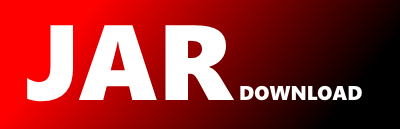
de.flapdoodle.embed.mongo.packageresolver.ImmutableFeatureSetRule Maven / Gradle / Ivy
package de.flapdoodle.embed.mongo.packageresolver;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.EnumSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
/**
* Immutable implementation of {@link FeatureSetRule}.
*
* Use the builder to create immutable instances:
* {@code ImmutableFeatureSetRule.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableFeatureSetRule implements FeatureSetRule {
private final VersionRange versionRange;
private final Set features;
private ImmutableFeatureSetRule(
VersionRange versionRange,
Set features) {
this.versionRange = versionRange;
this.features = features;
}
/**
* @return The value of the {@code versionRange} attribute
*/
@Override
public VersionRange versionRange() {
return versionRange;
}
/**
* @return The value of the {@code features} attribute
*/
@Override
public Set features() {
return features;
}
/**
* Copy the current immutable object by setting a value for the {@link FeatureSetRule#versionRange() versionRange} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for versionRange
* @return A modified copy of the {@code this} object
*/
public final ImmutableFeatureSetRule withVersionRange(VersionRange value) {
if (this.versionRange == value) return this;
VersionRange newValue = Objects.requireNonNull(value, "versionRange");
return new ImmutableFeatureSetRule(newValue, this.features);
}
/**
* Copy the current immutable object with elements that replace the content of {@link FeatureSetRule#features() features}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableFeatureSetRule withFeatures(Feature... elements) {
Set newValue = createUnmodifiableEnumSet(Arrays.asList(elements));
return new ImmutableFeatureSetRule(this.versionRange, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link FeatureSetRule#features() features}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of features elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableFeatureSetRule withFeatures(Iterable elements) {
if (this.features == elements) return this;
Set newValue = createUnmodifiableEnumSet(elements);
return new ImmutableFeatureSetRule(this.versionRange, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableFeatureSetRule} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableFeatureSetRule
&& equalTo(0, (ImmutableFeatureSetRule) another);
}
private boolean equalTo(int synthetic, ImmutableFeatureSetRule another) {
return versionRange.equals(another.versionRange)
&& features.equals(another.features);
}
/**
* Computes a hash code from attributes: {@code versionRange}, {@code features}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + versionRange.hashCode();
h += (h << 5) + features.hashCode();
return h;
}
/**
* Prints the immutable value {@code FeatureSetRule} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "FeatureSetRule{"
+ "versionRange=" + versionRange
+ ", features=" + features
+ "}";
}
/**
* Creates an immutable copy of a {@link FeatureSetRule} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable FeatureSetRule instance
*/
public static ImmutableFeatureSetRule copyOf(FeatureSetRule instance) {
if (instance instanceof ImmutableFeatureSetRule) {
return (ImmutableFeatureSetRule) instance;
}
return ((ImmutableFeatureSetRule.Builder) ImmutableFeatureSetRule.builder())
.versionRange(instance.versionRange())
.addAllFeatures(instance.features())
.build();
}
/**
* Creates a builder for {@link ImmutableFeatureSetRule ImmutableFeatureSetRule}.
*
* ImmutableFeatureSetRule.builder()
* .versionRange(de.flapdoodle.embed.mongo.packageresolver.VersionRange) // required {@link FeatureSetRule#versionRange() versionRange}
* .addFeatures|addAllFeatures(de.flapdoodle.embed.mongo.packageresolver.Feature) // {@link FeatureSetRule#features() features} elements
* .build();
*
* @return A new ImmutableFeatureSetRule builder
*/
public static VersionRangeBuildStage builder() {
return new ImmutableFeatureSetRule.Builder();
}
/**
* Builds instances of type {@link ImmutableFeatureSetRule ImmutableFeatureSetRule}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder implements VersionRangeBuildStage, BuildFinal {
private static final long INIT_BIT_VERSION_RANGE = 0x1L;
private long initBits = 0x1L;
private VersionRange versionRange;
private final EnumSet features = EnumSet.noneOf(Feature.class);
private Builder() {
}
/**
* Initializes the value for the {@link FeatureSetRule#versionRange() versionRange} attribute.
* @param versionRange The value for versionRange
* @return {@code this} builder for use in a chained invocation
*/
public final Builder versionRange(VersionRange versionRange) {
checkNotIsSet(versionRangeIsSet(), "versionRange");
this.versionRange = Objects.requireNonNull(versionRange, "versionRange");
initBits &= ~INIT_BIT_VERSION_RANGE;
return this;
}
/**
* Adds one element to {@link FeatureSetRule#features() features} set.
* @param element A features element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addFeatures(Feature element) {
this.features.add(Objects.requireNonNull(element, "features element"));
return this;
}
/**
* Adds elements to {@link FeatureSetRule#features() features} set.
* @param elements An array of features elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addFeatures(Feature... elements) {
for (Feature element : elements) {
this.features.add(Objects.requireNonNull(element, "features element"));
}
return this;
}
/**
* Adds elements to {@link FeatureSetRule#features() features} set.
* @param elements An iterable of features elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllFeatures(Iterable elements) {
for (Feature element : elements) {
this.features.add(Objects.requireNonNull(element, "features element"));
}
return this;
}
/**
* Builds a new {@link ImmutableFeatureSetRule ImmutableFeatureSetRule}.
* @return An immutable instance of FeatureSetRule
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableFeatureSetRule build() {
checkRequiredAttributes();
return new ImmutableFeatureSetRule(versionRange, createUnmodifiableEnumSet(features));
}
private boolean versionRangeIsSet() {
return (initBits & INIT_BIT_VERSION_RANGE) == 0;
}
private static void checkNotIsSet(boolean isSet, String name) {
if (isSet) throw new IllegalStateException("Builder of FeatureSetRule is strict, attribute is already set: ".concat(name));
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!versionRangeIsSet()) attributes.add("versionRange");
return "Cannot build FeatureSetRule, some of required attributes are not set " + attributes;
}
}
public interface VersionRangeBuildStage {
/**
* Initializes the value for the {@link FeatureSetRule#versionRange() versionRange} attribute.
* @param versionRange The value for versionRange
* @return {@code this} builder for use in a chained invocation
*/
BuildFinal versionRange(VersionRange versionRange);
}
public interface BuildFinal {
/**
* Adds one element to {@link FeatureSetRule#features() features} set.
* @param element A features element
* @return {@code this} builder for use in a chained invocation
*/
BuildFinal addFeatures(Feature element);
/**
* Adds elements to {@link FeatureSetRule#features() features} set.
* @param elements An array of features elements
* @return {@code this} builder for use in a chained invocation
*/
BuildFinal addFeatures(Feature... elements);
/**
* Adds elements to {@link FeatureSetRule#features() features} set.
* @param elements An iterable of features elements
* @return {@code this} builder for use in a chained invocation
*/
BuildFinal addAllFeatures(Iterable elements);
/**
* Builds a new {@link ImmutableFeatureSetRule ImmutableFeatureSetRule}.
* @return An immutable instance of FeatureSetRule
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
ImmutableFeatureSetRule build();
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
@SuppressWarnings("unchecked")
private static > Set createUnmodifiableEnumSet(Iterable iterable) {
if (iterable instanceof EnumSet>) {
return Collections.unmodifiableSet(EnumSet.copyOf((EnumSet) iterable));
}
List list = createSafeList(iterable, true, false);
switch(list.size()) {
case 0: return Collections.emptySet();
case 1: return Collections.singleton(list.get(0));
default: return Collections.unmodifiableSet(EnumSet.copyOf(list));
}
}
}