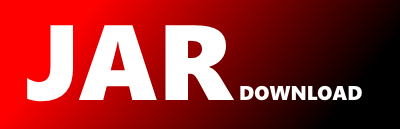
de.flapdoodle.embed.mongo.packageresolver.ImmutableUrlTemplatePackageFinder Maven / Gradle / Ivy
package de.flapdoodle.embed.mongo.packageresolver;
import de.flapdoodle.embed.process.config.store.FileSet;
import de.flapdoodle.embed.process.distribution.ArchiveType;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Immutable implementation of {@link UrlTemplatePackageFinder}.
*
* Use the builder to create immutable instances:
* {@code ImmutableUrlTemplatePackageFinder.builder()}.
*/
@SuppressWarnings({"all"})
public final class ImmutableUrlTemplatePackageFinder
extends UrlTemplatePackageFinder {
private final ArchiveType archiveType;
private final FileSet fileSet;
private final String urlTemplate;
private final boolean isDevVersion;
private ImmutableUrlTemplatePackageFinder(ImmutableUrlTemplatePackageFinder.Builder builder) {
this.archiveType = builder.archiveType;
this.fileSet = builder.fileSet;
this.urlTemplate = builder.urlTemplate;
this.isDevVersion = builder.isDevVersionIsSet()
? builder.isDevVersion
: super.isDevVersion();
}
private ImmutableUrlTemplatePackageFinder(
ArchiveType archiveType,
FileSet fileSet,
String urlTemplate,
boolean isDevVersion) {
this.archiveType = archiveType;
this.fileSet = fileSet;
this.urlTemplate = urlTemplate;
this.isDevVersion = isDevVersion;
}
/**
* @return The value of the {@code archiveType} attribute
*/
@Override
protected ArchiveType archiveType() {
return archiveType;
}
/**
* @return The value of the {@code fileSet} attribute
*/
@Override
protected FileSet fileSet() {
return fileSet;
}
/**
* @return The value of the {@code urlTemplate} attribute
*/
@Override
String urlTemplate() {
return urlTemplate;
}
/**
* @return The value of the {@code isDevVersion} attribute
*/
@Override
protected boolean isDevVersion() {
return isDevVersion;
}
/**
* Copy the current immutable object by setting a value for the {@link UrlTemplatePackageFinder#archiveType() archiveType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for archiveType
* @return A modified copy of the {@code this} object
*/
public final ImmutableUrlTemplatePackageFinder withArchiveType(ArchiveType value) {
ArchiveType newValue = Objects.requireNonNull(value, "archiveType");
if (this.archiveType == newValue) return this;
return new ImmutableUrlTemplatePackageFinder(newValue, this.fileSet, this.urlTemplate, this.isDevVersion);
}
/**
* Copy the current immutable object by setting a value for the {@link UrlTemplatePackageFinder#fileSet() fileSet} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for fileSet
* @return A modified copy of the {@code this} object
*/
public final ImmutableUrlTemplatePackageFinder withFileSet(FileSet value) {
if (this.fileSet == value) return this;
FileSet newValue = Objects.requireNonNull(value, "fileSet");
return new ImmutableUrlTemplatePackageFinder(this.archiveType, newValue, this.urlTemplate, this.isDevVersion);
}
/**
* Copy the current immutable object by setting a value for the {@link UrlTemplatePackageFinder#urlTemplate() urlTemplate} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for urlTemplate
* @return A modified copy of the {@code this} object
*/
public final ImmutableUrlTemplatePackageFinder withUrlTemplate(String value) {
String newValue = Objects.requireNonNull(value, "urlTemplate");
if (this.urlTemplate.equals(newValue)) return this;
return new ImmutableUrlTemplatePackageFinder(this.archiveType, this.fileSet, newValue, this.isDevVersion);
}
/**
* Copy the current immutable object by setting a value for the {@link UrlTemplatePackageFinder#isDevVersion() isDevVersion} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isDevVersion
* @return A modified copy of the {@code this} object
*/
public final ImmutableUrlTemplatePackageFinder withIsDevVersion(boolean value) {
if (this.isDevVersion == value) return this;
return new ImmutableUrlTemplatePackageFinder(this.archiveType, this.fileSet, this.urlTemplate, value);
}
/**
* This instance is equal to all instances of {@code ImmutableUrlTemplatePackageFinder} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableUrlTemplatePackageFinder
&& equalTo(0, (ImmutableUrlTemplatePackageFinder) another);
}
private boolean equalTo(int synthetic, ImmutableUrlTemplatePackageFinder another) {
return archiveType.equals(another.archiveType)
&& fileSet.equals(another.fileSet)
&& urlTemplate.equals(another.urlTemplate)
&& isDevVersion == another.isDevVersion;
}
/**
* Computes a hash code from attributes: {@code archiveType}, {@code fileSet}, {@code urlTemplate}, {@code isDevVersion}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + archiveType.hashCode();
h += (h << 5) + fileSet.hashCode();
h += (h << 5) + urlTemplate.hashCode();
h += (h << 5) + Boolean.hashCode(isDevVersion);
return h;
}
/**
* Prints the immutable value {@code UrlTemplatePackageFinder} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "UrlTemplatePackageFinder{"
+ "archiveType=" + archiveType
+ ", fileSet=" + fileSet
+ ", urlTemplate=" + urlTemplate
+ ", isDevVersion=" + isDevVersion
+ "}";
}
/**
* Creates an immutable copy of a {@link UrlTemplatePackageFinder} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable UrlTemplatePackageFinder instance
*/
public static ImmutableUrlTemplatePackageFinder copyOf(UrlTemplatePackageFinder instance) {
if (instance instanceof ImmutableUrlTemplatePackageFinder) {
return (ImmutableUrlTemplatePackageFinder) instance;
}
return ImmutableUrlTemplatePackageFinder.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableUrlTemplatePackageFinder ImmutableUrlTemplatePackageFinder}.
*
* ImmutableUrlTemplatePackageFinder.builder()
* .archiveType(de.flapdoodle.embed.process.distribution.ArchiveType) // required {@link UrlTemplatePackageFinder#archiveType() archiveType}
* .fileSet(de.flapdoodle.embed.process.config.store.FileSet) // required {@link UrlTemplatePackageFinder#fileSet() fileSet}
* .urlTemplate(String) // required {@link UrlTemplatePackageFinder#urlTemplate() urlTemplate}
* .isDevVersion(boolean) // optional {@link UrlTemplatePackageFinder#isDevVersion() isDevVersion}
* .build();
*
* @return A new ImmutableUrlTemplatePackageFinder builder
*/
public static ImmutableUrlTemplatePackageFinder.Builder builder() {
return new ImmutableUrlTemplatePackageFinder.Builder();
}
/**
* Builds instances of type {@link ImmutableUrlTemplatePackageFinder ImmutableUrlTemplatePackageFinder}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_ARCHIVE_TYPE = 0x1L;
private static final long INIT_BIT_FILE_SET = 0x2L;
private static final long INIT_BIT_URL_TEMPLATE = 0x4L;
private static final long OPT_BIT_IS_DEV_VERSION = 0x1L;
private long initBits = 0x7L;
private long optBits;
private ArchiveType archiveType;
private FileSet fileSet;
private String urlTemplate;
private boolean isDevVersion;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code UrlTemplatePackageFinder} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(UrlTemplatePackageFinder instance) {
Objects.requireNonNull(instance, "instance");
this.archiveType(instance.archiveType());
this.fileSet(instance.fileSet());
this.urlTemplate(instance.urlTemplate());
this.isDevVersion(instance.isDevVersion());
return this;
}
/**
* Initializes the value for the {@link UrlTemplatePackageFinder#archiveType() archiveType} attribute.
* @param archiveType The value for archiveType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder archiveType(ArchiveType archiveType) {
this.archiveType = Objects.requireNonNull(archiveType, "archiveType");
initBits &= ~INIT_BIT_ARCHIVE_TYPE;
return this;
}
/**
* Initializes the value for the {@link UrlTemplatePackageFinder#fileSet() fileSet} attribute.
* @param fileSet The value for fileSet
* @return {@code this} builder for use in a chained invocation
*/
public final Builder fileSet(FileSet fileSet) {
this.fileSet = Objects.requireNonNull(fileSet, "fileSet");
initBits &= ~INIT_BIT_FILE_SET;
return this;
}
/**
* Initializes the value for the {@link UrlTemplatePackageFinder#urlTemplate() urlTemplate} attribute.
* @param urlTemplate The value for urlTemplate
* @return {@code this} builder for use in a chained invocation
*/
public final Builder urlTemplate(String urlTemplate) {
this.urlTemplate = Objects.requireNonNull(urlTemplate, "urlTemplate");
initBits &= ~INIT_BIT_URL_TEMPLATE;
return this;
}
/**
* Initializes the value for the {@link UrlTemplatePackageFinder#isDevVersion() isDevVersion} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link UrlTemplatePackageFinder#isDevVersion() isDevVersion}.
* @param isDevVersion The value for isDevVersion
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isDevVersion(boolean isDevVersion) {
this.isDevVersion = isDevVersion;
optBits |= OPT_BIT_IS_DEV_VERSION;
return this;
}
/**
* Builds a new {@link ImmutableUrlTemplatePackageFinder ImmutableUrlTemplatePackageFinder}.
* @return An immutable instance of UrlTemplatePackageFinder
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableUrlTemplatePackageFinder build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableUrlTemplatePackageFinder(this);
}
private boolean isDevVersionIsSet() {
return (optBits & OPT_BIT_IS_DEV_VERSION) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ARCHIVE_TYPE) != 0) attributes.add("archiveType");
if ((initBits & INIT_BIT_FILE_SET) != 0) attributes.add("fileSet");
if ((initBits & INIT_BIT_URL_TEMPLATE) != 0) attributes.add("urlTemplate");
return "Cannot build UrlTemplatePackageFinder, some of required attributes are not set " + attributes;
}
}
}