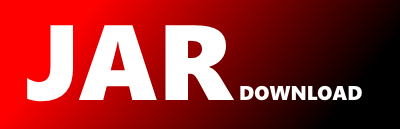
de.fraunhofer.iosb.ilt.configurableexample.FlagShapeList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ConfigurableExample Show documentation
Show all versions of ConfigurableExample Show documentation
An example of the use of the Configurable framework.
package de.fraunhofer.iosb.ilt.configurableexample;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.gson.JsonElement;
import de.fraunhofer.iosb.ilt.configurable.ConfigEditor;
import de.fraunhofer.iosb.ilt.configurable.Configurable;
import de.fraunhofer.iosb.ilt.configurable.ConfigurationException;
import de.fraunhofer.iosb.ilt.configurable.EditorFactory;
import de.fraunhofer.iosb.ilt.configurable.editor.EditorInt;
import de.fraunhofer.iosb.ilt.configurable.editor.EditorList;
import de.fraunhofer.iosb.ilt.configurable.editor.EditorMap;
import de.fraunhofer.iosb.ilt.configurable.editor.EditorSubclass;
/**
*
* @author scf
*/
public class FlagShapeList implements Configurable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy