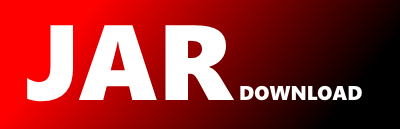
de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.relations.RelationManyToMany Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of FROST-Server.SQLjooq Show documentation
Show all versions of FROST-Server.SQLjooq Show documentation
SQL bindings for the FROST-Server.
/*
* Copyright (C) 2024 Fraunhofer Institut IOSB, Fraunhoferstr. 1, D 76131
* Karlsruhe, Germany.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.relations;
import static de.fraunhofer.iosb.ilt.frostserver.util.Constants.NOT_IMPLEMENTED_MULTI_VALUE_PK;
import de.fraunhofer.iosb.ilt.frostserver.model.EntityType;
import de.fraunhofer.iosb.ilt.frostserver.model.core.Entity;
import de.fraunhofer.iosb.ilt.frostserver.model.core.EntitySet;
import de.fraunhofer.iosb.ilt.frostserver.model.core.PkValue;
import de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.JooqPersistenceManager;
import de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.QueryBuilder;
import de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.tables.StaMainTable;
import de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.tables.StaTable;
import de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.utils.QueryState;
import de.fraunhofer.iosb.ilt.frostserver.persistence.pgjooq.utils.TableRef;
import de.fraunhofer.iosb.ilt.frostserver.property.NavigationPropertyMain;
import de.fraunhofer.iosb.ilt.frostserver.util.exception.IncompleteEntityException;
import de.fraunhofer.iosb.ilt.frostserver.util.exception.NoSuchEntityException;
import org.apache.commons.lang3.NotImplementedException;
import org.jooq.Condition;
import org.jooq.Field;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A relation from a source table to a target table.
*
* @author hylke
* @param The source table.
* @param The link table linking source and target entities.
* @param The target table.
*/
public class RelationManyToMany, L extends StaTable, T extends StaMainTable> implements Relation {
private static final Logger LOGGER = LoggerFactory.getLogger(RelationManyToMany.class.getName());
/**
* The navigation property this relation represents.
*/
private final NavigationPropertyMain navProp;
/**
* The target entity type of the relation.
*/
private final EntityType targetType;
/**
* The name of the relation. For official relations, this is the (singular)
* entity type name.
*/
private final String name;
/**
* The field on the source side that defines the relation.
*/
private FieldAccessor sourceFieldAcc;
private final L linkTable;
private FieldAccessor sourceLinkFieldAcc;
private FieldAccessor targetLinkFieldAcc;
/**
* The table that is the target side of the relation.
*/
private final T target;
/**
* The field on the target side that defines the relation.
*/
private FieldAccessor targetFieldAcc;
/**
* If the relation is symmetrical, on insert both [A,B] and [B,A] tuples are
* inserted, and on delete both are deleted.
*/
private boolean symmetrical;
public RelationManyToMany(NavigationPropertyMain navProp, S source, L linkTable, T target) {
this(navProp, source, linkTable, target, false);
}
public RelationManyToMany(NavigationPropertyMain navProp, S source, L linkTable, T target, boolean symmetrical) {
if (source == null) {
// Source is only used for finding the generics...
LOGGER.error("NULL source");
}
this.navProp = navProp;
this.linkTable = linkTable;
this.target = target;
this.targetType = navProp.getEntityType();
this.name = navProp.getName();
this.symmetrical = symmetrical;
if (source.getPkFields().size() != 1 || target.getPkFields().size() != 1) {
throw new NotImplementedException(NOT_IMPLEMENTED_MULTI_VALUE_PK);
}
}
public FieldAccessor getSourceFieldAcc() {
return sourceFieldAcc;
}
public RelationManyToMany setSourceFieldAcc(FieldAccessor sourceFieldAcc) {
this.sourceFieldAcc = sourceFieldAcc;
return this;
}
public L getLinkTable() {
return linkTable;
}
public FieldAccessor getSourceLinkFieldAcc() {
return sourceLinkFieldAcc;
}
public RelationManyToMany setSourceLinkFieldAcc(FieldAccessor sourceLinkFieldAcc) {
this.sourceLinkFieldAcc = sourceLinkFieldAcc;
return this;
}
public FieldAccessor getTargetLinkFieldAcc() {
return targetLinkFieldAcc;
}
public RelationManyToMany setTargetLinkFieldAcc(FieldAccessor targetLinkFieldAcc) {
this.targetLinkFieldAcc = targetLinkFieldAcc;
return this;
}
public FieldAccessor getTargetFieldAcc() {
return targetFieldAcc;
}
public RelationManyToMany setTargetFieldAcc(FieldAccessor targetFieldAcc) {
this.targetFieldAcc = targetFieldAcc;
return this;
}
public T getTarget() {
return target;
}
public NavigationPropertyMain getNavProp() {
return navProp;
}
public EntityType getTargetType() {
return targetType;
}
@Override
public TableRef join(S source, QueryState> queryState, TableRef sourceRef) {
L linkTableAliased = (L) linkTable.as(queryState.getNextAlias());
T targetAliased = (T) target.asSecure(queryState.getNextAlias(), queryState.getPersistenceManager());
Field
© 2015 - 2025 Weber Informatics LLC | Privacy Policy