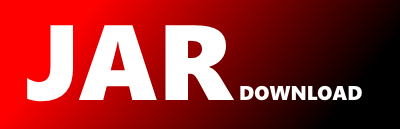
boomerang.Boomerang Maven / Gradle / Ivy
/**
* ***************************************************************************** Copyright (c) 2018
* Fraunhofer IEM, Paderborn, Germany. This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* SPDX-License-Identifier: EPL-2.0
*
*
Contributors: Johannes Spaeth - initial API and implementation
* *****************************************************************************
*/
package boomerang;
import boomerang.scene.CallGraph;
import boomerang.scene.ControlFlowGraph.Edge;
import boomerang.scene.DataFlowScope;
import boomerang.scene.Field;
import boomerang.scene.Val;
import sync.pds.solver.OneWeightFunctions;
import sync.pds.solver.WeightFunctions;
import wpds.impl.Weight;
public class Boomerang extends WeightedBoomerang {
private OneWeightFunctions fieldWeights;
private OneWeightFunctions callWeights;
public Boomerang(CallGraph callGraph, DataFlowScope scope) {
super(callGraph, scope);
}
public Boomerang(CallGraph callGraph, DataFlowScope scope, BoomerangOptions opt) {
super(callGraph, scope, opt);
}
@Override
protected WeightFunctions getForwardFieldWeights() {
return getOrCreateFieldWeights();
}
@Override
protected WeightFunctions getBackwardFieldWeights() {
return getOrCreateFieldWeights();
}
@Override
protected WeightFunctions getBackwardCallWeights() {
return getOrCreateCallWeights();
}
@Override
protected WeightFunctions getForwardCallWeights(
ForwardQuery sourceQuery) {
return getOrCreateCallWeights();
}
private WeightFunctions getOrCreateFieldWeights() {
if (fieldWeights == null) {
fieldWeights = new OneWeightFunctions<>(Weight.NO_WEIGHT_ONE);
}
return fieldWeights;
}
private WeightFunctions getOrCreateCallWeights() {
if (callWeights == null) {
callWeights = new OneWeightFunctions<>(Weight.NO_WEIGHT_ONE);
}
return callWeights;
}
}