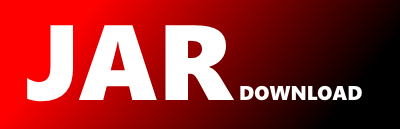
de.florianmichael.rclasses.functional.tuple.mutable.MutableSeptet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of functional Show documentation
Show all versions of functional Show documentation
Random collection of Java classes and utils in different submodules which together form a commons library
/*
* This file is part of RClasses - https://github.com/FlorianMichael/RClasses
* Copyright (C) 2023 FlorianMichael/EnZaXD and contributors
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.florianmichael.rclasses.functional.tuple.mutable;
import de.florianmichael.rclasses.functional.tuple.Septet;
import java.util.Objects;
/**
* Represents a mutable tuple of seven elements.
*/
public final class MutableSeptet extends Septet {
private A first;
private B second;
private C third;
private D fourth;
private E fifth;
private F sixth;
private G seventh;
public MutableSeptet() {
this(null, null, null, null, null, null, null);
}
public MutableSeptet(final A first, final B second, final C third, final D fourth, final E fifth, final F sixth, final G seventh) {
this.first = first;
this.second = second;
this.third = third;
this.fourth = fourth;
this.fifth = fifth;
this.sixth = sixth;
this.seventh = seventh;
}
@Override
public A getFirst() {
return this.first;
}
@Override
public B getSecond() {
return this.second;
}
@Override
public C getThird() {
return this.third;
}
@Override
public D getFourth() {
return this.fourth;
}
@Override
public E getFifth() {
return this.fifth;
}
@Override
public F getSixth() {
return this.sixth;
}
@Override
public G getSeventh() {
return this.seventh;
}
@Override
public void setFirst(final A first) {
this.first = first;
}
@Override
public void setSecond(final B second) {
this.second = second;
}
@Override
public void setThird(final C third) {
this.third = third;
}
@Override
public void setFourth(final D fourth) {
this.fourth = fourth;
}
@Override
public void setFifth(final E fifth) {
this.fifth = fifth;
}
@Override
public void setSixth(final F sixth) {
this.sixth = sixth;
}
@Override
public void setSeventh(final G seventh) {
this.seventh = seventh;
}
@Override
public String toString() {
return "MutableSeptet{" +
"first=" + first +
", second=" + second +
", third=" + third +
", fourth=" + fourth +
", fifth=" + fifth +
", sixth=" + sixth +
", seventh=" + seventh +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MutableSeptet, ?, ?, ?, ?, ?, ?> that = (MutableSeptet, ?, ?, ?, ?, ?, ?>) o;
return Objects.equals(first, that.first) && Objects.equals(second, that.second) && Objects.equals(third, that.third) && Objects.equals(fourth, that.fourth) && Objects.equals(fifth, that.fifth) && Objects.equals(sixth, that.sixth) && Objects.equals(seventh, that.seventh);
}
@Override
public int hashCode() {
return Objects.hash(first, second, third, fourth, fifth, sixth, seventh);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy