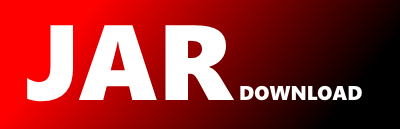
de.gematik.ws.conn.eventservice.v7.Event Maven / Gradle / Ivy
package de.gematik.ws.conn.eventservice.v7;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Topic" type="{http://ws.gematik.de/conn/EventService/v7.2}TopicType"/>
* <element name="Type" type="{http://ws.gematik.de/conn/EventService/v7.2}EventType"/>
* <element name="Severity" type="{http://ws.gematik.de/conn/EventService/v7.2}EventSeverityType"/>
* <element ref="{http://ws.gematik.de/conn/EventService/v7.2}SubscriptionID"/>
* <element name="Message">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Parameter" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Key">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="64"/>
* </restriction>
* </simpleType>
* </element>
* <element name="Value">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="5000"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"topic",
"type",
"severity",
"subscriptionID",
"message"
})
@XmlRootElement(name = "Event")
public class Event {
@XmlElement(name = "Topic", required = true)
protected String topic;
@XmlElement(name = "Type", required = true)
@XmlSchemaType(name = "token")
protected EventType type;
@XmlElement(name = "Severity", required = true)
@XmlSchemaType(name = "token")
protected EventSeverityType severity;
@XmlElement(name = "SubscriptionID", required = true)
protected String subscriptionID;
@XmlElement(name = "Message", required = true)
protected Event.Message message;
/**
* Gets the value of the topic property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTopic() {
return topic;
}
/**
* Sets the value of the topic property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTopic(String value) {
this.topic = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link EventType }
*
*/
public EventType getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link EventType }
*
*/
public void setType(EventType value) {
this.type = value;
}
/**
* Gets the value of the severity property.
*
* @return
* possible object is
* {@link EventSeverityType }
*
*/
public EventSeverityType getSeverity() {
return severity;
}
/**
* Sets the value of the severity property.
*
* @param value
* allowed object is
* {@link EventSeverityType }
*
*/
public void setSeverity(EventSeverityType value) {
this.severity = value;
}
/**
* Gets the value of the subscriptionID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSubscriptionID() {
return subscriptionID;
}
/**
* Sets the value of the subscriptionID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSubscriptionID(String value) {
this.subscriptionID = value;
}
/**
* Gets the value of the message property.
*
* @return
* possible object is
* {@link Event.Message }
*
*/
public Event.Message getMessage() {
return message;
}
/**
* Sets the value of the message property.
*
* @param value
* allowed object is
* {@link Event.Message }
*
*/
public void setMessage(Event.Message value) {
this.message = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Parameter" maxOccurs="unbounded" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Key">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="64"/>
* </restriction>
* </simpleType>
* </element>
* <element name="Value">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="5000"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"parameter"
})
public static class Message {
@XmlElement(name = "Parameter")
protected List parameter;
/**
* Gets the value of the parameter property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the parameter property.
*
*
* For example, to add a new item, do as follows:
*
* getParameter().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Event.Message.Parameter }
*
*
*/
public List getParameter() {
if (parameter == null) {
parameter = new ArrayList();
}
return this.parameter;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Key">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="64"/>
* </restriction>
* </simpleType>
* </element>
* <element name="Value">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <maxLength value="5000"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"key",
"value"
})
public static class Parameter {
@XmlElement(name = "Key", required = true)
protected String key;
@XmlElement(name = "Value", required = true)
protected String value;
/**
* Gets the value of the key property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKey() {
return key;
}
/**
* Sets the value of the key property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKey(String value) {
this.key = value;
}
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValue(String value) {
this.value = value;
}
}
}
}