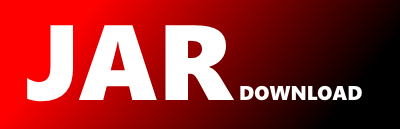
boomerang.util.DefaultValueMap Maven / Gradle / Ivy
/**
* ***************************************************************************** Copyright (c) 2018
* Fraunhofer IEM, Paderborn, Germany. This program and the accompanying materials are made
* available under the terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* SPDX-License-Identifier: EPL-2.0
*
*
Contributors: Johannes Spaeth - initial API and implementation
* *****************************************************************************
*/
package boomerang.util;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public abstract class DefaultValueMap implements Map {
private HashMap map;
public DefaultValueMap() {
map = new HashMap();
}
@Override
public int size() {
return map.size();
}
@Override
public boolean isEmpty() {
return map.isEmpty();
}
@Override
public boolean containsKey(Object key) {
return map.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return map.containsValue(value);
}
protected abstract V createItem(K key);
public V getOrCreate(K key) {
if (!map.containsKey(key)) {
V value = createItem((K) key);
map.put((K) key, value);
return value;
}
return map.get(key);
}
@Override
public V get(Object key) {
return map.get(key);
}
@Override
public V put(K key, V value) {
return map.put(key, value);
}
@Override
public V remove(Object key) {
return map.remove(key);
}
@Override
public void putAll(Map extends K, ? extends V> m) {
map.putAll(m);
}
@Override
public void clear() {
map.clear();
}
@Override
public Set keySet() {
return map.keySet();
}
@Override
public Collection values() {
return map.values();
}
@Override
public Set> entrySet() {
return map.entrySet();
}
}