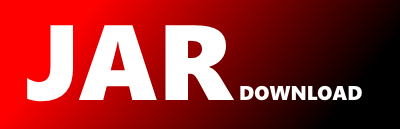
de.focus_shift.jollyday.jackson.mapping.Holidays Maven / Gradle / Ivy
Show all versions of jollyday-jackson Show documentation
package de.focus_shift.jollyday.jackson.mapping;
import com.fasterxml.jackson.dataformat.xml.annotation.JacksonXmlElementWrapper;
import java.util.ArrayList;
import java.util.List;
public class Holidays {
@JacksonXmlElementWrapper(useWrapping = false)
protected List fixed;
@JacksonXmlElementWrapper(useWrapping = false)
protected List relativeToFixed;
@JacksonXmlElementWrapper(useWrapping = false)
protected List relativeToWeekdayInMonth;
@JacksonXmlElementWrapper(useWrapping = false)
protected List fixedWeekday;
@JacksonXmlElementWrapper(useWrapping = false)
protected List christianHoliday;
@JacksonXmlElementWrapper(useWrapping = false)
protected List islamicHoliday;
@JacksonXmlElementWrapper(useWrapping = false)
protected List fixedWeekdayBetweenFixed;
@JacksonXmlElementWrapper(useWrapping = false)
protected List fixedWeekdayRelativeToFixed;
@JacksonXmlElementWrapper(useWrapping = false)
protected List ethiopianOrthodoxHoliday;
@JacksonXmlElementWrapper(useWrapping = false)
protected List relativeToEasterSunday;
/**
* Gets the value of the fixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fixed property.
*
*
* For example, to add a new item, do as follows:
*
* getFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Fixed }
*/
public List getFixed() {
if (fixed == null) {
fixed = new ArrayList<>();
}
return this.fixed;
}
/**
* Gets the value of the relativeToFixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the relativeToFixed property.
*
*
* For example, to add a new item, do as follows:
*
* getRelativeToFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelativeToFixed }
*/
public List getRelativeToFixed() {
if (relativeToFixed == null) {
relativeToFixed = new ArrayList<>();
}
return this.relativeToFixed;
}
/**
* Gets the value of the relativeToWeekdayInMonth property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the relativeToWeekdayInMonth property.
*
*
* For example, to add a new item, do as follows:
*
* getRelativeToWeekdayInMonth().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelativeToWeekdayInMonth }
*/
public List getRelativeToWeekdayInMonth() {
if (relativeToWeekdayInMonth == null) {
relativeToWeekdayInMonth = new ArrayList<>();
}
return this.relativeToWeekdayInMonth;
}
/**
* Gets the value of the fixedWeekday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fixedWeekday property.
*
*
* For example, to add a new item, do as follows:
*
* getFixedWeekday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FixedWeekdayInMonth }
*/
public List getFixedWeekday() {
if (fixedWeekday == null) {
fixedWeekday = new ArrayList<>();
}
return this.fixedWeekday;
}
/**
* Gets the value of the christianHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the christianHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getChristianHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ChristianHoliday }
*/
public List getChristianHoliday() {
if (christianHoliday == null) {
christianHoliday = new ArrayList<>();
}
return this.christianHoliday;
}
/**
* Gets the value of the islamicHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the islamicHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getIslamicHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IslamicHoliday }
*/
public List getIslamicHoliday() {
if (islamicHoliday == null) {
islamicHoliday = new ArrayList<>();
}
return this.islamicHoliday;
}
/**
* Gets the value of the fixedWeekdayBetweenFixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fixedWeekdayBetweenFixed property.
*
*
* For example, to add a new item, do as follows:
*
* getFixedWeekdayBetweenFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FixedWeekdayBetweenFixed }
*/
public List getFixedWeekdayBetweenFixed() {
if (fixedWeekdayBetweenFixed == null) {
fixedWeekdayBetweenFixed = new ArrayList<>();
}
return this.fixedWeekdayBetweenFixed;
}
/**
* Gets the value of the fixedWeekdayRelativeToFixed property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the fixedWeekdayRelativeToFixed property.
*
*
* For example, to add a new item, do as follows:
*
* getFixedWeekdayRelativeToFixed().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FixedWeekdayRelativeToFixed }
*/
public List getFixedWeekdayRelativeToFixed() {
if (fixedWeekdayRelativeToFixed == null) {
fixedWeekdayRelativeToFixed = new ArrayList<>();
}
return this.fixedWeekdayRelativeToFixed;
}
/**
* Gets the value of the ethiopianOrthodoxHoliday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the ethiopianOrthodoxHoliday property.
*
*
* For example, to add a new item, do as follows:
*
* getEthiopianOrthodoxHoliday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link EthiopianOrthodoxHoliday }
*/
public List getEthiopianOrthodoxHoliday() {
if (ethiopianOrthodoxHoliday == null) {
ethiopianOrthodoxHoliday = new ArrayList<>();
}
return this.ethiopianOrthodoxHoliday;
}
/**
* Gets the value of the relativeToEasterSunday property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the relativeToEasterSunday property.
*
*
* For example, to add a new item, do as follows:
*
* getRelativeToEasterSunday().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RelativeToEasterSunday }
*/
public List getRelativeToEasterSunday() {
if (relativeToEasterSunday == null) {
relativeToEasterSunday = new ArrayList<>();
}
return this.relativeToEasterSunday;
}
}