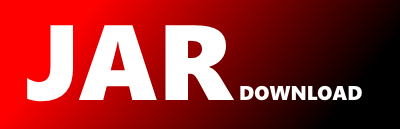
de.fraunhofer.iosb.ilt.frostserver.plugin.openapi.spec.OAComponents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of FROST-Server.Plugin.OpenApi Show documentation
Show all versions of FROST-Server.Plugin.OpenApi Show documentation
OpenAPI definition generator for the SensorThings API interface.
/*
* Copyright (C) 2024 Fraunhofer Institut IOSB, Fraunhoferstr. 1, D 76131
* Karlsruhe, Germany.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package de.fraunhofer.iosb.ilt.frostserver.plugin.openapi.spec;
import com.fasterxml.jackson.annotation.JsonInclude;
import java.util.Map;
import java.util.TreeMap;
/**
* An OpenAPI components object.
*
* @author scf
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
public final class OAComponents {
private Map schemas;
private Map parameters;
private Map responses;
private Map headers;
public Map getSchemas() {
return schemas;
}
public void addSchema(String name, OASchema schema) {
if (schemas == null) {
schemas = new TreeMap<>();
}
schemas.put(name, schema);
}
public boolean hasSchema(String name) {
if (schemas == null) {
return false;
}
return schemas.containsKey(name);
}
public OASchema getSchema(String name) {
if (schemas == null) {
return null;
}
return schemas.get(name);
}
public Map getParameters() {
return parameters;
}
public void addParameter(String name, OAParameter param) {
if (parameters == null) {
parameters = new TreeMap<>();
}
parameters.put(name, param);
}
public boolean hasParameter(String name) {
if (parameters == null) {
return false;
}
return parameters.containsKey(name);
}
public OAParameter getParameter(String name) {
if (parameters == null) {
return null;
}
return parameters.get(name);
}
public Map getResponses() {
return responses;
}
public void addResponse(String name, OAResponse value) {
if (responses == null) {
responses = new TreeMap<>();
}
responses.put(name, value);
}
public boolean hasResponse(String name) {
if (responses == null) {
return false;
}
return responses.containsKey(name);
}
public OAResponse getResponse(String name) {
if (responses == null) {
return null;
}
return responses.get(name);
}
public Map getHeaders() {
return headers;
}
public void addHeader(String name, OAHeader value) {
if (headers == null) {
headers = new TreeMap<>();
}
headers.put(name, value);
}
public boolean hasHeader(String name) {
if (headers == null) {
return false;
}
return headers.containsKey(name);
}
public OAHeader getHeader(String name) {
if (headers == null) {
return null;
}
return headers.get(name);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy