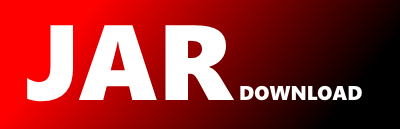
de.fraunhofer.iese.ind2uce.json.JacksonConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core.webservice Show documentation
Show all versions of core.webservice Show documentation
IND2UCE :: Webproject related contents like JSON, Autoconfiguration of Spring, etc.
/*-
* =================================LICENSE_START=================================
* IND2UCE
* %%
* Copyright (C) 2016 Fraunhofer IESE (www.iese.fraunhofer.de)
* %%
* The Fraunhofer-Gesellschaft zur Foerderung der angewandten Forschung e.V., Hansastrasse 27c, 80686 Munich, Germany (further: Fraunhofer) is holder of all proprietary rights on this computer program.
* You can only use this computer program if you have closed a license agreement with Fraunhofer or you get the right to use the computer program from someone who is authorized to grant you that right.
* Any use of the computer program without a valid license is prohibited and liable to prosecution.
*
* Copyright (C) 2018 Fraunhofer-Gesellschaft zur Foerderung der angewandten Forschung e.V. acting on behalf of its Fraunhofer Institute for Experimental Software Engineering (IESE)
*
* All rights reserved.
*
* Contact: [email protected]
* =================================LICENSE_END=================================
*/
package de.fraunhofer.iese.ind2uce.json;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.http.HttpInputMessage;
import org.springframework.http.HttpOutputMessage;
import org.springframework.http.MediaType;
import org.springframework.http.converter.json.AbstractJackson2HttpMessageConverter;
import org.springframework.http.converter.json.Jackson2ObjectMapperBuilder;
import java.io.IOException;
// TODO: Auto-generated Javadoc
/**
* Implementation of
* {@link org.springframework.http.converter.HttpMessageConverter
* HttpMessageConverter} that can read and write JSON using
* Jackson 2.x's {@link ObjectMapper}
* .
*
* This converter can be used to bind to typed beans, or untyped
* {@link java.util.HashMap HashMap} instances.
*
*
* By default, this converter supports {@code application/json} and
* {@code application/*+json}. This can be overridden by setting the
* {@link #setSupportedMediaTypes supportedMediaTypes} property.
*
*
* The default constructor uses the default configuration provided by
* {@link Jackson2ObjectMapperBuilder}.
*
* Compatible with Jackson 2.1 and higher.
*
*
* @author Arjen Poutsma
* @author Keith Donald
* @author Rossen Stoyanchev
* @author Juergen Hoeller
* @author Sebastien Deleuze
* @since 3.1.2
*/
public class JacksonConverter extends AbstractJackson2HttpMessageConverter {
/**
* Construct a new {@link JacksonConverter} using default configuration
* provided by {@link Jackson2ObjectMapperBuilder}.
*/
public JacksonConverter() {
this(Jackson2ObjectMapperBuilder.json().build());
}
/**
* Construct a new {@link JacksonConverter} with a custom {@link ObjectMapper}
* . You can use {@link Jackson2ObjectMapperBuilder} to build it easily.
*
* @param objectMapper the object mapper
* @see Jackson2ObjectMapperBuilder#json()
*/
public JacksonConverter(ObjectMapper objectMapper) {
super(objectMapper, new MediaType("application", "xml", DEFAULT_CHARSET));
}
/*
* (non-Javadoc)
* @see org.springframework.http.converter.json.
* AbstractJackson2HttpMessageConverter#writeInternal(java.lang.Object,
* org.springframework.http.HttpOutputMessage)
*/
@Override
public void writeInternal(Object object, HttpOutputMessage outputMessage) throws IOException {
super.writeInternal(object, outputMessage);
}
/*
* (non-Javadoc)
* @see org.springframework.http.converter.json.
* AbstractJackson2HttpMessageConverter#readInternal(java.lang.Class,
* org.springframework.http.HttpInputMessage)
*/
@Override
public Object readInternal(Class> clazz, HttpInputMessage inputMessage) throws IOException {
return super.readInternal(clazz, inputMessage);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy