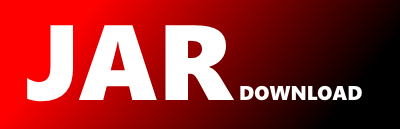
crosby.binary.Osmformat Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: osmformat.proto
package crosby.binary;
public final class Osmformat {
private Osmformat() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public interface HeaderBlockOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.HeaderBlock)
com.google.protobuf.MessageLiteOrBuilder {
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
boolean hasBbox();
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
crosby.binary.Osmformat.HeaderBBox getBbox();
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
java.util.List
getRequiredFeaturesList();
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
int getRequiredFeaturesCount();
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
java.lang.String getRequiredFeatures(int index);
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
com.google.protobuf.ByteString
getRequiredFeaturesBytes(int index);
/**
* repeated string optional_features = 5;
*/
java.util.List
getOptionalFeaturesList();
/**
* repeated string optional_features = 5;
*/
int getOptionalFeaturesCount();
/**
* repeated string optional_features = 5;
*/
java.lang.String getOptionalFeatures(int index);
/**
* repeated string optional_features = 5;
*/
com.google.protobuf.ByteString
getOptionalFeaturesBytes(int index);
/**
* optional string writingprogram = 16;
*/
boolean hasWritingprogram();
/**
* optional string writingprogram = 16;
*/
java.lang.String getWritingprogram();
/**
* optional string writingprogram = 16;
*/
com.google.protobuf.ByteString
getWritingprogramBytes();
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
boolean hasSource();
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
java.lang.String getSource();
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
com.google.protobuf.ByteString
getSourceBytes();
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
boolean hasOsmosisReplicationTimestamp();
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
long getOsmosisReplicationTimestamp();
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
boolean hasOsmosisReplicationSequenceNumber();
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
long getOsmosisReplicationSequenceNumber();
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
boolean hasOsmosisReplicationBaseUrl();
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
java.lang.String getOsmosisReplicationBaseUrl();
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
com.google.protobuf.ByteString
getOsmosisReplicationBaseUrlBytes();
}
/**
* Protobuf type {@code OSMPBF.HeaderBlock}
*/
public static final class HeaderBlock extends
com.google.protobuf.GeneratedMessageLite<
HeaderBlock, HeaderBlock.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.HeaderBlock)
HeaderBlockOrBuilder {
private HeaderBlock() {
requiredFeatures_ = com.google.protobuf.GeneratedMessageLite.emptyProtobufList();
optionalFeatures_ = com.google.protobuf.GeneratedMessageLite.emptyProtobufList();
writingprogram_ = "";
source_ = "";
osmosisReplicationBaseUrl_ = "";
}
private int bitField0_;
public static final int BBOX_FIELD_NUMBER = 1;
private crosby.binary.Osmformat.HeaderBBox bbox_;
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public boolean hasBbox() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public crosby.binary.Osmformat.HeaderBBox getBbox() {
return bbox_ == null ? crosby.binary.Osmformat.HeaderBBox.getDefaultInstance() : bbox_;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
private void setBbox(crosby.binary.Osmformat.HeaderBBox value) {
if (value == null) {
throw new NullPointerException();
}
bbox_ = value;
bitField0_ |= 0x00000001;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
private void setBbox(
crosby.binary.Osmformat.HeaderBBox.Builder builderForValue) {
bbox_ = builderForValue.build();
bitField0_ |= 0x00000001;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
private void mergeBbox(crosby.binary.Osmformat.HeaderBBox value) {
if (bbox_ != null &&
bbox_ != crosby.binary.Osmformat.HeaderBBox.getDefaultInstance()) {
bbox_ =
crosby.binary.Osmformat.HeaderBBox.newBuilder(bbox_).mergeFrom(value).buildPartial();
} else {
bbox_ = value;
}
bitField0_ |= 0x00000001;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
private void clearBbox() { bbox_ = null;
bitField0_ = (bitField0_ & ~0x00000001);
}
public static final int REQUIRED_FEATURES_FIELD_NUMBER = 4;
private com.google.protobuf.Internal.ProtobufList requiredFeatures_;
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public java.util.List getRequiredFeaturesList() {
return requiredFeatures_;
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public int getRequiredFeaturesCount() {
return requiredFeatures_.size();
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public java.lang.String getRequiredFeatures(int index) {
return requiredFeatures_.get(index);
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public com.google.protobuf.ByteString
getRequiredFeaturesBytes(int index) {
return com.google.protobuf.ByteString.copyFromUtf8(
requiredFeatures_.get(index));
}
private void ensureRequiredFeaturesIsMutable() {
if (!requiredFeatures_.isModifiable()) {
requiredFeatures_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(requiredFeatures_);
}
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
private void setRequiredFeatures(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRequiredFeaturesIsMutable();
requiredFeatures_.set(index, value);
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
private void addRequiredFeatures(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRequiredFeaturesIsMutable();
requiredFeatures_.add(value);
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
private void addAllRequiredFeatures(
java.lang.Iterable values) {
ensureRequiredFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, requiredFeatures_);
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
private void clearRequiredFeatures() {
requiredFeatures_ = com.google.protobuf.GeneratedMessageLite.emptyProtobufList();
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
private void addRequiredFeaturesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRequiredFeaturesIsMutable();
requiredFeatures_.add(value.toStringUtf8());
}
public static final int OPTIONAL_FEATURES_FIELD_NUMBER = 5;
private com.google.protobuf.Internal.ProtobufList optionalFeatures_;
/**
* repeated string optional_features = 5;
*/
public java.util.List getOptionalFeaturesList() {
return optionalFeatures_;
}
/**
* repeated string optional_features = 5;
*/
public int getOptionalFeaturesCount() {
return optionalFeatures_.size();
}
/**
* repeated string optional_features = 5;
*/
public java.lang.String getOptionalFeatures(int index) {
return optionalFeatures_.get(index);
}
/**
* repeated string optional_features = 5;
*/
public com.google.protobuf.ByteString
getOptionalFeaturesBytes(int index) {
return com.google.protobuf.ByteString.copyFromUtf8(
optionalFeatures_.get(index));
}
private void ensureOptionalFeaturesIsMutable() {
if (!optionalFeatures_.isModifiable()) {
optionalFeatures_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(optionalFeatures_);
}
}
/**
* repeated string optional_features = 5;
*/
private void setOptionalFeatures(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalFeaturesIsMutable();
optionalFeatures_.set(index, value);
}
/**
* repeated string optional_features = 5;
*/
private void addOptionalFeatures(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalFeaturesIsMutable();
optionalFeatures_.add(value);
}
/**
* repeated string optional_features = 5;
*/
private void addAllOptionalFeatures(
java.lang.Iterable values) {
ensureOptionalFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, optionalFeatures_);
}
/**
* repeated string optional_features = 5;
*/
private void clearOptionalFeatures() {
optionalFeatures_ = com.google.protobuf.GeneratedMessageLite.emptyProtobufList();
}
/**
* repeated string optional_features = 5;
*/
private void addOptionalFeaturesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalFeaturesIsMutable();
optionalFeatures_.add(value.toStringUtf8());
}
public static final int WRITINGPROGRAM_FIELD_NUMBER = 16;
private java.lang.String writingprogram_;
/**
* optional string writingprogram = 16;
*/
public boolean hasWritingprogram() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string writingprogram = 16;
*/
public java.lang.String getWritingprogram() {
return writingprogram_;
}
/**
* optional string writingprogram = 16;
*/
public com.google.protobuf.ByteString
getWritingprogramBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(writingprogram_);
}
/**
* optional string writingprogram = 16;
*/
private void setWritingprogram(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
writingprogram_ = value;
}
/**
* optional string writingprogram = 16;
*/
private void clearWritingprogram() {
bitField0_ = (bitField0_ & ~0x00000002);
writingprogram_ = getDefaultInstance().getWritingprogram();
}
/**
* optional string writingprogram = 16;
*/
private void setWritingprogramBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
writingprogram_ = value.toStringUtf8();
}
public static final int SOURCE_FIELD_NUMBER = 17;
private java.lang.String source_;
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public boolean hasSource() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public java.lang.String getSource() {
return source_;
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public com.google.protobuf.ByteString
getSourceBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(source_);
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
private void setSource(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
source_ = value;
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
private void clearSource() {
bitField0_ = (bitField0_ & ~0x00000004);
source_ = getDefaultInstance().getSource();
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
private void setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
source_ = value.toStringUtf8();
}
public static final int OSMOSIS_REPLICATION_TIMESTAMP_FIELD_NUMBER = 32;
private long osmosisReplicationTimestamp_;
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
public boolean hasOsmosisReplicationTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
public long getOsmosisReplicationTimestamp() {
return osmosisReplicationTimestamp_;
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
private void setOsmosisReplicationTimestamp(long value) {
bitField0_ |= 0x00000008;
osmosisReplicationTimestamp_ = value;
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
private void clearOsmosisReplicationTimestamp() {
bitField0_ = (bitField0_ & ~0x00000008);
osmosisReplicationTimestamp_ = 0L;
}
public static final int OSMOSIS_REPLICATION_SEQUENCE_NUMBER_FIELD_NUMBER = 33;
private long osmosisReplicationSequenceNumber_;
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
public boolean hasOsmosisReplicationSequenceNumber() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
public long getOsmosisReplicationSequenceNumber() {
return osmosisReplicationSequenceNumber_;
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
private void setOsmosisReplicationSequenceNumber(long value) {
bitField0_ |= 0x00000010;
osmosisReplicationSequenceNumber_ = value;
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
private void clearOsmosisReplicationSequenceNumber() {
bitField0_ = (bitField0_ & ~0x00000010);
osmosisReplicationSequenceNumber_ = 0L;
}
public static final int OSMOSIS_REPLICATION_BASE_URL_FIELD_NUMBER = 34;
private java.lang.String osmosisReplicationBaseUrl_;
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public boolean hasOsmosisReplicationBaseUrl() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public java.lang.String getOsmosisReplicationBaseUrl() {
return osmosisReplicationBaseUrl_;
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public com.google.protobuf.ByteString
getOsmosisReplicationBaseUrlBytes() {
return com.google.protobuf.ByteString.copyFromUtf8(osmosisReplicationBaseUrl_);
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
private void setOsmosisReplicationBaseUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
osmosisReplicationBaseUrl_ = value;
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
private void clearOsmosisReplicationBaseUrl() {
bitField0_ = (bitField0_ & ~0x00000020);
osmosisReplicationBaseUrl_ = getDefaultInstance().getOsmosisReplicationBaseUrl();
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
private void setOsmosisReplicationBaseUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
osmosisReplicationBaseUrl_ = value.toStringUtf8();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getBbox());
}
for (int i = 0; i < requiredFeatures_.size(); i++) {
output.writeString(4, requiredFeatures_.get(i));
}
for (int i = 0; i < optionalFeatures_.size(); i++) {
output.writeString(5, optionalFeatures_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeString(16, getWritingprogram());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeString(17, getSource());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(32, osmosisReplicationTimestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt64(33, osmosisReplicationSequenceNumber_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeString(34, getOsmosisReplicationBaseUrl());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getBbox());
}
{
int dataSize = 0;
for (int i = 0; i < requiredFeatures_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(requiredFeatures_.get(i));
}
size += dataSize;
size += 1 * getRequiredFeaturesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < optionalFeatures_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeStringSizeNoTag(optionalFeatures_.get(i));
}
size += dataSize;
size += 1 * getOptionalFeaturesList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(16, getWritingprogram());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(17, getSource());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(32, osmosisReplicationTimestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(33, osmosisReplicationSequenceNumber_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(34, getOsmosisReplicationBaseUrl());
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBlock parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.HeaderBlock parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.HeaderBlock parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.HeaderBlock prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.HeaderBlock}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.HeaderBlock, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.HeaderBlock)
crosby.binary.Osmformat.HeaderBlockOrBuilder {
// Construct using crosby.binary.Osmformat.HeaderBlock.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public boolean hasBbox() {
return instance.hasBbox();
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public crosby.binary.Osmformat.HeaderBBox getBbox() {
return instance.getBbox();
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public Builder setBbox(crosby.binary.Osmformat.HeaderBBox value) {
copyOnWrite();
instance.setBbox(value);
return this;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public Builder setBbox(
crosby.binary.Osmformat.HeaderBBox.Builder builderForValue) {
copyOnWrite();
instance.setBbox(builderForValue);
return this;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public Builder mergeBbox(crosby.binary.Osmformat.HeaderBBox value) {
copyOnWrite();
instance.mergeBbox(value);
return this;
}
/**
* optional .OSMPBF.HeaderBBox bbox = 1;
*/
public Builder clearBbox() { copyOnWrite();
instance.clearBbox();
return this;
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public java.util.List
getRequiredFeaturesList() {
return java.util.Collections.unmodifiableList(
instance.getRequiredFeaturesList());
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public int getRequiredFeaturesCount() {
return instance.getRequiredFeaturesCount();
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public java.lang.String getRequiredFeatures(int index) {
return instance.getRequiredFeatures(index);
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public com.google.protobuf.ByteString
getRequiredFeaturesBytes(int index) {
return instance.getRequiredFeaturesBytes(index);
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public Builder setRequiredFeatures(
int index, java.lang.String value) {
copyOnWrite();
instance.setRequiredFeatures(index, value);
return this;
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public Builder addRequiredFeatures(
java.lang.String value) {
copyOnWrite();
instance.addRequiredFeatures(value);
return this;
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public Builder addAllRequiredFeatures(
java.lang.Iterable values) {
copyOnWrite();
instance.addAllRequiredFeatures(values);
return this;
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public Builder clearRequiredFeatures() {
copyOnWrite();
instance.clearRequiredFeatures();
return this;
}
/**
*
* Additional tags to aid in parsing this dataset
*
*
* repeated string required_features = 4;
*/
public Builder addRequiredFeaturesBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.addRequiredFeaturesBytes(value);
return this;
}
/**
* repeated string optional_features = 5;
*/
public java.util.List
getOptionalFeaturesList() {
return java.util.Collections.unmodifiableList(
instance.getOptionalFeaturesList());
}
/**
* repeated string optional_features = 5;
*/
public int getOptionalFeaturesCount() {
return instance.getOptionalFeaturesCount();
}
/**
* repeated string optional_features = 5;
*/
public java.lang.String getOptionalFeatures(int index) {
return instance.getOptionalFeatures(index);
}
/**
* repeated string optional_features = 5;
*/
public com.google.protobuf.ByteString
getOptionalFeaturesBytes(int index) {
return instance.getOptionalFeaturesBytes(index);
}
/**
* repeated string optional_features = 5;
*/
public Builder setOptionalFeatures(
int index, java.lang.String value) {
copyOnWrite();
instance.setOptionalFeatures(index, value);
return this;
}
/**
* repeated string optional_features = 5;
*/
public Builder addOptionalFeatures(
java.lang.String value) {
copyOnWrite();
instance.addOptionalFeatures(value);
return this;
}
/**
* repeated string optional_features = 5;
*/
public Builder addAllOptionalFeatures(
java.lang.Iterable values) {
copyOnWrite();
instance.addAllOptionalFeatures(values);
return this;
}
/**
* repeated string optional_features = 5;
*/
public Builder clearOptionalFeatures() {
copyOnWrite();
instance.clearOptionalFeatures();
return this;
}
/**
* repeated string optional_features = 5;
*/
public Builder addOptionalFeaturesBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.addOptionalFeaturesBytes(value);
return this;
}
/**
* optional string writingprogram = 16;
*/
public boolean hasWritingprogram() {
return instance.hasWritingprogram();
}
/**
* optional string writingprogram = 16;
*/
public java.lang.String getWritingprogram() {
return instance.getWritingprogram();
}
/**
* optional string writingprogram = 16;
*/
public com.google.protobuf.ByteString
getWritingprogramBytes() {
return instance.getWritingprogramBytes();
}
/**
* optional string writingprogram = 16;
*/
public Builder setWritingprogram(
java.lang.String value) {
copyOnWrite();
instance.setWritingprogram(value);
return this;
}
/**
* optional string writingprogram = 16;
*/
public Builder clearWritingprogram() {
copyOnWrite();
instance.clearWritingprogram();
return this;
}
/**
* optional string writingprogram = 16;
*/
public Builder setWritingprogramBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setWritingprogramBytes(value);
return this;
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public boolean hasSource() {
return instance.hasSource();
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public java.lang.String getSource() {
return instance.getSource();
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public com.google.protobuf.ByteString
getSourceBytes() {
return instance.getSourceBytes();
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public Builder setSource(
java.lang.String value) {
copyOnWrite();
instance.setSource(value);
return this;
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public Builder clearSource() {
copyOnWrite();
instance.clearSource();
return this;
}
/**
*
* From the bbox field.
*
*
* optional string source = 17;
*/
public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setSourceBytes(value);
return this;
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
public boolean hasOsmosisReplicationTimestamp() {
return instance.hasOsmosisReplicationTimestamp();
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
public long getOsmosisReplicationTimestamp() {
return instance.getOsmosisReplicationTimestamp();
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
public Builder setOsmosisReplicationTimestamp(long value) {
copyOnWrite();
instance.setOsmosisReplicationTimestamp(value);
return this;
}
/**
*
* replication timestamp, expressed in seconds since the epoch,
* otherwise the same value as in the "timestamp=..." field
* in the state.txt file used by Osmosis
*
*
* optional int64 osmosis_replication_timestamp = 32;
*/
public Builder clearOsmosisReplicationTimestamp() {
copyOnWrite();
instance.clearOsmosisReplicationTimestamp();
return this;
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
public boolean hasOsmosisReplicationSequenceNumber() {
return instance.hasOsmosisReplicationSequenceNumber();
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
public long getOsmosisReplicationSequenceNumber() {
return instance.getOsmosisReplicationSequenceNumber();
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
public Builder setOsmosisReplicationSequenceNumber(long value) {
copyOnWrite();
instance.setOsmosisReplicationSequenceNumber(value);
return this;
}
/**
*
* replication sequence number (sequenceNumber in state.txt)
*
*
* optional int64 osmosis_replication_sequence_number = 33;
*/
public Builder clearOsmosisReplicationSequenceNumber() {
copyOnWrite();
instance.clearOsmosisReplicationSequenceNumber();
return this;
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public boolean hasOsmosisReplicationBaseUrl() {
return instance.hasOsmosisReplicationBaseUrl();
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public java.lang.String getOsmosisReplicationBaseUrl() {
return instance.getOsmosisReplicationBaseUrl();
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public com.google.protobuf.ByteString
getOsmosisReplicationBaseUrlBytes() {
return instance.getOsmosisReplicationBaseUrlBytes();
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public Builder setOsmosisReplicationBaseUrl(
java.lang.String value) {
copyOnWrite();
instance.setOsmosisReplicationBaseUrl(value);
return this;
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public Builder clearOsmosisReplicationBaseUrl() {
copyOnWrite();
instance.clearOsmosisReplicationBaseUrl();
return this;
}
/**
*
* replication base URL (from Osmosis' configuration.txt file)
*
*
* optional string osmosis_replication_base_url = 34;
*/
public Builder setOsmosisReplicationBaseUrlBytes(
com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setOsmosisReplicationBaseUrlBytes(value);
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.HeaderBlock)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.HeaderBlock();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (hasBbox()) {
if (!getBbox().isInitialized()) {
return null;
}
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
requiredFeatures_.makeImmutable();
optionalFeatures_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.HeaderBlock other = (crosby.binary.Osmformat.HeaderBlock) arg1;
bbox_ = visitor.visitMessage(bbox_, other.bbox_);
requiredFeatures_= visitor.visitList(requiredFeatures_, other.requiredFeatures_);
optionalFeatures_= visitor.visitList(optionalFeatures_, other.optionalFeatures_);
writingprogram_ = visitor.visitString(
hasWritingprogram(), writingprogram_,
other.hasWritingprogram(), other.writingprogram_);
source_ = visitor.visitString(
hasSource(), source_,
other.hasSource(), other.source_);
osmosisReplicationTimestamp_ = visitor.visitLong(
hasOsmosisReplicationTimestamp(), osmosisReplicationTimestamp_,
other.hasOsmosisReplicationTimestamp(), other.osmosisReplicationTimestamp_);
osmosisReplicationSequenceNumber_ = visitor.visitLong(
hasOsmosisReplicationSequenceNumber(), osmosisReplicationSequenceNumber_,
other.hasOsmosisReplicationSequenceNumber(), other.osmosisReplicationSequenceNumber_);
osmosisReplicationBaseUrl_ = visitor.visitString(
hasOsmosisReplicationBaseUrl(), osmosisReplicationBaseUrl_,
other.hasOsmosisReplicationBaseUrl(), other.osmosisReplicationBaseUrl_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 10: {
crosby.binary.Osmformat.HeaderBBox.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = bbox_.toBuilder();
}
bbox_ = input.readMessage(crosby.binary.Osmformat.HeaderBBox.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(bbox_);
bbox_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 34: {
java.lang.String s = input.readString();
if (!requiredFeatures_.isModifiable()) {
requiredFeatures_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(requiredFeatures_);
}
requiredFeatures_.add(s);
break;
}
case 42: {
java.lang.String s = input.readString();
if (!optionalFeatures_.isModifiable()) {
optionalFeatures_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(optionalFeatures_);
}
optionalFeatures_.add(s);
break;
}
case 130: {
java.lang.String s = input.readString();
bitField0_ |= 0x00000002;
writingprogram_ = s;
break;
}
case 138: {
java.lang.String s = input.readString();
bitField0_ |= 0x00000004;
source_ = s;
break;
}
case 256: {
bitField0_ |= 0x00000008;
osmosisReplicationTimestamp_ = input.readInt64();
break;
}
case 264: {
bitField0_ |= 0x00000010;
osmosisReplicationSequenceNumber_ = input.readInt64();
break;
}
case 274: {
java.lang.String s = input.readString();
bitField0_ |= 0x00000020;
osmosisReplicationBaseUrl_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.HeaderBlock.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.HeaderBlock)
private static final crosby.binary.Osmformat.HeaderBlock DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new HeaderBlock();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.HeaderBlock getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface HeaderBBoxOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.HeaderBBox)
com.google.protobuf.MessageLiteOrBuilder {
/**
* required sint64 left = 1;
*/
boolean hasLeft();
/**
* required sint64 left = 1;
*/
long getLeft();
/**
* required sint64 right = 2;
*/
boolean hasRight();
/**
* required sint64 right = 2;
*/
long getRight();
/**
* required sint64 top = 3;
*/
boolean hasTop();
/**
* required sint64 top = 3;
*/
long getTop();
/**
* required sint64 bottom = 4;
*/
boolean hasBottom();
/**
* required sint64 bottom = 4;
*/
long getBottom();
}
/**
* Protobuf type {@code OSMPBF.HeaderBBox}
*/
public static final class HeaderBBox extends
com.google.protobuf.GeneratedMessageLite<
HeaderBBox, HeaderBBox.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.HeaderBBox)
HeaderBBoxOrBuilder {
private HeaderBBox() {
}
private int bitField0_;
public static final int LEFT_FIELD_NUMBER = 1;
private long left_;
/**
* required sint64 left = 1;
*/
public boolean hasLeft() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required sint64 left = 1;
*/
public long getLeft() {
return left_;
}
/**
* required sint64 left = 1;
*/
private void setLeft(long value) {
bitField0_ |= 0x00000001;
left_ = value;
}
/**
* required sint64 left = 1;
*/
private void clearLeft() {
bitField0_ = (bitField0_ & ~0x00000001);
left_ = 0L;
}
public static final int RIGHT_FIELD_NUMBER = 2;
private long right_;
/**
* required sint64 right = 2;
*/
public boolean hasRight() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required sint64 right = 2;
*/
public long getRight() {
return right_;
}
/**
* required sint64 right = 2;
*/
private void setRight(long value) {
bitField0_ |= 0x00000002;
right_ = value;
}
/**
* required sint64 right = 2;
*/
private void clearRight() {
bitField0_ = (bitField0_ & ~0x00000002);
right_ = 0L;
}
public static final int TOP_FIELD_NUMBER = 3;
private long top_;
/**
* required sint64 top = 3;
*/
public boolean hasTop() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required sint64 top = 3;
*/
public long getTop() {
return top_;
}
/**
* required sint64 top = 3;
*/
private void setTop(long value) {
bitField0_ |= 0x00000004;
top_ = value;
}
/**
* required sint64 top = 3;
*/
private void clearTop() {
bitField0_ = (bitField0_ & ~0x00000004);
top_ = 0L;
}
public static final int BOTTOM_FIELD_NUMBER = 4;
private long bottom_;
/**
* required sint64 bottom = 4;
*/
public boolean hasBottom() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required sint64 bottom = 4;
*/
public long getBottom() {
return bottom_;
}
/**
* required sint64 bottom = 4;
*/
private void setBottom(long value) {
bitField0_ |= 0x00000008;
bottom_ = value;
}
/**
* required sint64 bottom = 4;
*/
private void clearBottom() {
bitField0_ = (bitField0_ & ~0x00000008);
bottom_ = 0L;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeSInt64(1, left_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeSInt64(2, right_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeSInt64(3, top_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeSInt64(4, bottom_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, left_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, right_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(3, top_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(4, bottom_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBBox parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.HeaderBBox parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.HeaderBBox parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.HeaderBBox prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.HeaderBBox}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.HeaderBBox, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.HeaderBBox)
crosby.binary.Osmformat.HeaderBBoxOrBuilder {
// Construct using crosby.binary.Osmformat.HeaderBBox.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* required sint64 left = 1;
*/
public boolean hasLeft() {
return instance.hasLeft();
}
/**
* required sint64 left = 1;
*/
public long getLeft() {
return instance.getLeft();
}
/**
* required sint64 left = 1;
*/
public Builder setLeft(long value) {
copyOnWrite();
instance.setLeft(value);
return this;
}
/**
* required sint64 left = 1;
*/
public Builder clearLeft() {
copyOnWrite();
instance.clearLeft();
return this;
}
/**
* required sint64 right = 2;
*/
public boolean hasRight() {
return instance.hasRight();
}
/**
* required sint64 right = 2;
*/
public long getRight() {
return instance.getRight();
}
/**
* required sint64 right = 2;
*/
public Builder setRight(long value) {
copyOnWrite();
instance.setRight(value);
return this;
}
/**
* required sint64 right = 2;
*/
public Builder clearRight() {
copyOnWrite();
instance.clearRight();
return this;
}
/**
* required sint64 top = 3;
*/
public boolean hasTop() {
return instance.hasTop();
}
/**
* required sint64 top = 3;
*/
public long getTop() {
return instance.getTop();
}
/**
* required sint64 top = 3;
*/
public Builder setTop(long value) {
copyOnWrite();
instance.setTop(value);
return this;
}
/**
* required sint64 top = 3;
*/
public Builder clearTop() {
copyOnWrite();
instance.clearTop();
return this;
}
/**
* required sint64 bottom = 4;
*/
public boolean hasBottom() {
return instance.hasBottom();
}
/**
* required sint64 bottom = 4;
*/
public long getBottom() {
return instance.getBottom();
}
/**
* required sint64 bottom = 4;
*/
public Builder setBottom(long value) {
copyOnWrite();
instance.setBottom(value);
return this;
}
/**
* required sint64 bottom = 4;
*/
public Builder clearBottom() {
copyOnWrite();
instance.clearBottom();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.HeaderBBox)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.HeaderBBox();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasLeft()) {
return null;
}
if (!hasRight()) {
return null;
}
if (!hasTop()) {
return null;
}
if (!hasBottom()) {
return null;
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.HeaderBBox other = (crosby.binary.Osmformat.HeaderBBox) arg1;
left_ = visitor.visitLong(
hasLeft(), left_,
other.hasLeft(), other.left_);
right_ = visitor.visitLong(
hasRight(), right_,
other.hasRight(), other.right_);
top_ = visitor.visitLong(
hasTop(), top_,
other.hasTop(), other.top_);
bottom_ = visitor.visitLong(
hasBottom(), bottom_,
other.hasBottom(), other.bottom_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
left_ = input.readSInt64();
break;
}
case 16: {
bitField0_ |= 0x00000002;
right_ = input.readSInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
top_ = input.readSInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
bottom_ = input.readSInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.HeaderBBox.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.HeaderBBox)
private static final crosby.binary.Osmformat.HeaderBBox DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new HeaderBBox();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.HeaderBBox getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface PrimitiveBlockOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.PrimitiveBlock)
com.google.protobuf.MessageLiteOrBuilder {
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
boolean hasStringtable();
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
crosby.binary.Osmformat.StringTable getStringtable();
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
java.util.List
getPrimitivegroupList();
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
crosby.binary.Osmformat.PrimitiveGroup getPrimitivegroup(int index);
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
int getPrimitivegroupCount();
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
boolean hasGranularity();
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
int getGranularity();
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
boolean hasLatOffset();
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
long getLatOffset();
/**
* optional int64 lon_offset = 20 [default = 0];
*/
boolean hasLonOffset();
/**
* optional int64 lon_offset = 20 [default = 0];
*/
long getLonOffset();
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
boolean hasDateGranularity();
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
int getDateGranularity();
}
/**
* Protobuf type {@code OSMPBF.PrimitiveBlock}
*/
public static final class PrimitiveBlock extends
com.google.protobuf.GeneratedMessageLite<
PrimitiveBlock, PrimitiveBlock.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.PrimitiveBlock)
PrimitiveBlockOrBuilder {
private PrimitiveBlock() {
primitivegroup_ = emptyProtobufList();
granularity_ = 100;
dateGranularity_ = 1000;
}
private int bitField0_;
public static final int STRINGTABLE_FIELD_NUMBER = 1;
private crosby.binary.Osmformat.StringTable stringtable_;
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public boolean hasStringtable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public crosby.binary.Osmformat.StringTable getStringtable() {
return stringtable_ == null ? crosby.binary.Osmformat.StringTable.getDefaultInstance() : stringtable_;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
private void setStringtable(crosby.binary.Osmformat.StringTable value) {
if (value == null) {
throw new NullPointerException();
}
stringtable_ = value;
bitField0_ |= 0x00000001;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
private void setStringtable(
crosby.binary.Osmformat.StringTable.Builder builderForValue) {
stringtable_ = builderForValue.build();
bitField0_ |= 0x00000001;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
private void mergeStringtable(crosby.binary.Osmformat.StringTable value) {
if (stringtable_ != null &&
stringtable_ != crosby.binary.Osmformat.StringTable.getDefaultInstance()) {
stringtable_ =
crosby.binary.Osmformat.StringTable.newBuilder(stringtable_).mergeFrom(value).buildPartial();
} else {
stringtable_ = value;
}
bitField0_ |= 0x00000001;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
private void clearStringtable() { stringtable_ = null;
bitField0_ = (bitField0_ & ~0x00000001);
}
public static final int PRIMITIVEGROUP_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.ProtobufList primitivegroup_;
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public java.util.List getPrimitivegroupList() {
return primitivegroup_;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public java.util.List extends crosby.binary.Osmformat.PrimitiveGroupOrBuilder>
getPrimitivegroupOrBuilderList() {
return primitivegroup_;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public int getPrimitivegroupCount() {
return primitivegroup_.size();
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public crosby.binary.Osmformat.PrimitiveGroup getPrimitivegroup(int index) {
return primitivegroup_.get(index);
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public crosby.binary.Osmformat.PrimitiveGroupOrBuilder getPrimitivegroupOrBuilder(
int index) {
return primitivegroup_.get(index);
}
private void ensurePrimitivegroupIsMutable() {
if (!primitivegroup_.isModifiable()) {
primitivegroup_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(primitivegroup_);
}
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void setPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrimitivegroupIsMutable();
primitivegroup_.set(index, value);
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void setPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup.Builder builderForValue) {
ensurePrimitivegroupIsMutable();
primitivegroup_.set(index, builderForValue.build());
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void addPrimitivegroup(crosby.binary.Osmformat.PrimitiveGroup value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrimitivegroupIsMutable();
primitivegroup_.add(value);
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void addPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup value) {
if (value == null) {
throw new NullPointerException();
}
ensurePrimitivegroupIsMutable();
primitivegroup_.add(index, value);
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void addPrimitivegroup(
crosby.binary.Osmformat.PrimitiveGroup.Builder builderForValue) {
ensurePrimitivegroupIsMutable();
primitivegroup_.add(builderForValue.build());
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void addPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup.Builder builderForValue) {
ensurePrimitivegroupIsMutable();
primitivegroup_.add(index, builderForValue.build());
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void addAllPrimitivegroup(
java.lang.Iterable extends crosby.binary.Osmformat.PrimitiveGroup> values) {
ensurePrimitivegroupIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, primitivegroup_);
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void clearPrimitivegroup() {
primitivegroup_ = emptyProtobufList();
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
private void removePrimitivegroup(int index) {
ensurePrimitivegroupIsMutable();
primitivegroup_.remove(index);
}
public static final int GRANULARITY_FIELD_NUMBER = 17;
private int granularity_;
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
public boolean hasGranularity() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
public int getGranularity() {
return granularity_;
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
private void setGranularity(int value) {
bitField0_ |= 0x00000002;
granularity_ = value;
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
private void clearGranularity() {
bitField0_ = (bitField0_ & ~0x00000002);
granularity_ = 100;
}
public static final int LAT_OFFSET_FIELD_NUMBER = 19;
private long latOffset_;
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
public boolean hasLatOffset() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
public long getLatOffset() {
return latOffset_;
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
private void setLatOffset(long value) {
bitField0_ |= 0x00000004;
latOffset_ = value;
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
private void clearLatOffset() {
bitField0_ = (bitField0_ & ~0x00000004);
latOffset_ = 0L;
}
public static final int LON_OFFSET_FIELD_NUMBER = 20;
private long lonOffset_;
/**
* optional int64 lon_offset = 20 [default = 0];
*/
public boolean hasLonOffset() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
public long getLonOffset() {
return lonOffset_;
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
private void setLonOffset(long value) {
bitField0_ |= 0x00000008;
lonOffset_ = value;
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
private void clearLonOffset() {
bitField0_ = (bitField0_ & ~0x00000008);
lonOffset_ = 0L;
}
public static final int DATE_GRANULARITY_FIELD_NUMBER = 18;
private int dateGranularity_;
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
public boolean hasDateGranularity() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
public int getDateGranularity() {
return dateGranularity_;
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
private void setDateGranularity(int value) {
bitField0_ |= 0x00000010;
dateGranularity_ = value;
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
private void clearDateGranularity() {
bitField0_ = (bitField0_ & ~0x00000010);
dateGranularity_ = 1000;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, getStringtable());
}
for (int i = 0; i < primitivegroup_.size(); i++) {
output.writeMessage(2, primitivegroup_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(17, granularity_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(18, dateGranularity_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(19, latOffset_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(20, lonOffset_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStringtable());
}
for (int i = 0; i < primitivegroup_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, primitivegroup_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(17, granularity_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(18, dateGranularity_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, latOffset_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(20, lonOffset_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.PrimitiveBlock parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.PrimitiveBlock prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.PrimitiveBlock}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.PrimitiveBlock, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.PrimitiveBlock)
crosby.binary.Osmformat.PrimitiveBlockOrBuilder {
// Construct using crosby.binary.Osmformat.PrimitiveBlock.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public boolean hasStringtable() {
return instance.hasStringtable();
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public crosby.binary.Osmformat.StringTable getStringtable() {
return instance.getStringtable();
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public Builder setStringtable(crosby.binary.Osmformat.StringTable value) {
copyOnWrite();
instance.setStringtable(value);
return this;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public Builder setStringtable(
crosby.binary.Osmformat.StringTable.Builder builderForValue) {
copyOnWrite();
instance.setStringtable(builderForValue);
return this;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public Builder mergeStringtable(crosby.binary.Osmformat.StringTable value) {
copyOnWrite();
instance.mergeStringtable(value);
return this;
}
/**
* required .OSMPBF.StringTable stringtable = 1;
*/
public Builder clearStringtable() { copyOnWrite();
instance.clearStringtable();
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public java.util.List getPrimitivegroupList() {
return java.util.Collections.unmodifiableList(
instance.getPrimitivegroupList());
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public int getPrimitivegroupCount() {
return instance.getPrimitivegroupCount();
}/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public crosby.binary.Osmformat.PrimitiveGroup getPrimitivegroup(int index) {
return instance.getPrimitivegroup(index);
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder setPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup value) {
copyOnWrite();
instance.setPrimitivegroup(index, value);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder setPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup.Builder builderForValue) {
copyOnWrite();
instance.setPrimitivegroup(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder addPrimitivegroup(crosby.binary.Osmformat.PrimitiveGroup value) {
copyOnWrite();
instance.addPrimitivegroup(value);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder addPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup value) {
copyOnWrite();
instance.addPrimitivegroup(index, value);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder addPrimitivegroup(
crosby.binary.Osmformat.PrimitiveGroup.Builder builderForValue) {
copyOnWrite();
instance.addPrimitivegroup(builderForValue);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder addPrimitivegroup(
int index, crosby.binary.Osmformat.PrimitiveGroup.Builder builderForValue) {
copyOnWrite();
instance.addPrimitivegroup(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder addAllPrimitivegroup(
java.lang.Iterable extends crosby.binary.Osmformat.PrimitiveGroup> values) {
copyOnWrite();
instance.addAllPrimitivegroup(values);
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder clearPrimitivegroup() {
copyOnWrite();
instance.clearPrimitivegroup();
return this;
}
/**
* repeated .OSMPBF.PrimitiveGroup primitivegroup = 2;
*/
public Builder removePrimitivegroup(int index) {
copyOnWrite();
instance.removePrimitivegroup(index);
return this;
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
public boolean hasGranularity() {
return instance.hasGranularity();
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
public int getGranularity() {
return instance.getGranularity();
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
public Builder setGranularity(int value) {
copyOnWrite();
instance.setGranularity(value);
return this;
}
/**
*
* Granularity, units of nanodegrees, used to store coordinates in this block
*
*
* optional int32 granularity = 17 [default = 100];
*/
public Builder clearGranularity() {
copyOnWrite();
instance.clearGranularity();
return this;
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
public boolean hasLatOffset() {
return instance.hasLatOffset();
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
public long getLatOffset() {
return instance.getLatOffset();
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
public Builder setLatOffset(long value) {
copyOnWrite();
instance.setLatOffset(value);
return this;
}
/**
*
* Offset value between the output coordinates coordinates and the granularity grid in unites of nanodegrees.
*
*
* optional int64 lat_offset = 19 [default = 0];
*/
public Builder clearLatOffset() {
copyOnWrite();
instance.clearLatOffset();
return this;
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
public boolean hasLonOffset() {
return instance.hasLonOffset();
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
public long getLonOffset() {
return instance.getLonOffset();
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
public Builder setLonOffset(long value) {
copyOnWrite();
instance.setLonOffset(value);
return this;
}
/**
* optional int64 lon_offset = 20 [default = 0];
*/
public Builder clearLonOffset() {
copyOnWrite();
instance.clearLonOffset();
return this;
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
public boolean hasDateGranularity() {
return instance.hasDateGranularity();
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
public int getDateGranularity() {
return instance.getDateGranularity();
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
public Builder setDateGranularity(int value) {
copyOnWrite();
instance.setDateGranularity(value);
return this;
}
/**
*
* Granularity of dates, normally represented in units of milliseconds since the 1970 epoch.
*
*
* optional int32 date_granularity = 18 [default = 1000];
*/
public Builder clearDateGranularity() {
copyOnWrite();
instance.clearDateGranularity();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.PrimitiveBlock)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.PrimitiveBlock();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasStringtable()) {
return null;
}
for (int i = 0; i < getPrimitivegroupCount(); i++) {
if (!getPrimitivegroup(i).isInitialized()) {
return null;
}
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
primitivegroup_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.PrimitiveBlock other = (crosby.binary.Osmformat.PrimitiveBlock) arg1;
stringtable_ = visitor.visitMessage(stringtable_, other.stringtable_);
primitivegroup_= visitor.visitList(primitivegroup_, other.primitivegroup_);
granularity_ = visitor.visitInt(
hasGranularity(), granularity_,
other.hasGranularity(), other.granularity_);
latOffset_ = visitor.visitLong(
hasLatOffset(), latOffset_,
other.hasLatOffset(), other.latOffset_);
lonOffset_ = visitor.visitLong(
hasLonOffset(), lonOffset_,
other.hasLonOffset(), other.lonOffset_);
dateGranularity_ = visitor.visitInt(
hasDateGranularity(), dateGranularity_,
other.hasDateGranularity(), other.dateGranularity_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 10: {
crosby.binary.Osmformat.StringTable.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = stringtable_.toBuilder();
}
stringtable_ = input.readMessage(crosby.binary.Osmformat.StringTable.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stringtable_);
stringtable_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
if (!primitivegroup_.isModifiable()) {
primitivegroup_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(primitivegroup_);
}
primitivegroup_.add(
input.readMessage(crosby.binary.Osmformat.PrimitiveGroup.parser(), extensionRegistry));
break;
}
case 136: {
bitField0_ |= 0x00000002;
granularity_ = input.readInt32();
break;
}
case 144: {
bitField0_ |= 0x00000010;
dateGranularity_ = input.readInt32();
break;
}
case 152: {
bitField0_ |= 0x00000004;
latOffset_ = input.readInt64();
break;
}
case 160: {
bitField0_ |= 0x00000008;
lonOffset_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.PrimitiveBlock.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.PrimitiveBlock)
private static final crosby.binary.Osmformat.PrimitiveBlock DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PrimitiveBlock();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.PrimitiveBlock getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface PrimitiveGroupOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.PrimitiveGroup)
com.google.protobuf.MessageLiteOrBuilder {
/**
* repeated .OSMPBF.Node nodes = 1;
*/
java.util.List
getNodesList();
/**
* repeated .OSMPBF.Node nodes = 1;
*/
crosby.binary.Osmformat.Node getNodes(int index);
/**
* repeated .OSMPBF.Node nodes = 1;
*/
int getNodesCount();
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
boolean hasDense();
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
crosby.binary.Osmformat.DenseNodes getDense();
/**
* repeated .OSMPBF.Way ways = 3;
*/
java.util.List
getWaysList();
/**
* repeated .OSMPBF.Way ways = 3;
*/
crosby.binary.Osmformat.Way getWays(int index);
/**
* repeated .OSMPBF.Way ways = 3;
*/
int getWaysCount();
/**
* repeated .OSMPBF.Relation relations = 4;
*/
java.util.List
getRelationsList();
/**
* repeated .OSMPBF.Relation relations = 4;
*/
crosby.binary.Osmformat.Relation getRelations(int index);
/**
* repeated .OSMPBF.Relation relations = 4;
*/
int getRelationsCount();
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
java.util.List
getChangesetsList();
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
crosby.binary.Osmformat.ChangeSet getChangesets(int index);
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
int getChangesetsCount();
}
/**
*
* Group of OSMPrimitives. All primitives in a group must be the same type.
*
*
* Protobuf type {@code OSMPBF.PrimitiveGroup}
*/
public static final class PrimitiveGroup extends
com.google.protobuf.GeneratedMessageLite<
PrimitiveGroup, PrimitiveGroup.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.PrimitiveGroup)
PrimitiveGroupOrBuilder {
private PrimitiveGroup() {
nodes_ = emptyProtobufList();
ways_ = emptyProtobufList();
relations_ = emptyProtobufList();
changesets_ = emptyProtobufList();
}
private int bitField0_;
public static final int NODES_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.ProtobufList nodes_;
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public java.util.List getNodesList() {
return nodes_;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public java.util.List extends crosby.binary.Osmformat.NodeOrBuilder>
getNodesOrBuilderList() {
return nodes_;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public int getNodesCount() {
return nodes_.size();
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public crosby.binary.Osmformat.Node getNodes(int index) {
return nodes_.get(index);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public crosby.binary.Osmformat.NodeOrBuilder getNodesOrBuilder(
int index) {
return nodes_.get(index);
}
private void ensureNodesIsMutable() {
if (!nodes_.isModifiable()) {
nodes_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(nodes_);
}
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void setNodes(
int index, crosby.binary.Osmformat.Node value) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.set(index, value);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void setNodes(
int index, crosby.binary.Osmformat.Node.Builder builderForValue) {
ensureNodesIsMutable();
nodes_.set(index, builderForValue.build());
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void addNodes(crosby.binary.Osmformat.Node value) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.add(value);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void addNodes(
int index, crosby.binary.Osmformat.Node value) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.add(index, value);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void addNodes(
crosby.binary.Osmformat.Node.Builder builderForValue) {
ensureNodesIsMutable();
nodes_.add(builderForValue.build());
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void addNodes(
int index, crosby.binary.Osmformat.Node.Builder builderForValue) {
ensureNodesIsMutable();
nodes_.add(index, builderForValue.build());
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void addAllNodes(
java.lang.Iterable extends crosby.binary.Osmformat.Node> values) {
ensureNodesIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, nodes_);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void clearNodes() {
nodes_ = emptyProtobufList();
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
private void removeNodes(int index) {
ensureNodesIsMutable();
nodes_.remove(index);
}
public static final int DENSE_FIELD_NUMBER = 2;
private crosby.binary.Osmformat.DenseNodes dense_;
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public boolean hasDense() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public crosby.binary.Osmformat.DenseNodes getDense() {
return dense_ == null ? crosby.binary.Osmformat.DenseNodes.getDefaultInstance() : dense_;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
private void setDense(crosby.binary.Osmformat.DenseNodes value) {
if (value == null) {
throw new NullPointerException();
}
dense_ = value;
bitField0_ |= 0x00000001;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
private void setDense(
crosby.binary.Osmformat.DenseNodes.Builder builderForValue) {
dense_ = builderForValue.build();
bitField0_ |= 0x00000001;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
private void mergeDense(crosby.binary.Osmformat.DenseNodes value) {
if (dense_ != null &&
dense_ != crosby.binary.Osmformat.DenseNodes.getDefaultInstance()) {
dense_ =
crosby.binary.Osmformat.DenseNodes.newBuilder(dense_).mergeFrom(value).buildPartial();
} else {
dense_ = value;
}
bitField0_ |= 0x00000001;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
private void clearDense() { dense_ = null;
bitField0_ = (bitField0_ & ~0x00000001);
}
public static final int WAYS_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.ProtobufList ways_;
/**
* repeated .OSMPBF.Way ways = 3;
*/
public java.util.List getWaysList() {
return ways_;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public java.util.List extends crosby.binary.Osmformat.WayOrBuilder>
getWaysOrBuilderList() {
return ways_;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public int getWaysCount() {
return ways_.size();
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public crosby.binary.Osmformat.Way getWays(int index) {
return ways_.get(index);
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public crosby.binary.Osmformat.WayOrBuilder getWaysOrBuilder(
int index) {
return ways_.get(index);
}
private void ensureWaysIsMutable() {
if (!ways_.isModifiable()) {
ways_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(ways_);
}
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void setWays(
int index, crosby.binary.Osmformat.Way value) {
if (value == null) {
throw new NullPointerException();
}
ensureWaysIsMutable();
ways_.set(index, value);
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void setWays(
int index, crosby.binary.Osmformat.Way.Builder builderForValue) {
ensureWaysIsMutable();
ways_.set(index, builderForValue.build());
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void addWays(crosby.binary.Osmformat.Way value) {
if (value == null) {
throw new NullPointerException();
}
ensureWaysIsMutable();
ways_.add(value);
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void addWays(
int index, crosby.binary.Osmformat.Way value) {
if (value == null) {
throw new NullPointerException();
}
ensureWaysIsMutable();
ways_.add(index, value);
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void addWays(
crosby.binary.Osmformat.Way.Builder builderForValue) {
ensureWaysIsMutable();
ways_.add(builderForValue.build());
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void addWays(
int index, crosby.binary.Osmformat.Way.Builder builderForValue) {
ensureWaysIsMutable();
ways_.add(index, builderForValue.build());
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void addAllWays(
java.lang.Iterable extends crosby.binary.Osmformat.Way> values) {
ensureWaysIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, ways_);
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void clearWays() {
ways_ = emptyProtobufList();
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
private void removeWays(int index) {
ensureWaysIsMutable();
ways_.remove(index);
}
public static final int RELATIONS_FIELD_NUMBER = 4;
private com.google.protobuf.Internal.ProtobufList relations_;
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public java.util.List getRelationsList() {
return relations_;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public java.util.List extends crosby.binary.Osmformat.RelationOrBuilder>
getRelationsOrBuilderList() {
return relations_;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public int getRelationsCount() {
return relations_.size();
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public crosby.binary.Osmformat.Relation getRelations(int index) {
return relations_.get(index);
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public crosby.binary.Osmformat.RelationOrBuilder getRelationsOrBuilder(
int index) {
return relations_.get(index);
}
private void ensureRelationsIsMutable() {
if (!relations_.isModifiable()) {
relations_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(relations_);
}
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void setRelations(
int index, crosby.binary.Osmformat.Relation value) {
if (value == null) {
throw new NullPointerException();
}
ensureRelationsIsMutable();
relations_.set(index, value);
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void setRelations(
int index, crosby.binary.Osmformat.Relation.Builder builderForValue) {
ensureRelationsIsMutable();
relations_.set(index, builderForValue.build());
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void addRelations(crosby.binary.Osmformat.Relation value) {
if (value == null) {
throw new NullPointerException();
}
ensureRelationsIsMutable();
relations_.add(value);
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void addRelations(
int index, crosby.binary.Osmformat.Relation value) {
if (value == null) {
throw new NullPointerException();
}
ensureRelationsIsMutable();
relations_.add(index, value);
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void addRelations(
crosby.binary.Osmformat.Relation.Builder builderForValue) {
ensureRelationsIsMutable();
relations_.add(builderForValue.build());
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void addRelations(
int index, crosby.binary.Osmformat.Relation.Builder builderForValue) {
ensureRelationsIsMutable();
relations_.add(index, builderForValue.build());
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void addAllRelations(
java.lang.Iterable extends crosby.binary.Osmformat.Relation> values) {
ensureRelationsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, relations_);
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void clearRelations() {
relations_ = emptyProtobufList();
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
private void removeRelations(int index) {
ensureRelationsIsMutable();
relations_.remove(index);
}
public static final int CHANGESETS_FIELD_NUMBER = 5;
private com.google.protobuf.Internal.ProtobufList changesets_;
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public java.util.List getChangesetsList() {
return changesets_;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public java.util.List extends crosby.binary.Osmformat.ChangeSetOrBuilder>
getChangesetsOrBuilderList() {
return changesets_;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public int getChangesetsCount() {
return changesets_.size();
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public crosby.binary.Osmformat.ChangeSet getChangesets(int index) {
return changesets_.get(index);
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public crosby.binary.Osmformat.ChangeSetOrBuilder getChangesetsOrBuilder(
int index) {
return changesets_.get(index);
}
private void ensureChangesetsIsMutable() {
if (!changesets_.isModifiable()) {
changesets_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(changesets_);
}
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void setChangesets(
int index, crosby.binary.Osmformat.ChangeSet value) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesetsIsMutable();
changesets_.set(index, value);
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void setChangesets(
int index, crosby.binary.Osmformat.ChangeSet.Builder builderForValue) {
ensureChangesetsIsMutable();
changesets_.set(index, builderForValue.build());
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void addChangesets(crosby.binary.Osmformat.ChangeSet value) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesetsIsMutable();
changesets_.add(value);
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void addChangesets(
int index, crosby.binary.Osmformat.ChangeSet value) {
if (value == null) {
throw new NullPointerException();
}
ensureChangesetsIsMutable();
changesets_.add(index, value);
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void addChangesets(
crosby.binary.Osmformat.ChangeSet.Builder builderForValue) {
ensureChangesetsIsMutable();
changesets_.add(builderForValue.build());
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void addChangesets(
int index, crosby.binary.Osmformat.ChangeSet.Builder builderForValue) {
ensureChangesetsIsMutable();
changesets_.add(index, builderForValue.build());
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void addAllChangesets(
java.lang.Iterable extends crosby.binary.Osmformat.ChangeSet> values) {
ensureChangesetsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, changesets_);
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void clearChangesets() {
changesets_ = emptyProtobufList();
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
private void removeChangesets(int index) {
ensureChangesetsIsMutable();
changesets_.remove(index);
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nodes_.size(); i++) {
output.writeMessage(1, nodes_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(2, getDense());
}
for (int i = 0; i < ways_.size(); i++) {
output.writeMessage(3, ways_.get(i));
}
for (int i = 0; i < relations_.size(); i++) {
output.writeMessage(4, relations_.get(i));
}
for (int i = 0; i < changesets_.size(); i++) {
output.writeMessage(5, changesets_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nodes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nodes_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getDense());
}
for (int i = 0; i < ways_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, ways_.get(i));
}
for (int i = 0; i < relations_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, relations_.get(i));
}
for (int i = 0; i < changesets_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, changesets_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.PrimitiveGroup parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.PrimitiveGroup prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
* Group of OSMPrimitives. All primitives in a group must be the same type.
*
*
* Protobuf type {@code OSMPBF.PrimitiveGroup}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.PrimitiveGroup, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.PrimitiveGroup)
crosby.binary.Osmformat.PrimitiveGroupOrBuilder {
// Construct using crosby.binary.Osmformat.PrimitiveGroup.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public java.util.List getNodesList() {
return java.util.Collections.unmodifiableList(
instance.getNodesList());
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public int getNodesCount() {
return instance.getNodesCount();
}/**
* repeated .OSMPBF.Node nodes = 1;
*/
public crosby.binary.Osmformat.Node getNodes(int index) {
return instance.getNodes(index);
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder setNodes(
int index, crosby.binary.Osmformat.Node value) {
copyOnWrite();
instance.setNodes(index, value);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder setNodes(
int index, crosby.binary.Osmformat.Node.Builder builderForValue) {
copyOnWrite();
instance.setNodes(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder addNodes(crosby.binary.Osmformat.Node value) {
copyOnWrite();
instance.addNodes(value);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder addNodes(
int index, crosby.binary.Osmformat.Node value) {
copyOnWrite();
instance.addNodes(index, value);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder addNodes(
crosby.binary.Osmformat.Node.Builder builderForValue) {
copyOnWrite();
instance.addNodes(builderForValue);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder addNodes(
int index, crosby.binary.Osmformat.Node.Builder builderForValue) {
copyOnWrite();
instance.addNodes(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder addAllNodes(
java.lang.Iterable extends crosby.binary.Osmformat.Node> values) {
copyOnWrite();
instance.addAllNodes(values);
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder clearNodes() {
copyOnWrite();
instance.clearNodes();
return this;
}
/**
* repeated .OSMPBF.Node nodes = 1;
*/
public Builder removeNodes(int index) {
copyOnWrite();
instance.removeNodes(index);
return this;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public boolean hasDense() {
return instance.hasDense();
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public crosby.binary.Osmformat.DenseNodes getDense() {
return instance.getDense();
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public Builder setDense(crosby.binary.Osmformat.DenseNodes value) {
copyOnWrite();
instance.setDense(value);
return this;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public Builder setDense(
crosby.binary.Osmformat.DenseNodes.Builder builderForValue) {
copyOnWrite();
instance.setDense(builderForValue);
return this;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public Builder mergeDense(crosby.binary.Osmformat.DenseNodes value) {
copyOnWrite();
instance.mergeDense(value);
return this;
}
/**
* optional .OSMPBF.DenseNodes dense = 2;
*/
public Builder clearDense() { copyOnWrite();
instance.clearDense();
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public java.util.List getWaysList() {
return java.util.Collections.unmodifiableList(
instance.getWaysList());
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public int getWaysCount() {
return instance.getWaysCount();
}/**
* repeated .OSMPBF.Way ways = 3;
*/
public crosby.binary.Osmformat.Way getWays(int index) {
return instance.getWays(index);
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder setWays(
int index, crosby.binary.Osmformat.Way value) {
copyOnWrite();
instance.setWays(index, value);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder setWays(
int index, crosby.binary.Osmformat.Way.Builder builderForValue) {
copyOnWrite();
instance.setWays(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder addWays(crosby.binary.Osmformat.Way value) {
copyOnWrite();
instance.addWays(value);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder addWays(
int index, crosby.binary.Osmformat.Way value) {
copyOnWrite();
instance.addWays(index, value);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder addWays(
crosby.binary.Osmformat.Way.Builder builderForValue) {
copyOnWrite();
instance.addWays(builderForValue);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder addWays(
int index, crosby.binary.Osmformat.Way.Builder builderForValue) {
copyOnWrite();
instance.addWays(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder addAllWays(
java.lang.Iterable extends crosby.binary.Osmformat.Way> values) {
copyOnWrite();
instance.addAllWays(values);
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder clearWays() {
copyOnWrite();
instance.clearWays();
return this;
}
/**
* repeated .OSMPBF.Way ways = 3;
*/
public Builder removeWays(int index) {
copyOnWrite();
instance.removeWays(index);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public java.util.List getRelationsList() {
return java.util.Collections.unmodifiableList(
instance.getRelationsList());
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public int getRelationsCount() {
return instance.getRelationsCount();
}/**
* repeated .OSMPBF.Relation relations = 4;
*/
public crosby.binary.Osmformat.Relation getRelations(int index) {
return instance.getRelations(index);
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder setRelations(
int index, crosby.binary.Osmformat.Relation value) {
copyOnWrite();
instance.setRelations(index, value);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder setRelations(
int index, crosby.binary.Osmformat.Relation.Builder builderForValue) {
copyOnWrite();
instance.setRelations(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder addRelations(crosby.binary.Osmformat.Relation value) {
copyOnWrite();
instance.addRelations(value);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder addRelations(
int index, crosby.binary.Osmformat.Relation value) {
copyOnWrite();
instance.addRelations(index, value);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder addRelations(
crosby.binary.Osmformat.Relation.Builder builderForValue) {
copyOnWrite();
instance.addRelations(builderForValue);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder addRelations(
int index, crosby.binary.Osmformat.Relation.Builder builderForValue) {
copyOnWrite();
instance.addRelations(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder addAllRelations(
java.lang.Iterable extends crosby.binary.Osmformat.Relation> values) {
copyOnWrite();
instance.addAllRelations(values);
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder clearRelations() {
copyOnWrite();
instance.clearRelations();
return this;
}
/**
* repeated .OSMPBF.Relation relations = 4;
*/
public Builder removeRelations(int index) {
copyOnWrite();
instance.removeRelations(index);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public java.util.List getChangesetsList() {
return java.util.Collections.unmodifiableList(
instance.getChangesetsList());
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public int getChangesetsCount() {
return instance.getChangesetsCount();
}/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public crosby.binary.Osmformat.ChangeSet getChangesets(int index) {
return instance.getChangesets(index);
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder setChangesets(
int index, crosby.binary.Osmformat.ChangeSet value) {
copyOnWrite();
instance.setChangesets(index, value);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder setChangesets(
int index, crosby.binary.Osmformat.ChangeSet.Builder builderForValue) {
copyOnWrite();
instance.setChangesets(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder addChangesets(crosby.binary.Osmformat.ChangeSet value) {
copyOnWrite();
instance.addChangesets(value);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder addChangesets(
int index, crosby.binary.Osmformat.ChangeSet value) {
copyOnWrite();
instance.addChangesets(index, value);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder addChangesets(
crosby.binary.Osmformat.ChangeSet.Builder builderForValue) {
copyOnWrite();
instance.addChangesets(builderForValue);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder addChangesets(
int index, crosby.binary.Osmformat.ChangeSet.Builder builderForValue) {
copyOnWrite();
instance.addChangesets(index, builderForValue);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder addAllChangesets(
java.lang.Iterable extends crosby.binary.Osmformat.ChangeSet> values) {
copyOnWrite();
instance.addAllChangesets(values);
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder clearChangesets() {
copyOnWrite();
instance.clearChangesets();
return this;
}
/**
* repeated .OSMPBF.ChangeSet changesets = 5;
*/
public Builder removeChangesets(int index) {
copyOnWrite();
instance.removeChangesets(index);
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.PrimitiveGroup)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.PrimitiveGroup();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
for (int i = 0; i < getNodesCount(); i++) {
if (!getNodes(i).isInitialized()) {
return null;
}
}
for (int i = 0; i < getWaysCount(); i++) {
if (!getWays(i).isInitialized()) {
return null;
}
}
for (int i = 0; i < getRelationsCount(); i++) {
if (!getRelations(i).isInitialized()) {
return null;
}
}
for (int i = 0; i < getChangesetsCount(); i++) {
if (!getChangesets(i).isInitialized()) {
return null;
}
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
nodes_.makeImmutable();
ways_.makeImmutable();
relations_.makeImmutable();
changesets_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.PrimitiveGroup other = (crosby.binary.Osmformat.PrimitiveGroup) arg1;
nodes_= visitor.visitList(nodes_, other.nodes_);
dense_ = visitor.visitMessage(dense_, other.dense_);
ways_= visitor.visitList(ways_, other.ways_);
relations_= visitor.visitList(relations_, other.relations_);
changesets_= visitor.visitList(changesets_, other.changesets_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 10: {
if (!nodes_.isModifiable()) {
nodes_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(nodes_);
}
nodes_.add(
input.readMessage(crosby.binary.Osmformat.Node.parser(), extensionRegistry));
break;
}
case 18: {
crosby.binary.Osmformat.DenseNodes.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = dense_.toBuilder();
}
dense_ = input.readMessage(crosby.binary.Osmformat.DenseNodes.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(dense_);
dense_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 26: {
if (!ways_.isModifiable()) {
ways_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(ways_);
}
ways_.add(
input.readMessage(crosby.binary.Osmformat.Way.parser(), extensionRegistry));
break;
}
case 34: {
if (!relations_.isModifiable()) {
relations_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(relations_);
}
relations_.add(
input.readMessage(crosby.binary.Osmformat.Relation.parser(), extensionRegistry));
break;
}
case 42: {
if (!changesets_.isModifiable()) {
changesets_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(changesets_);
}
changesets_.add(
input.readMessage(crosby.binary.Osmformat.ChangeSet.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.PrimitiveGroup.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.PrimitiveGroup)
private static final crosby.binary.Osmformat.PrimitiveGroup DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new PrimitiveGroup();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.PrimitiveGroup getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface StringTableOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.StringTable)
com.google.protobuf.MessageLiteOrBuilder {
/**
* repeated bytes s = 1;
*/
java.util.List getSList();
/**
* repeated bytes s = 1;
*/
int getSCount();
/**
* repeated bytes s = 1;
*/
com.google.protobuf.ByteString getS(int index);
}
/**
*
** String table, contains the common strings in each block.
*Note that we reserve index '0' as a delimiter, so the entry at that
*index in the table is ALWAYS blank and unused.
*
*
* Protobuf type {@code OSMPBF.StringTable}
*/
public static final class StringTable extends
com.google.protobuf.GeneratedMessageLite<
StringTable, StringTable.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.StringTable)
StringTableOrBuilder {
private StringTable() {
s_ = emptyProtobufList();
}
public static final int S_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.ProtobufList s_;
/**
* repeated bytes s = 1;
*/
public java.util.List
getSList() {
return s_;
}
/**
* repeated bytes s = 1;
*/
public int getSCount() {
return s_.size();
}
/**
* repeated bytes s = 1;
*/
public com.google.protobuf.ByteString getS(int index) {
return s_.get(index);
}
private void ensureSIsMutable() {
if (!s_.isModifiable()) {
s_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(s_);
}
}
/**
* repeated bytes s = 1;
*/
private void setS(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSIsMutable();
s_.set(index, value);
}
/**
* repeated bytes s = 1;
*/
private void addS(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSIsMutable();
s_.add(value);
}
/**
* repeated bytes s = 1;
*/
private void addAllS(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureSIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, s_);
}
/**
* repeated bytes s = 1;
*/
private void clearS() {
s_ = emptyProtobufList();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < s_.size(); i++) {
output.writeBytes(1, s_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < s_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(s_.get(i));
}
size += dataSize;
size += 1 * getSList().size();
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.StringTable parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.StringTable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.StringTable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.StringTable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.StringTable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.StringTable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.StringTable prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
** String table, contains the common strings in each block.
*Note that we reserve index '0' as a delimiter, so the entry at that
*index in the table is ALWAYS blank and unused.
*
*
* Protobuf type {@code OSMPBF.StringTable}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.StringTable, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.StringTable)
crosby.binary.Osmformat.StringTableOrBuilder {
// Construct using crosby.binary.Osmformat.StringTable.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* repeated bytes s = 1;
*/
public java.util.List
getSList() {
return java.util.Collections.unmodifiableList(
instance.getSList());
}
/**
* repeated bytes s = 1;
*/
public int getSCount() {
return instance.getSCount();
}
/**
* repeated bytes s = 1;
*/
public com.google.protobuf.ByteString getS(int index) {
return instance.getS(index);
}
/**
* repeated bytes s = 1;
*/
public Builder setS(
int index, com.google.protobuf.ByteString value) {
copyOnWrite();
instance.setS(index, value);
return this;
}
/**
* repeated bytes s = 1;
*/
public Builder addS(com.google.protobuf.ByteString value) {
copyOnWrite();
instance.addS(value);
return this;
}
/**
* repeated bytes s = 1;
*/
public Builder addAllS(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
copyOnWrite();
instance.addAllS(values);
return this;
}
/**
* repeated bytes s = 1;
*/
public Builder clearS() {
copyOnWrite();
instance.clearS();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.StringTable)
}
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.StringTable();
}
case IS_INITIALIZED: {
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
s_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.StringTable other = (crosby.binary.Osmformat.StringTable) arg1;
s_= visitor.visitList(s_, other.s_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 10: {
if (!s_.isModifiable()) {
s_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(s_);
}
s_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.StringTable.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.StringTable)
private static final crosby.binary.Osmformat.StringTable DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new StringTable();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.StringTable getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface InfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.Info)
com.google.protobuf.MessageLiteOrBuilder {
/**
* optional int32 version = 1 [default = -1];
*/
boolean hasVersion();
/**
* optional int32 version = 1 [default = -1];
*/
int getVersion();
/**
* optional int64 timestamp = 2;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 2;
*/
long getTimestamp();
/**
* optional int64 changeset = 3;
*/
boolean hasChangeset();
/**
* optional int64 changeset = 3;
*/
long getChangeset();
/**
* optional int32 uid = 4;
*/
boolean hasUid();
/**
* optional int32 uid = 4;
*/
int getUid();
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
boolean hasUserSid();
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
int getUserSid();
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
boolean hasVisible();
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
boolean getVisible();
}
/**
*
* Optional metadata that may be included into each primitive.
*
*
* Protobuf type {@code OSMPBF.Info}
*/
public static final class Info extends
com.google.protobuf.GeneratedMessageLite<
Info, Info.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.Info)
InfoOrBuilder {
private Info() {
version_ = -1;
}
private int bitField0_;
public static final int VERSION_FIELD_NUMBER = 1;
private int version_;
/**
* optional int32 version = 1 [default = -1];
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 version = 1 [default = -1];
*/
public int getVersion() {
return version_;
}
/**
* optional int32 version = 1 [default = -1];
*/
private void setVersion(int value) {
bitField0_ |= 0x00000001;
version_ = value;
}
/**
* optional int32 version = 1 [default = -1];
*/
private void clearVersion() {
bitField0_ = (bitField0_ & ~0x00000001);
version_ = -1;
}
public static final int TIMESTAMP_FIELD_NUMBER = 2;
private long timestamp_;
/**
* optional int64 timestamp = 2;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 timestamp = 2;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 2;
*/
private void setTimestamp(long value) {
bitField0_ |= 0x00000002;
timestamp_ = value;
}
/**
* optional int64 timestamp = 2;
*/
private void clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000002);
timestamp_ = 0L;
}
public static final int CHANGESET_FIELD_NUMBER = 3;
private long changeset_;
/**
* optional int64 changeset = 3;
*/
public boolean hasChangeset() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 changeset = 3;
*/
public long getChangeset() {
return changeset_;
}
/**
* optional int64 changeset = 3;
*/
private void setChangeset(long value) {
bitField0_ |= 0x00000004;
changeset_ = value;
}
/**
* optional int64 changeset = 3;
*/
private void clearChangeset() {
bitField0_ = (bitField0_ & ~0x00000004);
changeset_ = 0L;
}
public static final int UID_FIELD_NUMBER = 4;
private int uid_;
/**
* optional int32 uid = 4;
*/
public boolean hasUid() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int32 uid = 4;
*/
public int getUid() {
return uid_;
}
/**
* optional int32 uid = 4;
*/
private void setUid(int value) {
bitField0_ |= 0x00000008;
uid_ = value;
}
/**
* optional int32 uid = 4;
*/
private void clearUid() {
bitField0_ = (bitField0_ & ~0x00000008);
uid_ = 0;
}
public static final int USER_SID_FIELD_NUMBER = 5;
private int userSid_;
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
public boolean hasUserSid() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
public int getUserSid() {
return userSid_;
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
private void setUserSid(int value) {
bitField0_ |= 0x00000010;
userSid_ = value;
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
private void clearUserSid() {
bitField0_ = (bitField0_ & ~0x00000010);
userSid_ = 0;
}
public static final int VISIBLE_FIELD_NUMBER = 6;
private boolean visible_;
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
public boolean hasVisible() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
public boolean getVisible() {
return visible_;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
private void setVisible(boolean value) {
bitField0_ |= 0x00000020;
visible_ = value;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
private void clearVisible() {
bitField0_ = (bitField0_ & ~0x00000020);
visible_ = false;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, version_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, timestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, changeset_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt32(4, uid_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeUInt32(5, userSid_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(6, visible_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, version_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, timestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, changeset_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, uid_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, userSid_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, visible_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.Info parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Info parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Info parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Info parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Info parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Info parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Info parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Info parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Info parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Info parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Info parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Info parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.Info prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
* Optional metadata that may be included into each primitive.
*
*
* Protobuf type {@code OSMPBF.Info}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.Info, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.Info)
crosby.binary.Osmformat.InfoOrBuilder {
// Construct using crosby.binary.Osmformat.Info.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* optional int32 version = 1 [default = -1];
*/
public boolean hasVersion() {
return instance.hasVersion();
}
/**
* optional int32 version = 1 [default = -1];
*/
public int getVersion() {
return instance.getVersion();
}
/**
* optional int32 version = 1 [default = -1];
*/
public Builder setVersion(int value) {
copyOnWrite();
instance.setVersion(value);
return this;
}
/**
* optional int32 version = 1 [default = -1];
*/
public Builder clearVersion() {
copyOnWrite();
instance.clearVersion();
return this;
}
/**
* optional int64 timestamp = 2;
*/
public boolean hasTimestamp() {
return instance.hasTimestamp();
}
/**
* optional int64 timestamp = 2;
*/
public long getTimestamp() {
return instance.getTimestamp();
}
/**
* optional int64 timestamp = 2;
*/
public Builder setTimestamp(long value) {
copyOnWrite();
instance.setTimestamp(value);
return this;
}
/**
* optional int64 timestamp = 2;
*/
public Builder clearTimestamp() {
copyOnWrite();
instance.clearTimestamp();
return this;
}
/**
* optional int64 changeset = 3;
*/
public boolean hasChangeset() {
return instance.hasChangeset();
}
/**
* optional int64 changeset = 3;
*/
public long getChangeset() {
return instance.getChangeset();
}
/**
* optional int64 changeset = 3;
*/
public Builder setChangeset(long value) {
copyOnWrite();
instance.setChangeset(value);
return this;
}
/**
* optional int64 changeset = 3;
*/
public Builder clearChangeset() {
copyOnWrite();
instance.clearChangeset();
return this;
}
/**
* optional int32 uid = 4;
*/
public boolean hasUid() {
return instance.hasUid();
}
/**
* optional int32 uid = 4;
*/
public int getUid() {
return instance.getUid();
}
/**
* optional int32 uid = 4;
*/
public Builder setUid(int value) {
copyOnWrite();
instance.setUid(value);
return this;
}
/**
* optional int32 uid = 4;
*/
public Builder clearUid() {
copyOnWrite();
instance.clearUid();
return this;
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
public boolean hasUserSid() {
return instance.hasUserSid();
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
public int getUserSid() {
return instance.getUserSid();
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
public Builder setUserSid(int value) {
copyOnWrite();
instance.setUserSid(value);
return this;
}
/**
*
* String IDs
*
*
* optional uint32 user_sid = 5;
*/
public Builder clearUserSid() {
copyOnWrite();
instance.clearUserSid();
return this;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
public boolean hasVisible() {
return instance.hasVisible();
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
public boolean getVisible() {
return instance.getVisible();
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
public Builder setVisible(boolean value) {
copyOnWrite();
instance.setVisible(value);
return this;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* optional bool visible = 6;
*/
public Builder clearVisible() {
copyOnWrite();
instance.clearVisible();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.Info)
}
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.Info();
}
case IS_INITIALIZED: {
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.Info other = (crosby.binary.Osmformat.Info) arg1;
version_ = visitor.visitInt(
hasVersion(), version_,
other.hasVersion(), other.version_);
timestamp_ = visitor.visitLong(
hasTimestamp(), timestamp_,
other.hasTimestamp(), other.timestamp_);
changeset_ = visitor.visitLong(
hasChangeset(), changeset_,
other.hasChangeset(), other.changeset_);
uid_ = visitor.visitInt(
hasUid(), uid_,
other.hasUid(), other.uid_);
userSid_ = visitor.visitInt(
hasUserSid(), userSid_,
other.hasUserSid(), other.userSid_);
visible_ = visitor.visitBoolean(
hasVisible(), visible_,
other.hasVisible(), other.visible_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
version_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
timestamp_ = input.readInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
changeset_ = input.readInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
uid_ = input.readInt32();
break;
}
case 40: {
bitField0_ |= 0x00000010;
userSid_ = input.readUInt32();
break;
}
case 48: {
bitField0_ |= 0x00000020;
visible_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.Info.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.Info)
private static final crosby.binary.Osmformat.Info DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Info();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.Info getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface DenseInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.DenseInfo)
com.google.protobuf.MessageLiteOrBuilder {
/**
* repeated int32 version = 1 [packed = true];
*/
java.util.List getVersionList();
/**
* repeated int32 version = 1 [packed = true];
*/
int getVersionCount();
/**
* repeated int32 version = 1 [packed = true];
*/
int getVersion(int index);
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
java.util.List getTimestampList();
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
int getTimestampCount();
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
long getTimestamp(int index);
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
java.util.List getChangesetList();
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
int getChangesetCount();
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
long getChangeset(int index);
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
java.util.List getUidList();
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
int getUidCount();
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
int getUid(int index);
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
java.util.List getUserSidList();
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
int getUserSidCount();
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
int getUserSid(int index);
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
java.util.List getVisibleList();
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
int getVisibleCount();
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
boolean getVisible(int index);
}
/**
*
** Optional metadata that may be included into each primitive. Special dense format used in DenseNodes.
*
*
* Protobuf type {@code OSMPBF.DenseInfo}
*/
public static final class DenseInfo extends
com.google.protobuf.GeneratedMessageLite<
DenseInfo, DenseInfo.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.DenseInfo)
DenseInfoOrBuilder {
private DenseInfo() {
version_ = emptyIntList();
timestamp_ = emptyLongList();
changeset_ = emptyLongList();
uid_ = emptyIntList();
userSid_ = emptyIntList();
visible_ = emptyBooleanList();
}
public static final int VERSION_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.IntList version_;
/**
* repeated int32 version = 1 [packed = true];
*/
public java.util.List
getVersionList() {
return version_;
}
/**
* repeated int32 version = 1 [packed = true];
*/
public int getVersionCount() {
return version_.size();
}
/**
* repeated int32 version = 1 [packed = true];
*/
public int getVersion(int index) {
return version_.getInt(index);
}
private int versionMemoizedSerializedSize = -1;
private void ensureVersionIsMutable() {
if (!version_.isModifiable()) {
version_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(version_);
}
}
/**
* repeated int32 version = 1 [packed = true];
*/
private void setVersion(
int index, int value) {
ensureVersionIsMutable();
version_.setInt(index, value);
}
/**
* repeated int32 version = 1 [packed = true];
*/
private void addVersion(int value) {
ensureVersionIsMutable();
version_.addInt(value);
}
/**
* repeated int32 version = 1 [packed = true];
*/
private void addAllVersion(
java.lang.Iterable extends java.lang.Integer> values) {
ensureVersionIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, version_);
}
/**
* repeated int32 version = 1 [packed = true];
*/
private void clearVersion() {
version_ = emptyIntList();
}
public static final int TIMESTAMP_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.LongList timestamp_;
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public java.util.List
getTimestampList() {
return timestamp_;
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public int getTimestampCount() {
return timestamp_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public long getTimestamp(int index) {
return timestamp_.getLong(index);
}
private int timestampMemoizedSerializedSize = -1;
private void ensureTimestampIsMutable() {
if (!timestamp_.isModifiable()) {
timestamp_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(timestamp_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
private void setTimestamp(
int index, long value) {
ensureTimestampIsMutable();
timestamp_.setLong(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
private void addTimestamp(long value) {
ensureTimestampIsMutable();
timestamp_.addLong(value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
private void addAllTimestamp(
java.lang.Iterable extends java.lang.Long> values) {
ensureTimestampIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, timestamp_);
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
private void clearTimestamp() {
timestamp_ = emptyLongList();
}
public static final int CHANGESET_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.LongList changeset_;
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public java.util.List
getChangesetList() {
return changeset_;
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public int getChangesetCount() {
return changeset_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public long getChangeset(int index) {
return changeset_.getLong(index);
}
private int changesetMemoizedSerializedSize = -1;
private void ensureChangesetIsMutable() {
if (!changeset_.isModifiable()) {
changeset_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(changeset_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
private void setChangeset(
int index, long value) {
ensureChangesetIsMutable();
changeset_.setLong(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
private void addChangeset(long value) {
ensureChangesetIsMutable();
changeset_.addLong(value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
private void addAllChangeset(
java.lang.Iterable extends java.lang.Long> values) {
ensureChangesetIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, changeset_);
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
private void clearChangeset() {
changeset_ = emptyLongList();
}
public static final int UID_FIELD_NUMBER = 4;
private com.google.protobuf.Internal.IntList uid_;
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public java.util.List
getUidList() {
return uid_;
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public int getUidCount() {
return uid_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public int getUid(int index) {
return uid_.getInt(index);
}
private int uidMemoizedSerializedSize = -1;
private void ensureUidIsMutable() {
if (!uid_.isModifiable()) {
uid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(uid_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
private void setUid(
int index, int value) {
ensureUidIsMutable();
uid_.setInt(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
private void addUid(int value) {
ensureUidIsMutable();
uid_.addInt(value);
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
private void addAllUid(
java.lang.Iterable extends java.lang.Integer> values) {
ensureUidIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, uid_);
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
private void clearUid() {
uid_ = emptyIntList();
}
public static final int USER_SID_FIELD_NUMBER = 5;
private com.google.protobuf.Internal.IntList userSid_;
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public java.util.List
getUserSidList() {
return userSid_;
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public int getUserSidCount() {
return userSid_.size();
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public int getUserSid(int index) {
return userSid_.getInt(index);
}
private int userSidMemoizedSerializedSize = -1;
private void ensureUserSidIsMutable() {
if (!userSid_.isModifiable()) {
userSid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(userSid_);
}
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
private void setUserSid(
int index, int value) {
ensureUserSidIsMutable();
userSid_.setInt(index, value);
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
private void addUserSid(int value) {
ensureUserSidIsMutable();
userSid_.addInt(value);
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
private void addAllUserSid(
java.lang.Iterable extends java.lang.Integer> values) {
ensureUserSidIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, userSid_);
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
private void clearUserSid() {
userSid_ = emptyIntList();
}
public static final int VISIBLE_FIELD_NUMBER = 6;
private com.google.protobuf.Internal.BooleanList visible_;
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public java.util.List
getVisibleList() {
return visible_;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public int getVisibleCount() {
return visible_.size();
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public boolean getVisible(int index) {
return visible_.getBoolean(index);
}
private int visibleMemoizedSerializedSize = -1;
private void ensureVisibleIsMutable() {
if (!visible_.isModifiable()) {
visible_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(visible_);
}
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
private void setVisible(
int index, boolean value) {
ensureVisibleIsMutable();
visible_.setBoolean(index, value);
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
private void addVisible(boolean value) {
ensureVisibleIsMutable();
visible_.addBoolean(value);
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
private void addAllVisible(
java.lang.Iterable extends java.lang.Boolean> values) {
ensureVisibleIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, visible_);
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
private void clearVisible() {
visible_ = emptyBooleanList();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getVersionList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(versionMemoizedSerializedSize);
}
for (int i = 0; i < version_.size(); i++) {
output.writeInt32NoTag(version_.getInt(i));
}
if (getTimestampList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(timestampMemoizedSerializedSize);
}
for (int i = 0; i < timestamp_.size(); i++) {
output.writeSInt64NoTag(timestamp_.getLong(i));
}
if (getChangesetList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(changesetMemoizedSerializedSize);
}
for (int i = 0; i < changeset_.size(); i++) {
output.writeSInt64NoTag(changeset_.getLong(i));
}
if (getUidList().size() > 0) {
output.writeUInt32NoTag(34);
output.writeUInt32NoTag(uidMemoizedSerializedSize);
}
for (int i = 0; i < uid_.size(); i++) {
output.writeSInt32NoTag(uid_.getInt(i));
}
if (getUserSidList().size() > 0) {
output.writeUInt32NoTag(42);
output.writeUInt32NoTag(userSidMemoizedSerializedSize);
}
for (int i = 0; i < userSid_.size(); i++) {
output.writeSInt32NoTag(userSid_.getInt(i));
}
if (getVisibleList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(visibleMemoizedSerializedSize);
}
for (int i = 0; i < visible_.size(); i++) {
output.writeBoolNoTag(visible_.getBoolean(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < version_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(version_.getInt(i));
}
size += dataSize;
if (!getVersionList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
versionMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < timestamp_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(timestamp_.getLong(i));
}
size += dataSize;
if (!getTimestampList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
timestampMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < changeset_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(changeset_.getLong(i));
}
size += dataSize;
if (!getChangesetList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
changesetMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < uid_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt32SizeNoTag(uid_.getInt(i));
}
size += dataSize;
if (!getUidList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
uidMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < userSid_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt32SizeNoTag(userSid_.getInt(i));
}
size += dataSize;
if (!getUserSidList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
userSidMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
dataSize = 1 * getVisibleList().size();
size += dataSize;
if (!getVisibleList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
visibleMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.DenseInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.DenseInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.DenseInfo prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
** Optional metadata that may be included into each primitive. Special dense format used in DenseNodes.
*
*
* Protobuf type {@code OSMPBF.DenseInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.DenseInfo, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.DenseInfo)
crosby.binary.Osmformat.DenseInfoOrBuilder {
// Construct using crosby.binary.Osmformat.DenseInfo.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* repeated int32 version = 1 [packed = true];
*/
public java.util.List
getVersionList() {
return java.util.Collections.unmodifiableList(
instance.getVersionList());
}
/**
* repeated int32 version = 1 [packed = true];
*/
public int getVersionCount() {
return instance.getVersionCount();
}
/**
* repeated int32 version = 1 [packed = true];
*/
public int getVersion(int index) {
return instance.getVersion(index);
}
/**
* repeated int32 version = 1 [packed = true];
*/
public Builder setVersion(
int index, int value) {
copyOnWrite();
instance.setVersion(index, value);
return this;
}
/**
* repeated int32 version = 1 [packed = true];
*/
public Builder addVersion(int value) {
copyOnWrite();
instance.addVersion(value);
return this;
}
/**
* repeated int32 version = 1 [packed = true];
*/
public Builder addAllVersion(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllVersion(values);
return this;
}
/**
* repeated int32 version = 1 [packed = true];
*/
public Builder clearVersion() {
copyOnWrite();
instance.clearVersion();
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public java.util.List
getTimestampList() {
return java.util.Collections.unmodifiableList(
instance.getTimestampList());
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public int getTimestampCount() {
return instance.getTimestampCount();
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public long getTimestamp(int index) {
return instance.getTimestamp(index);
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public Builder setTimestamp(
int index, long value) {
copyOnWrite();
instance.setTimestamp(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public Builder addTimestamp(long value) {
copyOnWrite();
instance.addTimestamp(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public Builder addAllTimestamp(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllTimestamp(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 timestamp = 2 [packed = true];
*/
public Builder clearTimestamp() {
copyOnWrite();
instance.clearTimestamp();
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public java.util.List
getChangesetList() {
return java.util.Collections.unmodifiableList(
instance.getChangesetList());
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public int getChangesetCount() {
return instance.getChangesetCount();
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public long getChangeset(int index) {
return instance.getChangeset(index);
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public Builder setChangeset(
int index, long value) {
copyOnWrite();
instance.setChangeset(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public Builder addChangeset(long value) {
copyOnWrite();
instance.addChangeset(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public Builder addAllChangeset(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllChangeset(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 changeset = 3 [packed = true];
*/
public Builder clearChangeset() {
copyOnWrite();
instance.clearChangeset();
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public java.util.List
getUidList() {
return java.util.Collections.unmodifiableList(
instance.getUidList());
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public int getUidCount() {
return instance.getUidCount();
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public int getUid(int index) {
return instance.getUid(index);
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public Builder setUid(
int index, int value) {
copyOnWrite();
instance.setUid(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public Builder addUid(int value) {
copyOnWrite();
instance.addUid(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public Builder addAllUid(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllUid(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint32 uid = 4 [packed = true];
*/
public Builder clearUid() {
copyOnWrite();
instance.clearUid();
return this;
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public java.util.List
getUserSidList() {
return java.util.Collections.unmodifiableList(
instance.getUserSidList());
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public int getUserSidCount() {
return instance.getUserSidCount();
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public int getUserSid(int index) {
return instance.getUserSid(index);
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public Builder setUserSid(
int index, int value) {
copyOnWrite();
instance.setUserSid(index, value);
return this;
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public Builder addUserSid(int value) {
copyOnWrite();
instance.addUserSid(value);
return this;
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public Builder addAllUserSid(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllUserSid(values);
return this;
}
/**
*
* String IDs for usernames. DELTA coded
*
*
* repeated sint32 user_sid = 5 [packed = true];
*/
public Builder clearUserSid() {
copyOnWrite();
instance.clearUserSid();
return this;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public java.util.List
getVisibleList() {
return java.util.Collections.unmodifiableList(
instance.getVisibleList());
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public int getVisibleCount() {
return instance.getVisibleCount();
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public boolean getVisible(int index) {
return instance.getVisible(index);
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public Builder setVisible(
int index, boolean value) {
copyOnWrite();
instance.setVisible(index, value);
return this;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public Builder addVisible(boolean value) {
copyOnWrite();
instance.addVisible(value);
return this;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public Builder addAllVisible(
java.lang.Iterable extends java.lang.Boolean> values) {
copyOnWrite();
instance.addAllVisible(values);
return this;
}
/**
*
* The visible flag is used to store history information. It indicates that
* the current object version has been created by a delete operation on the
* OSM API.
* When a writer sets this flag, it MUST add a required_features tag with
* value "HistoricalInformation" to the HeaderBlock.
* If this flag is not available for some object it MUST be assumed to be
* true if the file has the required_features tag "HistoricalInformation"
* set.
*
*
* repeated bool visible = 6 [packed = true];
*/
public Builder clearVisible() {
copyOnWrite();
instance.clearVisible();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.DenseInfo)
}
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.DenseInfo();
}
case IS_INITIALIZED: {
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
version_.makeImmutable();
timestamp_.makeImmutable();
changeset_.makeImmutable();
uid_.makeImmutable();
userSid_.makeImmutable();
visible_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.DenseInfo other = (crosby.binary.Osmformat.DenseInfo) arg1;
version_= visitor.visitIntList(version_, other.version_);
timestamp_= visitor.visitLongList(timestamp_, other.timestamp_);
changeset_= visitor.visitLongList(changeset_, other.changeset_);
uid_= visitor.visitIntList(uid_, other.uid_);
userSid_= visitor.visitIntList(userSid_, other.userSid_);
visible_= visitor.visitBooleanList(visible_, other.visible_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
if (!version_.isModifiable()) {
version_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(version_);
}
version_.addInt(input.readInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!version_.isModifiable() && input.getBytesUntilLimit() > 0) {
version_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(version_);
}
while (input.getBytesUntilLimit() > 0) {
version_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
case 16: {
if (!timestamp_.isModifiable()) {
timestamp_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(timestamp_);
}
timestamp_.addLong(input.readSInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!timestamp_.isModifiable() && input.getBytesUntilLimit() > 0) {
timestamp_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(timestamp_);
}
while (input.getBytesUntilLimit() > 0) {
timestamp_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 24: {
if (!changeset_.isModifiable()) {
changeset_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(changeset_);
}
changeset_.addLong(input.readSInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!changeset_.isModifiable() && input.getBytesUntilLimit() > 0) {
changeset_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(changeset_);
}
while (input.getBytesUntilLimit() > 0) {
changeset_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 32: {
if (!uid_.isModifiable()) {
uid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(uid_);
}
uid_.addInt(input.readSInt32());
break;
}
case 34: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!uid_.isModifiable() && input.getBytesUntilLimit() > 0) {
uid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(uid_);
}
while (input.getBytesUntilLimit() > 0) {
uid_.addInt(input.readSInt32());
}
input.popLimit(limit);
break;
}
case 40: {
if (!userSid_.isModifiable()) {
userSid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(userSid_);
}
userSid_.addInt(input.readSInt32());
break;
}
case 42: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!userSid_.isModifiable() && input.getBytesUntilLimit() > 0) {
userSid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(userSid_);
}
while (input.getBytesUntilLimit() > 0) {
userSid_.addInt(input.readSInt32());
}
input.popLimit(limit);
break;
}
case 48: {
if (!visible_.isModifiable()) {
visible_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(visible_);
}
visible_.addBoolean(input.readBool());
break;
}
case 50: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!visible_.isModifiable() && input.getBytesUntilLimit() > 0) {
final int currentSize = visible_.size();
visible_ = visible_.mutableCopyWithCapacity(
currentSize + (length/1));
}
while (input.getBytesUntilLimit() > 0) {
visible_.addBoolean(input.readBool());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.DenseInfo.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.DenseInfo)
private static final crosby.binary.Osmformat.DenseInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new DenseInfo();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.DenseInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface ChangeSetOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.ChangeSet)
com.google.protobuf.MessageLiteOrBuilder {
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
boolean hasId();
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
long getId();
}
/**
*
* THIS IS STUB DESIGN FOR CHANGESETS. NOT USED RIGHT NOW.
* TODO: REMOVE THIS?
*
*
* Protobuf type {@code OSMPBF.ChangeSet}
*/
public static final class ChangeSet extends
com.google.protobuf.GeneratedMessageLite<
ChangeSet, ChangeSet.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.ChangeSet)
ChangeSetOrBuilder {
private ChangeSet() {
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
public long getId() {
return id_;
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
private void setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
private void clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, id_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.ChangeSet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.ChangeSet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.ChangeSet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.ChangeSet prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
*
* THIS IS STUB DESIGN FOR CHANGESETS. NOT USED RIGHT NOW.
* TODO: REMOVE THIS?
*
*
* Protobuf type {@code OSMPBF.ChangeSet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.ChangeSet, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.ChangeSet)
crosby.binary.Osmformat.ChangeSetOrBuilder {
// Construct using crosby.binary.Osmformat.ChangeSet.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
public boolean hasId() {
return instance.hasId();
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
public long getId() {
return instance.getId();
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
public Builder setId(long value) {
copyOnWrite();
instance.setId(value);
return this;
}
/**
*
*
* // Parallel arrays.
* repeated uint32 keys = 2 [packed = true]; // String IDs.
* repeated uint32 vals = 3 [packed = true]; // String IDs.
* optional Info info = 4;
*
*
* required int64 id = 1;
*/
public Builder clearId() {
copyOnWrite();
instance.clearId();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.ChangeSet)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.ChangeSet();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasId()) {
return null;
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.ChangeSet other = (crosby.binary.Osmformat.ChangeSet) arg1;
id_ = visitor.visitLong(
hasId(), id_,
other.hasId(), other.id_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.ChangeSet.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.ChangeSet)
private static final crosby.binary.Osmformat.ChangeSet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ChangeSet();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.ChangeSet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface NodeOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.Node)
com.google.protobuf.MessageLiteOrBuilder {
/**
* required sint64 id = 1;
*/
boolean hasId();
/**
* required sint64 id = 1;
*/
long getId();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
java.util.List getKeysList();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
int getKeysCount();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
int getKeys(int index);
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
java.util.List getValsList();
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
int getValsCount();
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
int getVals(int index);
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
boolean hasInfo();
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
crosby.binary.Osmformat.Info getInfo();
/**
* required sint64 lat = 8;
*/
boolean hasLat();
/**
* required sint64 lat = 8;
*/
long getLat();
/**
* required sint64 lon = 9;
*/
boolean hasLon();
/**
* required sint64 lon = 9;
*/
long getLon();
}
/**
* Protobuf type {@code OSMPBF.Node}
*/
public static final class Node extends
com.google.protobuf.GeneratedMessageLite<
Node, Node.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.Node)
NodeOrBuilder {
private Node() {
keys_ = emptyIntList();
vals_ = emptyIntList();
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* required sint64 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required sint64 id = 1;
*/
public long getId() {
return id_;
}
/**
* required sint64 id = 1;
*/
private void setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
}
/**
* required sint64 id = 1;
*/
private void clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
}
public static final int KEYS_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.IntList keys_;
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public java.util.List
getKeysList() {
return keys_;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeysCount() {
return keys_.size();
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeys(int index) {
return keys_.getInt(index);
}
private int keysMemoizedSerializedSize = -1;
private void ensureKeysIsMutable() {
if (!keys_.isModifiable()) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void setKeys(
int index, int value) {
ensureKeysIsMutable();
keys_.setInt(index, value);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void addKeys(int value) {
ensureKeysIsMutable();
keys_.addInt(value);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void addAllKeys(
java.lang.Iterable extends java.lang.Integer> values) {
ensureKeysIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, keys_);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void clearKeys() {
keys_ = emptyIntList();
}
public static final int VALS_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.IntList vals_;
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public java.util.List
getValsList() {
return vals_;
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public int getValsCount() {
return vals_.size();
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public int getVals(int index) {
return vals_.getInt(index);
}
private int valsMemoizedSerializedSize = -1;
private void ensureValsIsMutable() {
if (!vals_.isModifiable()) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
private void setVals(
int index, int value) {
ensureValsIsMutable();
vals_.setInt(index, value);
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
private void addVals(int value) {
ensureValsIsMutable();
vals_.addInt(value);
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
private void addAllVals(
java.lang.Iterable extends java.lang.Integer> values) {
ensureValsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, vals_);
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
private void clearVals() {
vals_ = emptyIntList();
}
public static final int INFO_FIELD_NUMBER = 4;
private crosby.binary.Osmformat.Info info_;
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public crosby.binary.Osmformat.Info getInfo() {
return info_ == null ? crosby.binary.Osmformat.Info.getDefaultInstance() : info_;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
private void setInfo(crosby.binary.Osmformat.Info value) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
bitField0_ |= 0x00000002;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
private void setInfo(
crosby.binary.Osmformat.Info.Builder builderForValue) {
info_ = builderForValue.build();
bitField0_ |= 0x00000002;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
private void mergeInfo(crosby.binary.Osmformat.Info value) {
if (info_ != null &&
info_ != crosby.binary.Osmformat.Info.getDefaultInstance()) {
info_ =
crosby.binary.Osmformat.Info.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
bitField0_ |= 0x00000002;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
private void clearInfo() { info_ = null;
bitField0_ = (bitField0_ & ~0x00000002);
}
public static final int LAT_FIELD_NUMBER = 8;
private long lat_;
/**
* required sint64 lat = 8;
*/
public boolean hasLat() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required sint64 lat = 8;
*/
public long getLat() {
return lat_;
}
/**
* required sint64 lat = 8;
*/
private void setLat(long value) {
bitField0_ |= 0x00000004;
lat_ = value;
}
/**
* required sint64 lat = 8;
*/
private void clearLat() {
bitField0_ = (bitField0_ & ~0x00000004);
lat_ = 0L;
}
public static final int LON_FIELD_NUMBER = 9;
private long lon_;
/**
* required sint64 lon = 9;
*/
public boolean hasLon() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required sint64 lon = 9;
*/
public long getLon() {
return lon_;
}
/**
* required sint64 lon = 9;
*/
private void setLon(long value) {
bitField0_ |= 0x00000008;
lon_ = value;
}
/**
* required sint64 lon = 9;
*/
private void clearLon() {
bitField0_ = (bitField0_ & ~0x00000008);
lon_ = 0L;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeSInt64(1, id_);
}
if (getKeysList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(keysMemoizedSerializedSize);
}
for (int i = 0; i < keys_.size(); i++) {
output.writeUInt32NoTag(keys_.getInt(i));
}
if (getValsList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(valsMemoizedSerializedSize);
}
for (int i = 0; i < vals_.size(); i++) {
output.writeUInt32NoTag(vals_.getInt(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(4, getInfo());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeSInt64(8, lat_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeSInt64(9, lon_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, id_);
}
{
int dataSize = 0;
for (int i = 0; i < keys_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(keys_.getInt(i));
}
size += dataSize;
if (!getKeysList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
keysMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < vals_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(vals_.getInt(i));
}
size += dataSize;
if (!getValsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
valsMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getInfo());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(8, lat_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(9, lon_);
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.Node parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Node parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Node parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Node parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Node parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Node parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Node parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Node parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Node parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Node parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Node parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Node parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.Node prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.Node}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.Node, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.Node)
crosby.binary.Osmformat.NodeOrBuilder {
// Construct using crosby.binary.Osmformat.Node.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* required sint64 id = 1;
*/
public boolean hasId() {
return instance.hasId();
}
/**
* required sint64 id = 1;
*/
public long getId() {
return instance.getId();
}
/**
* required sint64 id = 1;
*/
public Builder setId(long value) {
copyOnWrite();
instance.setId(value);
return this;
}
/**
* required sint64 id = 1;
*/
public Builder clearId() {
copyOnWrite();
instance.clearId();
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public java.util.List
getKeysList() {
return java.util.Collections.unmodifiableList(
instance.getKeysList());
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeysCount() {
return instance.getKeysCount();
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeys(int index) {
return instance.getKeys(index);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder setKeys(
int index, int value) {
copyOnWrite();
instance.setKeys(index, value);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder addKeys(int value) {
copyOnWrite();
instance.addKeys(value);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder addAllKeys(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllKeys(values);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder clearKeys() {
copyOnWrite();
instance.clearKeys();
return this;
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public java.util.List
getValsList() {
return java.util.Collections.unmodifiableList(
instance.getValsList());
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public int getValsCount() {
return instance.getValsCount();
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public int getVals(int index) {
return instance.getVals(index);
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public Builder setVals(
int index, int value) {
copyOnWrite();
instance.setVals(index, value);
return this;
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public Builder addVals(int value) {
copyOnWrite();
instance.addVals(value);
return this;
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public Builder addAllVals(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllVals(values);
return this;
}
/**
*
* String IDs.
*
*
* repeated uint32 vals = 3 [packed = true];
*/
public Builder clearVals() {
copyOnWrite();
instance.clearVals();
return this;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public boolean hasInfo() {
return instance.hasInfo();
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public crosby.binary.Osmformat.Info getInfo() {
return instance.getInfo();
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public Builder setInfo(crosby.binary.Osmformat.Info value) {
copyOnWrite();
instance.setInfo(value);
return this;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public Builder setInfo(
crosby.binary.Osmformat.Info.Builder builderForValue) {
copyOnWrite();
instance.setInfo(builderForValue);
return this;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public Builder mergeInfo(crosby.binary.Osmformat.Info value) {
copyOnWrite();
instance.mergeInfo(value);
return this;
}
/**
*
* May be omitted in omitmeta
*
*
* optional .OSMPBF.Info info = 4;
*/
public Builder clearInfo() { copyOnWrite();
instance.clearInfo();
return this;
}
/**
* required sint64 lat = 8;
*/
public boolean hasLat() {
return instance.hasLat();
}
/**
* required sint64 lat = 8;
*/
public long getLat() {
return instance.getLat();
}
/**
* required sint64 lat = 8;
*/
public Builder setLat(long value) {
copyOnWrite();
instance.setLat(value);
return this;
}
/**
* required sint64 lat = 8;
*/
public Builder clearLat() {
copyOnWrite();
instance.clearLat();
return this;
}
/**
* required sint64 lon = 9;
*/
public boolean hasLon() {
return instance.hasLon();
}
/**
* required sint64 lon = 9;
*/
public long getLon() {
return instance.getLon();
}
/**
* required sint64 lon = 9;
*/
public Builder setLon(long value) {
copyOnWrite();
instance.setLon(value);
return this;
}
/**
* required sint64 lon = 9;
*/
public Builder clearLon() {
copyOnWrite();
instance.clearLon();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.Node)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.Node();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasId()) {
return null;
}
if (!hasLat()) {
return null;
}
if (!hasLon()) {
return null;
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
keys_.makeImmutable();
vals_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.Node other = (crosby.binary.Osmformat.Node) arg1;
id_ = visitor.visitLong(
hasId(), id_,
other.hasId(), other.id_);
keys_= visitor.visitIntList(keys_, other.keys_);
vals_= visitor.visitIntList(vals_, other.vals_);
info_ = visitor.visitMessage(info_, other.info_);
lat_ = visitor.visitLong(
hasLat(), lat_,
other.hasLat(), other.lat_);
lon_ = visitor.visitLong(
hasLon(), lon_,
other.hasLon(), other.lon_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readSInt64();
break;
}
case 16: {
if (!keys_.isModifiable()) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
keys_.addInt(input.readUInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!keys_.isModifiable() && input.getBytesUntilLimit() > 0) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
while (input.getBytesUntilLimit() > 0) {
keys_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 24: {
if (!vals_.isModifiable()) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
vals_.addInt(input.readUInt32());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!vals_.isModifiable() && input.getBytesUntilLimit() > 0) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
while (input.getBytesUntilLimit() > 0) {
vals_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 34: {
crosby.binary.Osmformat.Info.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(crosby.binary.Osmformat.Info.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 64: {
bitField0_ |= 0x00000004;
lat_ = input.readSInt64();
break;
}
case 72: {
bitField0_ |= 0x00000008;
lon_ = input.readSInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.Node.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.Node)
private static final crosby.binary.Osmformat.Node DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Node();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.Node getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface DenseNodesOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.DenseNodes)
com.google.protobuf.MessageLiteOrBuilder {
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
java.util.List getIdList();
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
int getIdCount();
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
long getId(int index);
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
boolean hasDenseinfo();
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
crosby.binary.Osmformat.DenseInfo getDenseinfo();
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
java.util.List getLatList();
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
int getLatCount();
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
long getLat(int index);
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
java.util.List getLonList();
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
int getLonCount();
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
long getLon(int index);
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
java.util.List getKeysValsList();
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
int getKeysValsCount();
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
int getKeysVals(int index);
}
/**
* Protobuf type {@code OSMPBF.DenseNodes}
*/
public static final class DenseNodes extends
com.google.protobuf.GeneratedMessageLite<
DenseNodes, DenseNodes.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.DenseNodes)
DenseNodesOrBuilder {
private DenseNodes() {
id_ = emptyLongList();
lat_ = emptyLongList();
lon_ = emptyLongList();
keysVals_ = emptyIntList();
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.LongList id_;
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public java.util.List
getIdList() {
return id_;
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public int getIdCount() {
return id_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public long getId(int index) {
return id_.getLong(index);
}
private int idMemoizedSerializedSize = -1;
private void ensureIdIsMutable() {
if (!id_.isModifiable()) {
id_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(id_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
private void setId(
int index, long value) {
ensureIdIsMutable();
id_.setLong(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
private void addId(long value) {
ensureIdIsMutable();
id_.addLong(value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
private void addAllId(
java.lang.Iterable extends java.lang.Long> values) {
ensureIdIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, id_);
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
private void clearId() {
id_ = emptyLongList();
}
public static final int DENSEINFO_FIELD_NUMBER = 5;
private crosby.binary.Osmformat.DenseInfo denseinfo_;
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public boolean hasDenseinfo() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public crosby.binary.Osmformat.DenseInfo getDenseinfo() {
return denseinfo_ == null ? crosby.binary.Osmformat.DenseInfo.getDefaultInstance() : denseinfo_;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
private void setDenseinfo(crosby.binary.Osmformat.DenseInfo value) {
if (value == null) {
throw new NullPointerException();
}
denseinfo_ = value;
bitField0_ |= 0x00000001;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
private void setDenseinfo(
crosby.binary.Osmformat.DenseInfo.Builder builderForValue) {
denseinfo_ = builderForValue.build();
bitField0_ |= 0x00000001;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
private void mergeDenseinfo(crosby.binary.Osmformat.DenseInfo value) {
if (denseinfo_ != null &&
denseinfo_ != crosby.binary.Osmformat.DenseInfo.getDefaultInstance()) {
denseinfo_ =
crosby.binary.Osmformat.DenseInfo.newBuilder(denseinfo_).mergeFrom(value).buildPartial();
} else {
denseinfo_ = value;
}
bitField0_ |= 0x00000001;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
private void clearDenseinfo() { denseinfo_ = null;
bitField0_ = (bitField0_ & ~0x00000001);
}
public static final int LAT_FIELD_NUMBER = 8;
private com.google.protobuf.Internal.LongList lat_;
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public java.util.List
getLatList() {
return lat_;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public int getLatCount() {
return lat_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public long getLat(int index) {
return lat_.getLong(index);
}
private int latMemoizedSerializedSize = -1;
private void ensureLatIsMutable() {
if (!lat_.isModifiable()) {
lat_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(lat_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
private void setLat(
int index, long value) {
ensureLatIsMutable();
lat_.setLong(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
private void addLat(long value) {
ensureLatIsMutable();
lat_.addLong(value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
private void addAllLat(
java.lang.Iterable extends java.lang.Long> values) {
ensureLatIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, lat_);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
private void clearLat() {
lat_ = emptyLongList();
}
public static final int LON_FIELD_NUMBER = 9;
private com.google.protobuf.Internal.LongList lon_;
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public java.util.List
getLonList() {
return lon_;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public int getLonCount() {
return lon_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public long getLon(int index) {
return lon_.getLong(index);
}
private int lonMemoizedSerializedSize = -1;
private void ensureLonIsMutable() {
if (!lon_.isModifiable()) {
lon_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(lon_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
private void setLon(
int index, long value) {
ensureLonIsMutable();
lon_.setLong(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
private void addLon(long value) {
ensureLonIsMutable();
lon_.addLong(value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
private void addAllLon(
java.lang.Iterable extends java.lang.Long> values) {
ensureLonIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, lon_);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
private void clearLon() {
lon_ = emptyLongList();
}
public static final int KEYS_VALS_FIELD_NUMBER = 10;
private com.google.protobuf.Internal.IntList keysVals_;
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public java.util.List
getKeysValsList() {
return keysVals_;
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public int getKeysValsCount() {
return keysVals_.size();
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public int getKeysVals(int index) {
return keysVals_.getInt(index);
}
private int keysValsMemoizedSerializedSize = -1;
private void ensureKeysValsIsMutable() {
if (!keysVals_.isModifiable()) {
keysVals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keysVals_);
}
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
private void setKeysVals(
int index, int value) {
ensureKeysValsIsMutable();
keysVals_.setInt(index, value);
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
private void addKeysVals(int value) {
ensureKeysValsIsMutable();
keysVals_.addInt(value);
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
private void addAllKeysVals(
java.lang.Iterable extends java.lang.Integer> values) {
ensureKeysValsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, keysVals_);
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
private void clearKeysVals() {
keysVals_ = emptyIntList();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getIdList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(idMemoizedSerializedSize);
}
for (int i = 0; i < id_.size(); i++) {
output.writeSInt64NoTag(id_.getLong(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(5, getDenseinfo());
}
if (getLatList().size() > 0) {
output.writeUInt32NoTag(66);
output.writeUInt32NoTag(latMemoizedSerializedSize);
}
for (int i = 0; i < lat_.size(); i++) {
output.writeSInt64NoTag(lat_.getLong(i));
}
if (getLonList().size() > 0) {
output.writeUInt32NoTag(74);
output.writeUInt32NoTag(lonMemoizedSerializedSize);
}
for (int i = 0; i < lon_.size(); i++) {
output.writeSInt64NoTag(lon_.getLong(i));
}
if (getKeysValsList().size() > 0) {
output.writeUInt32NoTag(82);
output.writeUInt32NoTag(keysValsMemoizedSerializedSize);
}
for (int i = 0; i < keysVals_.size(); i++) {
output.writeInt32NoTag(keysVals_.getInt(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < id_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(id_.getLong(i));
}
size += dataSize;
if (!getIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
idMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getDenseinfo());
}
{
int dataSize = 0;
for (int i = 0; i < lat_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(lat_.getLong(i));
}
size += dataSize;
if (!getLatList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
latMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < lon_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(lon_.getLong(i));
}
size += dataSize;
if (!getLonList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
lonMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < keysVals_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(keysVals_.getInt(i));
}
size += dataSize;
if (!getKeysValsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
keysValsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseNodes parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.DenseNodes parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.DenseNodes parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.DenseNodes prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.DenseNodes}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.DenseNodes, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.DenseNodes)
crosby.binary.Osmformat.DenseNodesOrBuilder {
// Construct using crosby.binary.Osmformat.DenseNodes.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public java.util.List
getIdList() {
return java.util.Collections.unmodifiableList(
instance.getIdList());
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public int getIdCount() {
return instance.getIdCount();
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public long getId(int index) {
return instance.getId(index);
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public Builder setId(
int index, long value) {
copyOnWrite();
instance.setId(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public Builder addId(long value) {
copyOnWrite();
instance.addId(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public Builder addAllId(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllId(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 id = 1 [packed = true];
*/
public Builder clearId() {
copyOnWrite();
instance.clearId();
return this;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public boolean hasDenseinfo() {
return instance.hasDenseinfo();
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public crosby.binary.Osmformat.DenseInfo getDenseinfo() {
return instance.getDenseinfo();
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public Builder setDenseinfo(crosby.binary.Osmformat.DenseInfo value) {
copyOnWrite();
instance.setDenseinfo(value);
return this;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public Builder setDenseinfo(
crosby.binary.Osmformat.DenseInfo.Builder builderForValue) {
copyOnWrite();
instance.setDenseinfo(builderForValue);
return this;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public Builder mergeDenseinfo(crosby.binary.Osmformat.DenseInfo value) {
copyOnWrite();
instance.mergeDenseinfo(value);
return this;
}
/**
*
*repeated Info info = 4;
*
*
* optional .OSMPBF.DenseInfo denseinfo = 5;
*/
public Builder clearDenseinfo() { copyOnWrite();
instance.clearDenseinfo();
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public java.util.List
getLatList() {
return java.util.Collections.unmodifiableList(
instance.getLatList());
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public int getLatCount() {
return instance.getLatCount();
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public long getLat(int index) {
return instance.getLat(index);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public Builder setLat(
int index, long value) {
copyOnWrite();
instance.setLat(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public Builder addLat(long value) {
copyOnWrite();
instance.addLat(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public Builder addAllLat(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllLat(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lat = 8 [packed = true];
*/
public Builder clearLat() {
copyOnWrite();
instance.clearLat();
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public java.util.List
getLonList() {
return java.util.Collections.unmodifiableList(
instance.getLonList());
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public int getLonCount() {
return instance.getLonCount();
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public long getLon(int index) {
return instance.getLon(index);
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public Builder setLon(
int index, long value) {
copyOnWrite();
instance.setLon(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public Builder addLon(long value) {
copyOnWrite();
instance.addLon(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public Builder addAllLon(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllLon(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 lon = 9 [packed = true];
*/
public Builder clearLon() {
copyOnWrite();
instance.clearLon();
return this;
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public java.util.List
getKeysValsList() {
return java.util.Collections.unmodifiableList(
instance.getKeysValsList());
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public int getKeysValsCount() {
return instance.getKeysValsCount();
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public int getKeysVals(int index) {
return instance.getKeysVals(index);
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public Builder setKeysVals(
int index, int value) {
copyOnWrite();
instance.setKeysVals(index, value);
return this;
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public Builder addKeysVals(int value) {
copyOnWrite();
instance.addKeysVals(value);
return this;
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public Builder addAllKeysVals(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllKeysVals(values);
return this;
}
/**
*
* Special packing of keys and vals into one array. May be empty if all nodes in this block are tagless.
*
*
* repeated int32 keys_vals = 10 [packed = true];
*/
public Builder clearKeysVals() {
copyOnWrite();
instance.clearKeysVals();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.DenseNodes)
}
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.DenseNodes();
}
case IS_INITIALIZED: {
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
id_.makeImmutable();
lat_.makeImmutable();
lon_.makeImmutable();
keysVals_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.DenseNodes other = (crosby.binary.Osmformat.DenseNodes) arg1;
id_= visitor.visitLongList(id_, other.id_);
denseinfo_ = visitor.visitMessage(denseinfo_, other.denseinfo_);
lat_= visitor.visitLongList(lat_, other.lat_);
lon_= visitor.visitLongList(lon_, other.lon_);
keysVals_= visitor.visitIntList(keysVals_, other.keysVals_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
if (!id_.isModifiable()) {
id_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(id_);
}
id_.addLong(input.readSInt64());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!id_.isModifiable() && input.getBytesUntilLimit() > 0) {
id_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(id_);
}
while (input.getBytesUntilLimit() > 0) {
id_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 42: {
crosby.binary.Osmformat.DenseInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = denseinfo_.toBuilder();
}
denseinfo_ = input.readMessage(crosby.binary.Osmformat.DenseInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(denseinfo_);
denseinfo_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 64: {
if (!lat_.isModifiable()) {
lat_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(lat_);
}
lat_.addLong(input.readSInt64());
break;
}
case 66: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!lat_.isModifiable() && input.getBytesUntilLimit() > 0) {
lat_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(lat_);
}
while (input.getBytesUntilLimit() > 0) {
lat_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 72: {
if (!lon_.isModifiable()) {
lon_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(lon_);
}
lon_.addLong(input.readSInt64());
break;
}
case 74: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!lon_.isModifiable() && input.getBytesUntilLimit() > 0) {
lon_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(lon_);
}
while (input.getBytesUntilLimit() > 0) {
lon_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 80: {
if (!keysVals_.isModifiable()) {
keysVals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keysVals_);
}
keysVals_.addInt(input.readInt32());
break;
}
case 82: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!keysVals_.isModifiable() && input.getBytesUntilLimit() > 0) {
keysVals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keysVals_);
}
while (input.getBytesUntilLimit() > 0) {
keysVals_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.DenseNodes.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return (byte) 1;
}
case SET_MEMOIZED_IS_INITIALIZED: {
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.DenseNodes)
private static final crosby.binary.Osmformat.DenseNodes DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new DenseNodes();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.DenseNodes getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface WayOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.Way)
com.google.protobuf.MessageLiteOrBuilder {
/**
* required int64 id = 1;
*/
boolean hasId();
/**
* required int64 id = 1;
*/
long getId();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
java.util.List getKeysList();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
int getKeysCount();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
int getKeys(int index);
/**
* repeated uint32 vals = 3 [packed = true];
*/
java.util.List getValsList();
/**
* repeated uint32 vals = 3 [packed = true];
*/
int getValsCount();
/**
* repeated uint32 vals = 3 [packed = true];
*/
int getVals(int index);
/**
* optional .OSMPBF.Info info = 4;
*/
boolean hasInfo();
/**
* optional .OSMPBF.Info info = 4;
*/
crosby.binary.Osmformat.Info getInfo();
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
java.util.List getRefsList();
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
int getRefsCount();
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
long getRefs(int index);
}
/**
* Protobuf type {@code OSMPBF.Way}
*/
public static final class Way extends
com.google.protobuf.GeneratedMessageLite<
Way, Way.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.Way)
WayOrBuilder {
private Way() {
keys_ = emptyIntList();
vals_ = emptyIntList();
refs_ = emptyLongList();
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* required int64 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 id = 1;
*/
public long getId() {
return id_;
}
/**
* required int64 id = 1;
*/
private void setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
}
/**
* required int64 id = 1;
*/
private void clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
}
public static final int KEYS_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.IntList keys_;
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public java.util.List
getKeysList() {
return keys_;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeysCount() {
return keys_.size();
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeys(int index) {
return keys_.getInt(index);
}
private int keysMemoizedSerializedSize = -1;
private void ensureKeysIsMutable() {
if (!keys_.isModifiable()) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void setKeys(
int index, int value) {
ensureKeysIsMutable();
keys_.setInt(index, value);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void addKeys(int value) {
ensureKeysIsMutable();
keys_.addInt(value);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void addAllKeys(
java.lang.Iterable extends java.lang.Integer> values) {
ensureKeysIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, keys_);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void clearKeys() {
keys_ = emptyIntList();
}
public static final int VALS_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.IntList vals_;
/**
* repeated uint32 vals = 3 [packed = true];
*/
public java.util.List
getValsList() {
return vals_;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getValsCount() {
return vals_.size();
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getVals(int index) {
return vals_.getInt(index);
}
private int valsMemoizedSerializedSize = -1;
private void ensureValsIsMutable() {
if (!vals_.isModifiable()) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void setVals(
int index, int value) {
ensureValsIsMutable();
vals_.setInt(index, value);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void addVals(int value) {
ensureValsIsMutable();
vals_.addInt(value);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void addAllVals(
java.lang.Iterable extends java.lang.Integer> values) {
ensureValsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, vals_);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void clearVals() {
vals_ = emptyIntList();
}
public static final int INFO_FIELD_NUMBER = 4;
private crosby.binary.Osmformat.Info info_;
/**
* optional .OSMPBF.Info info = 4;
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .OSMPBF.Info info = 4;
*/
public crosby.binary.Osmformat.Info getInfo() {
return info_ == null ? crosby.binary.Osmformat.Info.getDefaultInstance() : info_;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void setInfo(crosby.binary.Osmformat.Info value) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
bitField0_ |= 0x00000002;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void setInfo(
crosby.binary.Osmformat.Info.Builder builderForValue) {
info_ = builderForValue.build();
bitField0_ |= 0x00000002;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void mergeInfo(crosby.binary.Osmformat.Info value) {
if (info_ != null &&
info_ != crosby.binary.Osmformat.Info.getDefaultInstance()) {
info_ =
crosby.binary.Osmformat.Info.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
bitField0_ |= 0x00000002;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void clearInfo() { info_ = null;
bitField0_ = (bitField0_ & ~0x00000002);
}
public static final int REFS_FIELD_NUMBER = 8;
private com.google.protobuf.Internal.LongList refs_;
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public java.util.List
getRefsList() {
return refs_;
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public int getRefsCount() {
return refs_.size();
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public long getRefs(int index) {
return refs_.getLong(index);
}
private int refsMemoizedSerializedSize = -1;
private void ensureRefsIsMutable() {
if (!refs_.isModifiable()) {
refs_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(refs_);
}
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
private void setRefs(
int index, long value) {
ensureRefsIsMutable();
refs_.setLong(index, value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
private void addRefs(long value) {
ensureRefsIsMutable();
refs_.addLong(value);
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
private void addAllRefs(
java.lang.Iterable extends java.lang.Long> values) {
ensureRefsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, refs_);
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
private void clearRefs() {
refs_ = emptyLongList();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, id_);
}
if (getKeysList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(keysMemoizedSerializedSize);
}
for (int i = 0; i < keys_.size(); i++) {
output.writeUInt32NoTag(keys_.getInt(i));
}
if (getValsList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(valsMemoizedSerializedSize);
}
for (int i = 0; i < vals_.size(); i++) {
output.writeUInt32NoTag(vals_.getInt(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(4, getInfo());
}
if (getRefsList().size() > 0) {
output.writeUInt32NoTag(66);
output.writeUInt32NoTag(refsMemoizedSerializedSize);
}
for (int i = 0; i < refs_.size(); i++) {
output.writeSInt64NoTag(refs_.getLong(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
{
int dataSize = 0;
for (int i = 0; i < keys_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(keys_.getInt(i));
}
size += dataSize;
if (!getKeysList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
keysMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < vals_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(vals_.getInt(i));
}
size += dataSize;
if (!getValsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
valsMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getInfo());
}
{
int dataSize = 0;
for (int i = 0; i < refs_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(refs_.getLong(i));
}
size += dataSize;
if (!getRefsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
refsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.Way parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Way parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Way parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Way parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Way parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Way parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Way parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Way parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Way parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Way parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Way parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Way parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.Way prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.Way}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.Way, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.Way)
crosby.binary.Osmformat.WayOrBuilder {
// Construct using crosby.binary.Osmformat.Way.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* required int64 id = 1;
*/
public boolean hasId() {
return instance.hasId();
}
/**
* required int64 id = 1;
*/
public long getId() {
return instance.getId();
}
/**
* required int64 id = 1;
*/
public Builder setId(long value) {
copyOnWrite();
instance.setId(value);
return this;
}
/**
* required int64 id = 1;
*/
public Builder clearId() {
copyOnWrite();
instance.clearId();
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public java.util.List
getKeysList() {
return java.util.Collections.unmodifiableList(
instance.getKeysList());
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeysCount() {
return instance.getKeysCount();
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeys(int index) {
return instance.getKeys(index);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder setKeys(
int index, int value) {
copyOnWrite();
instance.setKeys(index, value);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder addKeys(int value) {
copyOnWrite();
instance.addKeys(value);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder addAllKeys(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllKeys(values);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder clearKeys() {
copyOnWrite();
instance.clearKeys();
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public java.util.List
getValsList() {
return java.util.Collections.unmodifiableList(
instance.getValsList());
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getValsCount() {
return instance.getValsCount();
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getVals(int index) {
return instance.getVals(index);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder setVals(
int index, int value) {
copyOnWrite();
instance.setVals(index, value);
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder addVals(int value) {
copyOnWrite();
instance.addVals(value);
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder addAllVals(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllVals(values);
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder clearVals() {
copyOnWrite();
instance.clearVals();
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public boolean hasInfo() {
return instance.hasInfo();
}
/**
* optional .OSMPBF.Info info = 4;
*/
public crosby.binary.Osmformat.Info getInfo() {
return instance.getInfo();
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder setInfo(crosby.binary.Osmformat.Info value) {
copyOnWrite();
instance.setInfo(value);
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder setInfo(
crosby.binary.Osmformat.Info.Builder builderForValue) {
copyOnWrite();
instance.setInfo(builderForValue);
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder mergeInfo(crosby.binary.Osmformat.Info value) {
copyOnWrite();
instance.mergeInfo(value);
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder clearInfo() { copyOnWrite();
instance.clearInfo();
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public java.util.List
getRefsList() {
return java.util.Collections.unmodifiableList(
instance.getRefsList());
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public int getRefsCount() {
return instance.getRefsCount();
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public long getRefs(int index) {
return instance.getRefs(index);
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public Builder setRefs(
int index, long value) {
copyOnWrite();
instance.setRefs(index, value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public Builder addRefs(long value) {
copyOnWrite();
instance.addRefs(value);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public Builder addAllRefs(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllRefs(values);
return this;
}
/**
*
* DELTA coded
*
*
* repeated sint64 refs = 8 [packed = true];
*/
public Builder clearRefs() {
copyOnWrite();
instance.clearRefs();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.Way)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.Way();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasId()) {
return null;
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
keys_.makeImmutable();
vals_.makeImmutable();
refs_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.Way other = (crosby.binary.Osmformat.Way) arg1;
id_ = visitor.visitLong(
hasId(), id_,
other.hasId(), other.id_);
keys_= visitor.visitIntList(keys_, other.keys_);
vals_= visitor.visitIntList(vals_, other.vals_);
info_ = visitor.visitMessage(info_, other.info_);
refs_= visitor.visitLongList(refs_, other.refs_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readInt64();
break;
}
case 16: {
if (!keys_.isModifiable()) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
keys_.addInt(input.readUInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!keys_.isModifiable() && input.getBytesUntilLimit() > 0) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
while (input.getBytesUntilLimit() > 0) {
keys_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 24: {
if (!vals_.isModifiable()) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
vals_.addInt(input.readUInt32());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!vals_.isModifiable() && input.getBytesUntilLimit() > 0) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
while (input.getBytesUntilLimit() > 0) {
vals_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 34: {
crosby.binary.Osmformat.Info.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(crosby.binary.Osmformat.Info.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 64: {
if (!refs_.isModifiable()) {
refs_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(refs_);
}
refs_.addLong(input.readSInt64());
break;
}
case 66: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!refs_.isModifiable() && input.getBytesUntilLimit() > 0) {
refs_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(refs_);
}
while (input.getBytesUntilLimit() > 0) {
refs_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.Way.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.Way)
private static final crosby.binary.Osmformat.Way DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Way();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.Way getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
public interface RelationOrBuilder extends
// @@protoc_insertion_point(interface_extends:OSMPBF.Relation)
com.google.protobuf.MessageLiteOrBuilder {
/**
* required int64 id = 1;
*/
boolean hasId();
/**
* required int64 id = 1;
*/
long getId();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
java.util.List getKeysList();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
int getKeysCount();
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
int getKeys(int index);
/**
* repeated uint32 vals = 3 [packed = true];
*/
java.util.List getValsList();
/**
* repeated uint32 vals = 3 [packed = true];
*/
int getValsCount();
/**
* repeated uint32 vals = 3 [packed = true];
*/
int getVals(int index);
/**
* optional .OSMPBF.Info info = 4;
*/
boolean hasInfo();
/**
* optional .OSMPBF.Info info = 4;
*/
crosby.binary.Osmformat.Info getInfo();
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
java.util.List getRolesSidList();
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
int getRolesSidCount();
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
int getRolesSid(int index);
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
java.util.List getMemidsList();
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
int getMemidsCount();
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
long getMemids(int index);
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
java.util.List getTypesList();
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
int getTypesCount();
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
crosby.binary.Osmformat.Relation.MemberType getTypes(int index);
}
/**
* Protobuf type {@code OSMPBF.Relation}
*/
public static final class Relation extends
com.google.protobuf.GeneratedMessageLite<
Relation, Relation.Builder> implements
// @@protoc_insertion_point(message_implements:OSMPBF.Relation)
RelationOrBuilder {
private Relation() {
keys_ = emptyIntList();
vals_ = emptyIntList();
rolesSid_ = emptyIntList();
memids_ = emptyLongList();
types_ = emptyIntList();
}
/**
* Protobuf enum {@code OSMPBF.Relation.MemberType}
*/
public enum MemberType
implements com.google.protobuf.Internal.EnumLite {
/**
* NODE = 0;
*/
NODE(0),
/**
* WAY = 1;
*/
WAY(1),
/**
* RELATION = 2;
*/
RELATION(2),
;
/**
* NODE = 0;
*/
public static final int NODE_VALUE = 0;
/**
* WAY = 1;
*/
public static final int WAY_VALUE = 1;
/**
* RELATION = 2;
*/
public static final int RELATION_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MemberType valueOf(int value) {
return forNumber(value);
}
public static MemberType forNumber(int value) {
switch (value) {
case 0: return NODE;
case 1: return WAY;
case 2: return RELATION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MemberType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MemberType findValueByNumber(int number) {
return MemberType.forNumber(number);
}
};
private final int value;
private MemberType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:OSMPBF.Relation.MemberType)
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private long id_;
/**
* required int64 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int64 id = 1;
*/
public long getId() {
return id_;
}
/**
* required int64 id = 1;
*/
private void setId(long value) {
bitField0_ |= 0x00000001;
id_ = value;
}
/**
* required int64 id = 1;
*/
private void clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0L;
}
public static final int KEYS_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.IntList keys_;
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public java.util.List
getKeysList() {
return keys_;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeysCount() {
return keys_.size();
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeys(int index) {
return keys_.getInt(index);
}
private int keysMemoizedSerializedSize = -1;
private void ensureKeysIsMutable() {
if (!keys_.isModifiable()) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void setKeys(
int index, int value) {
ensureKeysIsMutable();
keys_.setInt(index, value);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void addKeys(int value) {
ensureKeysIsMutable();
keys_.addInt(value);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void addAllKeys(
java.lang.Iterable extends java.lang.Integer> values) {
ensureKeysIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, keys_);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
private void clearKeys() {
keys_ = emptyIntList();
}
public static final int VALS_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.IntList vals_;
/**
* repeated uint32 vals = 3 [packed = true];
*/
public java.util.List
getValsList() {
return vals_;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getValsCount() {
return vals_.size();
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getVals(int index) {
return vals_.getInt(index);
}
private int valsMemoizedSerializedSize = -1;
private void ensureValsIsMutable() {
if (!vals_.isModifiable()) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void setVals(
int index, int value) {
ensureValsIsMutable();
vals_.setInt(index, value);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void addVals(int value) {
ensureValsIsMutable();
vals_.addInt(value);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void addAllVals(
java.lang.Iterable extends java.lang.Integer> values) {
ensureValsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, vals_);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
private void clearVals() {
vals_ = emptyIntList();
}
public static final int INFO_FIELD_NUMBER = 4;
private crosby.binary.Osmformat.Info info_;
/**
* optional .OSMPBF.Info info = 4;
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .OSMPBF.Info info = 4;
*/
public crosby.binary.Osmformat.Info getInfo() {
return info_ == null ? crosby.binary.Osmformat.Info.getDefaultInstance() : info_;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void setInfo(crosby.binary.Osmformat.Info value) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
bitField0_ |= 0x00000002;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void setInfo(
crosby.binary.Osmformat.Info.Builder builderForValue) {
info_ = builderForValue.build();
bitField0_ |= 0x00000002;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void mergeInfo(crosby.binary.Osmformat.Info value) {
if (info_ != null &&
info_ != crosby.binary.Osmformat.Info.getDefaultInstance()) {
info_ =
crosby.binary.Osmformat.Info.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
bitField0_ |= 0x00000002;
}
/**
* optional .OSMPBF.Info info = 4;
*/
private void clearInfo() { info_ = null;
bitField0_ = (bitField0_ & ~0x00000002);
}
public static final int ROLES_SID_FIELD_NUMBER = 8;
private com.google.protobuf.Internal.IntList rolesSid_;
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public java.util.List
getRolesSidList() {
return rolesSid_;
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public int getRolesSidCount() {
return rolesSid_.size();
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public int getRolesSid(int index) {
return rolesSid_.getInt(index);
}
private int rolesSidMemoizedSerializedSize = -1;
private void ensureRolesSidIsMutable() {
if (!rolesSid_.isModifiable()) {
rolesSid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(rolesSid_);
}
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
private void setRolesSid(
int index, int value) {
ensureRolesSidIsMutable();
rolesSid_.setInt(index, value);
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
private void addRolesSid(int value) {
ensureRolesSidIsMutable();
rolesSid_.addInt(value);
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
private void addAllRolesSid(
java.lang.Iterable extends java.lang.Integer> values) {
ensureRolesSidIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, rolesSid_);
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
private void clearRolesSid() {
rolesSid_ = emptyIntList();
}
public static final int MEMIDS_FIELD_NUMBER = 9;
private com.google.protobuf.Internal.LongList memids_;
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public java.util.List
getMemidsList() {
return memids_;
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public int getMemidsCount() {
return memids_.size();
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public long getMemids(int index) {
return memids_.getLong(index);
}
private int memidsMemoizedSerializedSize = -1;
private void ensureMemidsIsMutable() {
if (!memids_.isModifiable()) {
memids_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(memids_);
}
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
private void setMemids(
int index, long value) {
ensureMemidsIsMutable();
memids_.setLong(index, value);
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
private void addMemids(long value) {
ensureMemidsIsMutable();
memids_.addLong(value);
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
private void addAllMemids(
java.lang.Iterable extends java.lang.Long> values) {
ensureMemidsIsMutable();
com.google.protobuf.AbstractMessageLite.addAll(
values, memids_);
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
private void clearMemids() {
memids_ = emptyLongList();
}
public static final int TYPES_FIELD_NUMBER = 10;
private com.google.protobuf.Internal.IntList types_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, crosby.binary.Osmformat.Relation.MemberType> types_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, crosby.binary.Osmformat.Relation.MemberType>() {
public crosby.binary.Osmformat.Relation.MemberType convert(java.lang.Integer from) {
crosby.binary.Osmformat.Relation.MemberType result = crosby.binary.Osmformat.Relation.MemberType.forNumber(from);
return result == null ? crosby.binary.Osmformat.Relation.MemberType.NODE : result;
}
};
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public java.util.List getTypesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, crosby.binary.Osmformat.Relation.MemberType>(types_, types_converter_);
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public int getTypesCount() {
return types_.size();
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public crosby.binary.Osmformat.Relation.MemberType getTypes(int index) {
return types_converter_.convert(types_.getInt(index));
}
private int typesMemoizedSerializedSize;
private void ensureTypesIsMutable() {
if (!types_.isModifiable()) {
types_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(types_);
}
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
private void setTypes(
int index, crosby.binary.Osmformat.Relation.MemberType value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypesIsMutable();
types_.setInt(index, value.getNumber());
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
private void addTypes(crosby.binary.Osmformat.Relation.MemberType value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypesIsMutable();
types_.addInt(value.getNumber());
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
private void addAllTypes(
java.lang.Iterable extends crosby.binary.Osmformat.Relation.MemberType> values) {
ensureTypesIsMutable();
for (crosby.binary.Osmformat.Relation.MemberType value : values) {
types_.addInt(value.getNumber());
}
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
private void clearTypes() {
types_ = emptyIntList();
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, id_);
}
if (getKeysList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(keysMemoizedSerializedSize);
}
for (int i = 0; i < keys_.size(); i++) {
output.writeUInt32NoTag(keys_.getInt(i));
}
if (getValsList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(valsMemoizedSerializedSize);
}
for (int i = 0; i < vals_.size(); i++) {
output.writeUInt32NoTag(vals_.getInt(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(4, getInfo());
}
if (getRolesSidList().size() > 0) {
output.writeUInt32NoTag(66);
output.writeUInt32NoTag(rolesSidMemoizedSerializedSize);
}
for (int i = 0; i < rolesSid_.size(); i++) {
output.writeInt32NoTag(rolesSid_.getInt(i));
}
if (getMemidsList().size() > 0) {
output.writeUInt32NoTag(74);
output.writeUInt32NoTag(memidsMemoizedSerializedSize);
}
for (int i = 0; i < memids_.size(); i++) {
output.writeSInt64NoTag(memids_.getLong(i));
}
if (getTypesList().size() > 0) {
output.writeUInt32NoTag(82);
output.writeUInt32NoTag(typesMemoizedSerializedSize);
}
for (int i = 0; i < types_.size(); i++) {
output.writeEnumNoTag(types_.getInt(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, id_);
}
{
int dataSize = 0;
for (int i = 0; i < keys_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(keys_.getInt(i));
}
size += dataSize;
if (!getKeysList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
keysMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < vals_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(vals_.getInt(i));
}
size += dataSize;
if (!getValsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
valsMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getInfo());
}
{
int dataSize = 0;
for (int i = 0; i < rolesSid_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(rolesSid_.getInt(i));
}
size += dataSize;
if (!getRolesSidList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
rolesSidMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < memids_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeSInt64SizeNoTag(memids_.getLong(i));
}
size += dataSize;
if (!getMemidsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
memidsMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < types_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(types_.getInt(i));
}
size += dataSize;
if (!getTypesList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}typesMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static crosby.binary.Osmformat.Relation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Relation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Relation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Relation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Relation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data);
}
public static crosby.binary.Osmformat.Relation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, data, extensionRegistry);
}
public static crosby.binary.Osmformat.Relation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Relation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Relation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Relation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return parseDelimitedFrom(DEFAULT_INSTANCE, input, extensionRegistry);
}
public static crosby.binary.Osmformat.Relation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input);
}
public static crosby.binary.Osmformat.Relation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageLite.parseFrom(
DEFAULT_INSTANCE, input, extensionRegistry);
}
public static Builder newBuilder() {
return (Builder) DEFAULT_INSTANCE.createBuilder();
}
public static Builder newBuilder(crosby.binary.Osmformat.Relation prototype) {
return (Builder) DEFAULT_INSTANCE.createBuilder(prototype);
}
/**
* Protobuf type {@code OSMPBF.Relation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageLite.Builder<
crosby.binary.Osmformat.Relation, Builder> implements
// @@protoc_insertion_point(builder_implements:OSMPBF.Relation)
crosby.binary.Osmformat.RelationOrBuilder {
// Construct using crosby.binary.Osmformat.Relation.newBuilder()
private Builder() {
super(DEFAULT_INSTANCE);
}
/**
* required int64 id = 1;
*/
public boolean hasId() {
return instance.hasId();
}
/**
* required int64 id = 1;
*/
public long getId() {
return instance.getId();
}
/**
* required int64 id = 1;
*/
public Builder setId(long value) {
copyOnWrite();
instance.setId(value);
return this;
}
/**
* required int64 id = 1;
*/
public Builder clearId() {
copyOnWrite();
instance.clearId();
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public java.util.List
getKeysList() {
return java.util.Collections.unmodifiableList(
instance.getKeysList());
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeysCount() {
return instance.getKeysCount();
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public int getKeys(int index) {
return instance.getKeys(index);
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder setKeys(
int index, int value) {
copyOnWrite();
instance.setKeys(index, value);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder addKeys(int value) {
copyOnWrite();
instance.addKeys(value);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder addAllKeys(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllKeys(values);
return this;
}
/**
*
* Parallel arrays.
*
*
* repeated uint32 keys = 2 [packed = true];
*/
public Builder clearKeys() {
copyOnWrite();
instance.clearKeys();
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public java.util.List
getValsList() {
return java.util.Collections.unmodifiableList(
instance.getValsList());
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getValsCount() {
return instance.getValsCount();
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public int getVals(int index) {
return instance.getVals(index);
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder setVals(
int index, int value) {
copyOnWrite();
instance.setVals(index, value);
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder addVals(int value) {
copyOnWrite();
instance.addVals(value);
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder addAllVals(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllVals(values);
return this;
}
/**
* repeated uint32 vals = 3 [packed = true];
*/
public Builder clearVals() {
copyOnWrite();
instance.clearVals();
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public boolean hasInfo() {
return instance.hasInfo();
}
/**
* optional .OSMPBF.Info info = 4;
*/
public crosby.binary.Osmformat.Info getInfo() {
return instance.getInfo();
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder setInfo(crosby.binary.Osmformat.Info value) {
copyOnWrite();
instance.setInfo(value);
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder setInfo(
crosby.binary.Osmformat.Info.Builder builderForValue) {
copyOnWrite();
instance.setInfo(builderForValue);
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder mergeInfo(crosby.binary.Osmformat.Info value) {
copyOnWrite();
instance.mergeInfo(value);
return this;
}
/**
* optional .OSMPBF.Info info = 4;
*/
public Builder clearInfo() { copyOnWrite();
instance.clearInfo();
return this;
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public java.util.List
getRolesSidList() {
return java.util.Collections.unmodifiableList(
instance.getRolesSidList());
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public int getRolesSidCount() {
return instance.getRolesSidCount();
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public int getRolesSid(int index) {
return instance.getRolesSid(index);
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public Builder setRolesSid(
int index, int value) {
copyOnWrite();
instance.setRolesSid(index, value);
return this;
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public Builder addRolesSid(int value) {
copyOnWrite();
instance.addRolesSid(value);
return this;
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public Builder addAllRolesSid(
java.lang.Iterable extends java.lang.Integer> values) {
copyOnWrite();
instance.addAllRolesSid(values);
return this;
}
/**
*
* Parallel arrays
*
*
* repeated int32 roles_sid = 8 [packed = true];
*/
public Builder clearRolesSid() {
copyOnWrite();
instance.clearRolesSid();
return this;
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public java.util.List
getMemidsList() {
return java.util.Collections.unmodifiableList(
instance.getMemidsList());
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public int getMemidsCount() {
return instance.getMemidsCount();
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public long getMemids(int index) {
return instance.getMemids(index);
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public Builder setMemids(
int index, long value) {
copyOnWrite();
instance.setMemids(index, value);
return this;
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public Builder addMemids(long value) {
copyOnWrite();
instance.addMemids(value);
return this;
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public Builder addAllMemids(
java.lang.Iterable extends java.lang.Long> values) {
copyOnWrite();
instance.addAllMemids(values);
return this;
}
/**
*
* DELTA encoded
*
*
* repeated sint64 memids = 9 [packed = true];
*/
public Builder clearMemids() {
copyOnWrite();
instance.clearMemids();
return this;
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public java.util.List getTypesList() {
return instance.getTypesList();
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public int getTypesCount() {
return instance.getTypesCount();
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public crosby.binary.Osmformat.Relation.MemberType getTypes(int index) {
return instance.getTypes(index);
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public Builder setTypes(
int index, crosby.binary.Osmformat.Relation.MemberType value) {
copyOnWrite();
instance.setTypes(index, value);
return this;
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public Builder addTypes(crosby.binary.Osmformat.Relation.MemberType value) {
copyOnWrite();
instance.addTypes(value);
return this;
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public Builder addAllTypes(
java.lang.Iterable extends crosby.binary.Osmformat.Relation.MemberType> values) {
copyOnWrite();
instance.addAllTypes(values); return this;
}
/**
* repeated .OSMPBF.Relation.MemberType types = 10 [packed = true];
*/
public Builder clearTypes() {
copyOnWrite();
instance.clearTypes();
return this;
}
// @@protoc_insertion_point(builder_scope:OSMPBF.Relation)
}
private byte memoizedIsInitialized = 2;
@java.lang.SuppressWarnings({"unchecked", "fallthrough"})
protected final java.lang.Object dynamicMethod(
com.google.protobuf.GeneratedMessageLite.MethodToInvoke method,
java.lang.Object arg0, java.lang.Object arg1) {
switch (method) {
case NEW_MUTABLE_INSTANCE: {
return new crosby.binary.Osmformat.Relation();
}
case IS_INITIALIZED: {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return DEFAULT_INSTANCE;
if (isInitialized == 0) return null;
boolean shouldMemoize = ((Boolean) arg0).booleanValue();
if (!hasId()) {
return null;
}
return DEFAULT_INSTANCE;
}
case MAKE_IMMUTABLE: {
keys_.makeImmutable();
vals_.makeImmutable();
rolesSid_.makeImmutable();
memids_.makeImmutable();
types_.makeImmutable();
return null;
}
case NEW_BUILDER: {
return new Builder();
}
case VISIT: {
Visitor visitor = (Visitor) arg0;
crosby.binary.Osmformat.Relation other = (crosby.binary.Osmformat.Relation) arg1;
id_ = visitor.visitLong(
hasId(), id_,
other.hasId(), other.id_);
keys_= visitor.visitIntList(keys_, other.keys_);
vals_= visitor.visitIntList(vals_, other.vals_);
info_ = visitor.visitMessage(info_, other.info_);
rolesSid_= visitor.visitIntList(rolesSid_, other.rolesSid_);
memids_= visitor.visitLongList(memids_, other.memids_);
types_= visitor.visitIntList(types_, other.types_);
if (visitor == com.google.protobuf.GeneratedMessageLite.MergeFromVisitor
.INSTANCE) {
bitField0_ |= other.bitField0_;
}
return this;
}
case MERGE_FROM_STREAM: {
com.google.protobuf.CodedInputStream input =
(com.google.protobuf.CodedInputStream) arg0;
com.google.protobuf.ExtensionRegistryLite extensionRegistry =
(com.google.protobuf.ExtensionRegistryLite) arg1;
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(tag, input)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readInt64();
break;
}
case 16: {
if (!keys_.isModifiable()) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
keys_.addInt(input.readUInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!keys_.isModifiable() && input.getBytesUntilLimit() > 0) {
keys_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(keys_);
}
while (input.getBytesUntilLimit() > 0) {
keys_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 24: {
if (!vals_.isModifiable()) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
vals_.addInt(input.readUInt32());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!vals_.isModifiable() && input.getBytesUntilLimit() > 0) {
vals_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(vals_);
}
while (input.getBytesUntilLimit() > 0) {
vals_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 34: {
crosby.binary.Osmformat.Info.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(crosby.binary.Osmformat.Info.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 64: {
if (!rolesSid_.isModifiable()) {
rolesSid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(rolesSid_);
}
rolesSid_.addInt(input.readInt32());
break;
}
case 66: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!rolesSid_.isModifiable() && input.getBytesUntilLimit() > 0) {
rolesSid_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(rolesSid_);
}
while (input.getBytesUntilLimit() > 0) {
rolesSid_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
case 72: {
if (!memids_.isModifiable()) {
memids_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(memids_);
}
memids_.addLong(input.readSInt64());
break;
}
case 74: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!memids_.isModifiable() && input.getBytesUntilLimit() > 0) {
memids_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(memids_);
}
while (input.getBytesUntilLimit() > 0) {
memids_.addLong(input.readSInt64());
}
input.popLimit(limit);
break;
}
case 80: {
if (!types_.isModifiable()) {
types_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(types_);
}
int rawValue = input.readEnum();
crosby.binary.Osmformat.Relation.MemberType value = crosby.binary.Osmformat.Relation.MemberType.forNumber(rawValue);
if (value == null) {
super.mergeVarintField(10, rawValue);
} else {
types_.addInt(rawValue);
}
break;
}
case 82: {
if (!types_.isModifiable()) {
types_ =
com.google.protobuf.GeneratedMessageLite.mutableCopy(types_);
}
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
crosby.binary.Osmformat.Relation.MemberType value = crosby.binary.Osmformat.Relation.MemberType.forNumber(rawValue);
if (value == null) {
super.mergeVarintField(10, rawValue);
} else {
types_.addInt(rawValue);
}
}
input.popLimit(oldLimit);
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw new RuntimeException(e.setUnfinishedMessage(this));
} catch (java.io.IOException e) {
throw new RuntimeException(
new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this));
} finally {
}
}
// fall through
case GET_DEFAULT_INSTANCE: {
return DEFAULT_INSTANCE;
}
case GET_PARSER: {
if (PARSER == null) { synchronized (crosby.binary.Osmformat.Relation.class) {
if (PARSER == null) {
PARSER = new DefaultInstanceBasedParser(DEFAULT_INSTANCE);
}
}
}
return PARSER;
}
case GET_MEMOIZED_IS_INITIALIZED: {
return memoizedIsInitialized;
}
case SET_MEMOIZED_IS_INITIALIZED: {
memoizedIsInitialized = (byte) (arg0 == null ? 0 : 1);
return null;
}
}
throw new UnsupportedOperationException();
}
// @@protoc_insertion_point(class_scope:OSMPBF.Relation)
private static final crosby.binary.Osmformat.Relation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Relation();
DEFAULT_INSTANCE.makeImmutable();
}
public static crosby.binary.Osmformat.Relation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static volatile com.google.protobuf.Parser PARSER;
public static com.google.protobuf.Parser parser() {
return DEFAULT_INSTANCE.getParserForType();
}
}
static {
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy