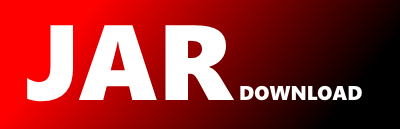
de.frachtwerk.essencium.backend.controller.AbstractRestController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of essencium-backend Show documentation
Show all versions of essencium-backend Show documentation
Essencium Backend is a software library built on top of Spring Boot that allows developers to quickly
get started on new software projects. Essencium provides, for example, a fully implemented role-rights concept
as well as various field-tested solutions for access management and authentication.
The newest version!
/*
* Copyright (C) 2024 Frachtwerk GmbH, Leopoldstraße 7C, 76133 Karlsruhe.
*
* This file is part of essencium-backend.
*
* essencium-backend is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* essencium-backend is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with essencium-backend. If not, see .
*/
package de.frachtwerk.essencium.backend.controller;
import de.frachtwerk.essencium.backend.model.AbstractBaseModel;
import de.frachtwerk.essencium.backend.model.representation.BasicRepresentation;
import de.frachtwerk.essencium.backend.service.AbstractEntityService;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.enums.ParameterIn;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
import jakarta.validation.Valid;
import jakarta.validation.constraints.NotNull;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
public abstract class AbstractRestController<
IN, ID extends Serializable, OUT extends AbstractBaseModel> {
protected final AbstractEntityService service;
protected AbstractRestController(AbstractEntityService service) {
this.service = service;
}
@GetMapping("/basic")
public List findAllBasic() {
return BasicRepresentation.from(service.getAll());
}
@GetMapping("/{id}")
@Parameter(
in = ParameterIn.PATH,
name = "id",
description = "ID of the entry to retrieve",
required = true,
content = @Content(schema = @Schema(type = "integer")))
public OUT findById(@PathVariable("id") @NotNull final ID id) {
return service.getById(id);
}
@PostMapping
@ResponseStatus(HttpStatus.CREATED)
public OUT create(@Valid @RequestBody @NotNull final IN input) {
return service.create(input);
}
@PutMapping(value = "/{id}")
@Parameter(
in = ParameterIn.PATH,
name = "id",
description = "ID of the entry to be updated",
required = true,
content = @Content(schema = @Schema(type = "integer")))
@ResponseStatus(HttpStatus.OK)
public OUT updateObject(
@PathVariable("id") @NotNull final ID id, @Valid @RequestBody @NotNull final IN input) {
return service.update(id, input);
}
@PatchMapping(value = "/{id}")
@Parameter(
in = ParameterIn.PATH,
name = "id",
description = "ID of the entry to be updated",
required = true,
content = @Content(schema = @Schema(type = "integer")))
@ResponseStatus(HttpStatus.OK)
public OUT patch(
@PathVariable("id") final ID id, @NotNull @RequestBody final Map fields) {
return service.patch(id, fields);
}
@DeleteMapping(value = "/{id}")
@Parameter(
in = ParameterIn.PATH,
name = "id",
description = "ID of the entry to be deleted",
required = true,
content = @Content(schema = @Schema(type = "integer")))
@ResponseStatus(HttpStatus.NO_CONTENT)
public void delete(@PathVariable("id") @NotNull final ID id) {
service.deleteById(id);
}
@RequestMapping(value = "/**", method = RequestMethod.OPTIONS)
public final ResponseEntity> collectionOptions() {
return ResponseEntity.ok().allow(getAllowedMethods().toArray(new HttpMethod[0])).build();
}
protected Set getAllowedMethods() {
return Set.of(
HttpMethod.GET,
HttpMethod.HEAD,
HttpMethod.POST,
HttpMethod.PUT,
HttpMethod.PATCH,
HttpMethod.DELETE,
HttpMethod.OPTIONS);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy