.processor.AbstractProcessor;
import de.dnb.stm.processor.Processor;
import de.dnb.stm.task.Task;
import de.dnb.stm.task.TaskConstants;
import de.dnb.stm.task.TaskDao;
import de.dnb.stm.task.TaskStopRequestDao;
/** ********************************************************************
* AbstractScheduledManager manages all active and terminated tasks.
* Specific implementation must implement its own algorithm.
*
* @author Matthias Neubauer, German National Library
* @author Kadir Karaca Kocer, German National Library
* @author Jürgen Kett, German National Library
* @version 20120627
*
* @see java.util.concurrent.ScheduledThreadPoolExecutor
* @see org.springframework.beans.factory.InitializingBean
* @since 2005
******************************************************************** */
/* ******************************************************************
* CHANGELOG:
*
* 20120618 dependencies to Quarz eliminated / Maven port
* Refactored 02.11.2010 by Matthias Neubauer, German National Library
* 20090303 fixed scheduledTasks(): zombie Tasks filtered
* 20081201 repositoryDao with all related methods added
* Refactored 05.08.2008 by Kadir Karaca Kocer, German National Library
* Created on 10.09.2005 by Kett / Slotta, German National Library
******************************************************************** */
public abstract class AbstractScheduledManager extends ScheduledThreadPoolExecutor implements ScheduledManager, ApplicationContextAware
{
private static final Log LOGGER = LogFactory.getLog(AbstractScheduledManager.class);
protected ApplicationContext context;
protected TaskDao taskDao;
protected TaskStopRequestDao taskStopRequestDao;
protected ExportHandlerDao exportHandlerDao;
protected Class extends AbstractProcessor> processorClass = AbstractProcessor.class;
/**
* Constructor
*
* @param corePoolSize Initial Core Pool Size for ScheduledThreadPoolExecutor
* @throws IllegalAccessException
* @throws InstantiationException
* @see java.util.concurrent.ScheduledThreadPoolExecutor
* @author Kadir Karaca Kocer, German National Library
*/
public AbstractScheduledManager(int corePoolSize) throws InstantiationException, IllegalAccessException {
super(corePoolSize);
Locale.setDefault(Locale.GERMANY);
LOGGER.info("[ScheduledManagerImpl] ScheduledManagerImpl is successfully initialised. corePoolSize is " + corePoolSize);
}
/**
* Method to initialise all objects after first start.
*
* @throws Exception
* @see org.springframework.beans.factory.InitializingBean
* @author Kadir Karaca Kocer, German National Library
*/
public void init() throws Exception {
this.taskDao = (TaskDao)this.context.getBean("taskDao");
this.taskStopRequestDao = (TaskStopRequestDao)this.context.getBean("taskStopRequestDao");
this.exportHandlerDao = (ExportHandlerDao) this.context.getBean("exportHandlerDao");
this.scheduleTasks();
}
// OaiTask Management ---------------------------
/**
* Schedules all Tasks which are not finished.
*
* @throws InstantiationException
* @throws IllegalAccessException
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void scheduleTasks() throws InstantiationException, IllegalAccessException {
LOGGER.info("[ScheduledManagerImpl] scheduleTasks() - start");
//first remove all finished requests and non-repeating tasks which are older than 2 Months
this.taskDao.garbageCollector(82000, 82000);
//then schedule the waiting and paused tasks
for (Task task : this.taskDao.getTaskList()) {
LOGGER.debug("[ScheduledManagerImpl] Found OaiTask with ID: " + task.getTaskId() + " Its Status is: " + task.getStatus());
//check if its a "Zombie-OaiTask" -> server shut down during harvesting
if (task.getStatus() == TaskConstants.TASK_STATUS_RUNNING) {
//it can not be running. its not initialized. this is an error. we log and cancel it
LOGGER.error("ERROR: OaiTask Status can not be RUNNING at this point. " +
"It seems that your Servlet Container gone down during harvesting process!");
//TODO: Define a new status (Zombie?). Find a functioning solution on Tomcat-Clusters
//task.setStatus(Constants.TASK_STATUS_CANCELLED);
//TODO: check this!!
task.setStatus(TaskConstants.TASK_STATUS_PAUSED);
this.taskDao.saveTask(task);
}
if ((task.getStatus() == TaskConstants.TASK_STATUS_WAITING) || (task.getStatus() == TaskConstants.TASK_STATUS_PAUSED)) {
//task is waiting or paused. check if its repeating
long period = task.getRunInterval();
if (period > 0) {
//its a repeating task. calculate the next running time
LOGGER.debug("[ScheduledManagerImpl] Run interval is: " + period + " seconds. Calculating new StartDate");
long now = System.currentTimeMillis() + 1000;
// task will be repeated
long step = period * 1000;
long startDate = task.getStartDate().getTime();
for (; startDate < now; startDate = startDate + step) {
// nothing to do. we just want to count the startDate higher;
}
Calendar c = Calendar.getInstance(ManagerConstants.LOCALE);
c.setTimeInMillis(startDate);
task.setStartDate(c.getTime());
LOGGER.debug("[ScheduledManagerImpl] New StartDate: " + task.getStartDate());
} // if
scheduleTask(task);
} // if
} // for
LOGGER.info("[ScheduledManagerImpl] scheduleTasks() - End. All tasks scheduled: " +
this.getTaskCount() + " tasks in Scheduled-Tasks-List");
}
/**
* Schedules a OaiTask in the thread pool.
*
* @param task OaiTask to schedule
* @throws InstantiationException
* @throws IllegalAccessException
* @see java.util.concurrent.ScheduledThreadPoolExecutor
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public abstract void scheduleTask(Task task) throws InstantiationException, IllegalAccessException;
/**
* Adds a OaiTask in the thread pool.
*
* @param task OaiTask to add.
* @throws InstantiationException
* @throws IllegalAccessException
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void addTask(Task task) throws InstantiationException, IllegalAccessException {
if (task == null) {
throw new IllegalArgumentException("[ScheduledManagerImpl] Task can not be null");
}
this.taskDao.saveTask(task);
task.setStatus(TaskConstants.TASK_STATUS_WAITING);
scheduleTask(task);
}
/**
* @return Returns the taskDao.
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public TaskDao getTaskDao() {
return this.taskDao;
}
/**
* @param newTaskDao The taskDao to set.
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void setTaskDao(TaskDao newTaskDao) {
this.taskDao = newTaskDao;
}
@Override
public TaskStopRequestDao getTaskStopRequestDao() {
return this.taskStopRequestDao;
}
@Override
public void setTaskStopRequestDao(TaskStopRequestDao taskStopRequestDao) {
this.taskStopRequestDao = taskStopRequestDao;
}
/**
* @return Returns the taskList.
* @author Kadir Karaca Kocer, German National Library
* @since 20.06.2008
*/
@Override
public List getTaskList() {
return this.taskDao.getTaskList();
}
/**
* Returns the task with the given id.
*
* @param id Id of the task
* @return The OaiTask with the given id. NULL if no such task exists.
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public Task getTaskById(long id) {
return this.taskDao.getTask(new Long(id));
}
/**
* @return Returns the exportHandlerDao.
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public ExportHandlerDao getExportHandlerDao() {
return this.exportHandlerDao;
}
/**
* @param ehd The exportHandlerDao to set.
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public void setExportHandlerDao(ExportHandlerDao ehd) {
this.exportHandlerDao = ehd;
}
/**
*
* @see de.dnb.stm.processor
* @author Kadir Karaca Kocer, German National Library
*/
@Override
public Processor newProcessor() throws InstantiationException, IllegalAccessException {
return this.processorClass.newInstance();
}
/**
* @return Returns the processorClass.
* @author Kadir Karaca Kocer, German National Library
* @since 20.06.2008
*/
public Class< ? extends AbstractProcessor> getProcessorClass() {
return this.processorClass;
}
/**
* @param processorclass The processorClass to set.
* @author Kadir Karaca Kocer, German National Library
* @since 20.06.2008
*/
public void setProcessorClass(Class< ? extends AbstractProcessor> processorclass) {
this.processorClass = processorclass;
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.context = applicationContext;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy