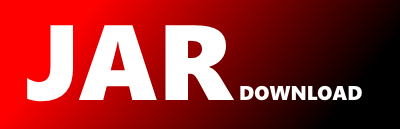
crosby.binary.Fileformat Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: fileformat.proto
package crosby.binary;
public final class Fileformat {
private Fileformat() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public interface BlobOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.Blob)
com.google.protobuf.MessageLiteOrBuilder {
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
boolean hasRaw();
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
com.google.protobuf.ByteString getRaw();
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
boolean hasRawSize();
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
int getRawSize();
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
boolean hasZlibData();
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
com.google.protobuf.ByteString getZlibData();
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
boolean hasLzmaData();
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
com.google.protobuf.ByteString getLzmaData();
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated boolean hasOBSOLETEBzip2Data();
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated com.google.protobuf.ByteString getOBSOLETEBzip2Data();
}
/**
* Protobuf type {@code OSMPBF.Blob}
*/
public static final class Blob extends
com.google.protobuf.GeneratedMessageLite<
Blob, Blob.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.Blob)
BlobOrBuilder {
private Blob() {
raw_ = com.google.protobuf.ByteString.EMPTY;
zlibData_ = com.google.protobuf.ByteString.EMPTY;
lzmaData_ = com.google.protobuf.ByteString.EMPTY;
oBSOLETEBzip2Data_ = com.google.protobuf.ByteString.EMPTY;
}
private int bitField0_;
public static final int RAW_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString raw_;
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
public boolean hasRaw() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
public com.google.protobuf.ByteString getRaw() {
return raw_;
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
private void setRaw(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
raw_ = value;
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
private void clearRaw() {
bitField0_ = (bitField0_ & ~0x00000001);
raw_ = getDefaultInstance().getRaw();
}
public static final int RAW_SIZE_FIELD_NUMBER = 2;
private int rawSize_;
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
public boolean hasRawSize() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
public int getRawSize() {
return rawSize_;
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
private void setRawSize(int value) {
bitField0_ |= 0x00000002;
rawSize_ = value;
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
private void clearRawSize() {
bitField0_ = (bitField0_ & ~0x00000002);
rawSize_ = 0;
}
public static final int ZLIB_DATA_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString zlibData_;
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
public boolean hasZlibData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
public com.google.protobuf.ByteString getZlibData() {
return zlibData_;
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
private void setZlibData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
zlibData_ = value;
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
private void clearZlibData() {
bitField0_ = (bitField0_ & ~0x00000004);
zlibData_ = getDefaultInstance().getZlibData();
}
public static final int LZMA_DATA_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString lzmaData_;
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
public boolean hasLzmaData() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
public com.google.protobuf.ByteString getLzmaData() {
return lzmaData_;
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
private void setLzmaData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
lzmaData_ = value;
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
private void clearLzmaData() {
bitField0_ = (bitField0_ & ~0x00000008);
lzmaData_ = getDefaultInstance().getLzmaData();
}
public static final int OBSOLETE_BZIP2_DATA_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString oBSOLETEBzip2Data_;
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasOBSOLETEBzip2Data() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString getOBSOLETEBzip2Data() {
return oBSOLETEBzip2Data_;
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
private void setOBSOLETEBzip2Data(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
oBSOLETEBzip2Data_ = value;
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
private void clearOBSOLETEBzip2Data() {
bitField0_ = (bitField0_ & ~0x00000010);
oBSOLETEBzip2Data_ = getDefaultInstance().getOBSOLETEBzip2Data();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, raw_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, rawSize_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, zlibData_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, lzmaData_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, oBSOLETEBzip2Data_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, raw_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, rawSize_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, zlibData_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, lzmaData_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, oBSOLETEBzip2Data_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Fileformat.Blob parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Fileformat.Blob parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Fileformat.Blob parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Fileformat.Blob parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Fileformat.Blob parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Fileformat.Blob parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Fileformat.Blob parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Fileformat.Blob parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Fileformat.Blob parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Fileformat.Blob parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Fileformat.Blob parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Fileformat.Blob parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Fileformat.Blob prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.Blob}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Fileformat.Blob, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.Blob)
crosby.binary.Fileformat.BlobOrBuilder {
// Construct using crosby.binary.Fileformat.Blob.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
public boolean hasRaw() {
return instance.hasRaw();
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
public com.google.protobuf.ByteString getRaw() {
return instance.getRaw();
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
public Builder setRaw(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setRaw(value);
return this;
}
/**
*
* No compression
*
*
* optional bytes raw = 1;
*/
public Builder clearRaw() {
copyOnWrite();
instance.clearRaw();
return this;
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
public boolean hasRawSize() {
return instance.hasRawSize();
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
public int getRawSize() {
return instance.getRawSize();
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
public Builder setRawSize(int value) {
copyOnWrite();
instance.setRawSize(value);
return this;
}
/**
*
* When compressed, the uncompressed size
*
*
* optional int32 raw_size = 2;
*/
public Builder clearRawSize() {
copyOnWrite();
instance.clearRawSize();
return this;
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
public boolean hasZlibData() {
return instance.hasZlibData();
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
public com.google.protobuf.ByteString getZlibData() {
return instance.getZlibData();
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
public Builder setZlibData(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setZlibData(value);
return this;
}
/**
*
* Possible compressed versions of the data.
*
*
* optional bytes zlib_data = 3;
*/
public Builder clearZlibData() {
copyOnWrite();
instance.clearZlibData();
return this;
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
public boolean hasLzmaData() {
return instance.hasLzmaData();
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
public com.google.protobuf.ByteString getLzmaData() {
return instance.getLzmaData();
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
public Builder setLzmaData(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setLzmaData(value);
return this;
}
/**
*
* PROPOSED feature for LZMA compressed data. SUPPORT IS NOT REQUIRED.
*
*
* optional bytes lzma_data = 4;
*/
public Builder clearLzmaData() {
copyOnWrite();
instance.clearLzmaData();
return this;
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasOBSOLETEBzip2Data() {
return instance.hasOBSOLETEBzip2Data();
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString getOBSOLETEBzip2Data() {
return instance.getOBSOLETEBzip2Data();
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated public Builder setOBSOLETEBzip2Data(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setOBSOLETEBzip2Data(value);
return this;
}
/**
*
* Formerly used for bzip2 compressed data. Depreciated in 2010.
*
*
* optional bytes OBSOLETE_bzip2_data = 5 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearOBSOLETEBzip2Data() {
copyOnWrite();
instance.clearOBSOLETEBzip2Data();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.Blob)
}
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Fileformat.Blob();
}
case IS_INITIALIZED: {
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Fileformat.Blob other = (crosby.binary.Fileformat.Blob) arg1;
raw_ = visitor.visitByteString(
hasRaw(), raw_,
other.hasRaw(), other.raw_);
rawSize_ = visitor.visitInt(
hasRawSize(), rawSize_,
other.hasRawSize(), other.rawSize_);
zlibData_ = visitor.visitByteString(
hasZlibData(), zlibData_,
other.hasZlibData(), other.zlibData_);
lzmaData_ = visitor.visitByteString(
hasLzmaData(), lzmaData_,
other.hasLzmaData(), other.lzmaData_);
oBSOLETEBzip2Data_ = visitor.visitByteString(
hasOBSOLETEBzip2Data(), oBSOLETEBzip2Data_,
other.hasOBSOLETEBzip2Data(), other.oBSOLETEBzip2Data_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
raw_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
rawSize_ = input.readInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
zlibData_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
lzmaData_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
oBSOLETEBzip2Data_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Fileformat.Blob.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.Blob)
private static final crosby.binary.Fileformat.Blob DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Blob();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Fileformat.Blob getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface BlobHeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.BlobHeader)
com.google.protobuf.MessageLiteOrBuilder {
/**
* required string type = 1;
*/
boolean hasType();
/**
* required string type = 1;
*/
java.lang.String getType();
/**
* required string type = 1;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* optional bytes indexdata = 2;
*/
boolean hasIndexdata();
/**
* optional bytes indexdata = 2;
*/
com.google.protobuf.ByteString getIndexdata();
/**
* required int32 datasize = 3;
*/
boolean hasDatasize();
/**
* required int32 datasize = 3;
*/
int getDatasize();
}
/**
* Protobuf type {@code OSMPBF.BlobHeader}
*/
public static final class BlobHeader extends
com.google.protobuf.GeneratedMessageLite<
BlobHeader, BlobHeader.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.BlobHeader)
BlobHeaderOrBuilder {
private BlobHeader() {
type_ = "";
indexdata_ = com.google.protobuf.ByteString.EMPTY;
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private java.lang.String type_;
/**
* required string type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string type = 1;
*/
public java.lang.String getType() {
return type_;
}
/**
* required string type = 1;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(type_);
}
/**
* required string type = 1;
*/
private void setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value;
}
/**
* required string type = 1;
*/
private void clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = getDefaultInstance().getType();
}
/**
* required string type = 1;
*/
private void setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.toStringUtf8();
}
public static final int INDEXDATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString indexdata_;
/**
* optional bytes indexdata = 2;
*/
public boolean hasIndexdata() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes indexdata = 2;
*/
public com.google.protobuf.ByteString getIndexdata() {
return indexdata_;
}
/**
* optional bytes indexdata = 2;
*/
private void setIndexdata(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
indexdata_ = value;
}
/**
* optional bytes indexdata = 2;
*/
private void clearIndexdata() {
bitField0_ = (bitField0_ & ~0x00000002);
indexdata_ = getDefaultInstance().getIndexdata();
}
public static final int DATASIZE_FIELD_NUMBER = 3;
private int datasize_;
/**
* required int32 datasize = 3;
*/
public boolean hasDatasize() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required int32 datasize = 3;
*/
public int getDatasize() {
return datasize_;
}
/**
* required int32 datasize = 3;
*/
private void setDatasize(int value) {
bitField0_ |= 0x00000004;
datasize_ = value;
}
/**
* required int32 datasize = 3;
*/
private void clearDatasize() {
bitField0_ = (bitField0_ & ~0x00000004);
datasize_ = 0;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeString(1, getType());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, indexdata_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, datasize_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getType());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, indexdata_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, datasize_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Fileformat.BlobHeader parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Fileformat.BlobHeader parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Fileformat.BlobHeader parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Fileformat.BlobHeader prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.BlobHeader}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Fileformat.BlobHeader, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.BlobHeader)
crosby.binary.Fileformat.BlobHeaderOrBuilder {
// Construct using crosby.binary.Fileformat.BlobHeader.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* required string type = 1;
*/
public boolean hasType() {
return instance.hasType();
}
/**
* required string type = 1;
*/
public java.lang.String getType() {
return instance.getType();
}
/**
* required string type = 1;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
return instance.getTypeBytes();
}
/**
* required string type = 1;
*/
public Builder setType(
java.lang.String value) {
copyOnWrite();
instance.setType(value);
return this;
}
/**
* required string type = 1;
*/
public Builder clearType() {
copyOnWrite();
instance.clearType();
return this;
}
/**
* required string type = 1;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setTypeBytes(value);
return this;
}
/**
* optional bytes indexdata = 2;
*/
public boolean hasIndexdata() {
return instance.hasIndexdata();
}
/**
* optional bytes indexdata = 2;
*/
public com.google.protobuf.ByteString getIndexdata() {
return instance.getIndexdata();
}
/**
* optional bytes indexdata = 2;
*/
public Builder setIndexdata(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setIndexdata(value);
return this;
}
/**
* optional bytes indexdata = 2;
*/
public Builder clearIndexdata() {
copyOnWrite();
instance.clearIndexdata();
return this;
}
/**
* required int32 datasize = 3;
*/
public boolean hasDatasize() {
return instance.hasDatasize();
}
/**
* required int32 datasize = 3;
*/
public int getDatasize() {
return instance.getDatasize();
}
/**
* required int32 datasize = 3;
*/
public Builder setDatasize(int value) {
copyOnWrite();
instance.setDatasize(value);
return this;
}
/**
* required int32 datasize = 3;
*/
public Builder clearDatasize() {
copyOnWrite();
instance.clearDatasize();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.BlobHeader)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Fileformat.BlobHeader();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasType()) {
return null;
}
if (!hasDatasize()) {
return null;
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Fileformat.BlobHeader other = (crosby.binary.Fileformat.BlobHeader) arg1;
type_ = visitor.visitString(
hasType(), type_,
other.hasType(), other.type_);
indexdata_ = visitor.visitByteString(
hasIndexdata(), indexdata_,
other.hasIndexdata(), other.indexdata_);
datasize_ = visitor.visitInt(
hasDatasize(), datasize_,
other.hasDatasize(), other.datasize_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readString();
bitField0_ |= 0x00000001;
type_ = s;
break;
}
case 18: {
bitField0_ |= 0x00000002;
indexdata_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
datasize_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Fileformat.BlobHeader.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.BlobHeader)
private static final crosby.binary.Fileformat.BlobHeader DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new BlobHeader();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Fileformat.BlobHeader getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
static {
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy