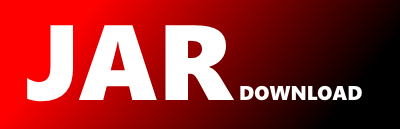
de.gematik.ws.conn.servicedirectory.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.0
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.11.11 at 11:20:41 AM CET
//
package de.gematik.ws.conn.servicedirectory;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the de.gematik.ws.conn.servicedirectory package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AAbstract_QNAME = new QName("http://ws.gematik.de/conn/ServiceDirectory/v3.1", "Abstract");
private final static QName _ProductInformation_QNAME = new QName("http://ws.gematik.de/int/version/ProductInformation/v1.1", "ProductInformation");
private final static QName _ServiceInformation_QNAME = new QName("http://ws.gematik.de/conn/ServiceInformation/v2.0", "ServiceInformation");
private final static QName _Abstract_QNAME = new QName("http://ws.gematik.de/conn/ServiceInformation/v2.0", "Abstract");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: de.gematik.ws.conn.servicedirectory
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ConnectorServices }
*
*/
public ConnectorServices createConnectorServices() {
return new ConnectorServices();
}
/**
* Create an instance of {@link ProductInformation }
*
*/
public ProductInformation createProductInformation() {
return new ProductInformation();
}
/**
* Create an instance of {@link ServicesType }
*
*/
public ServicesType createServicesType() {
return new ServicesType();
}
/**
* Create an instance of {@link EndpointType }
*
*/
public EndpointType createEndpointType() {
return new EndpointType();
}
/**
* Create an instance of {@link ServiceType }
*
*/
public ServiceType createServiceType() {
return new ServiceType();
}
/**
* Create an instance of {@link WSDLLocationType }
*
*/
public WSDLLocationType createWSDLLocationType() {
return new WSDLLocationType();
}
/**
* Create an instance of {@link VersionType }
*
*/
public VersionType createVersionType() {
return new VersionType();
}
/**
* Create an instance of {@link VersionsType }
*
*/
public VersionsType createVersionsType() {
return new VersionsType();
}
/**
* Create an instance of {@link ProductVersionLocal }
*
*/
public ProductVersionLocal createProductVersionLocal() {
return new ProductVersionLocal();
}
/**
* Create an instance of {@link ProductVersion }
*
*/
public ProductVersion createProductVersion() {
return new ProductVersion();
}
/**
* Create an instance of {@link ProductTypeInformation }
*
*/
public ProductTypeInformation createProductTypeInformation() {
return new ProductTypeInformation();
}
/**
* Create an instance of {@link ProductIdentification }
*
*/
public ProductIdentification createProductIdentification() {
return new ProductIdentification();
}
/**
* Create an instance of {@link ProductMiscellaneous }
*
*/
public ProductMiscellaneous createProductMiscellaneous() {
return new ProductMiscellaneous();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://ws.gematik.de/conn/ServiceDirectory/v3.1", name = "Abstract")
public JAXBElement createAAbstract(String value) {
return new JAXBElement(_AAbstract_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ProductInformation }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ProductInformation }{@code >}
*/
@XmlElementDecl(namespace = "http://ws.gematik.de/int/version/ProductInformation/v1.1", name = "ProductInformation")
public JAXBElement createProductInformation(ProductInformation value) {
return new JAXBElement(_ProductInformation_QNAME, ProductInformation.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ServicesType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ServicesType }{@code >}
*/
@XmlElementDecl(namespace = "http://ws.gematik.de/conn/ServiceInformation/v2.0", name = "ServiceInformation")
public JAXBElement createServiceInformation(ServicesType value) {
return new JAXBElement(_ServiceInformation_QNAME, ServicesType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://ws.gematik.de/conn/ServiceInformation/v2.0", name = "Abstract")
public JAXBElement createAbstract(String value) {
return new JAXBElement(_Abstract_QNAME, String.class, null, value);
}
}