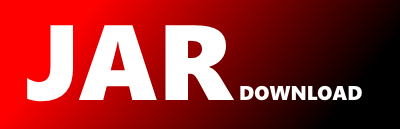
de.be4.classicalb.core.parser.FileSearchPathProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bparser Show documentation
Show all versions of bparser Show documentation
Part of the ProB Parser library
The newest version!
package de.be4.classicalb.core.parser;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
public class FileSearchPathProvider implements Iterable {
private final String fileName;
private final ArrayList searchPath = new ArrayList<>();
public FileSearchPathProvider(String fileName) {
this(".", fileName);
}
public FileSearchPathProvider(String prefix, String fileName) {
this(prefix, fileName, Collections.emptyList());
}
public FileSearchPathProvider(String prefix, String fileName, List paths) {
this.fileName = fileName;
searchPath.add(prefix);
searchPath.addAll(paths);
searchPath.addAll(getLibraryPath());
}
private List getLibraryPath() {
// User provided stdlib search path
final String stdlib = System.getProperty("prob.stdlib");
if (stdlib != null) {
return Arrays.asList(stdlib.split(File.pathSeparator));
} else {
return Collections.singletonList("." + File.separator + "stdlib");
}
}
@Override
public Iterator iterator() {
File file = new File(this.fileName);
if (file.isAbsolute()) {
// If the given file name is absolute, return it unchanged.
// This case must be handled explicitly,
// because the File(File, String) constructor always interprets the second path as relative,
// even if it's an absolute path.
return Collections.singletonList(file).iterator();
}
return this.searchPath.stream()
.map(base -> new File(base, this.getFilename()))
.iterator();
}
public int size() {
return this.searchPath.size();
}
public String getFilename() {
return fileName;
}
public File resolve() throws IOException {
for (File f : this) {
if (f.exists() && f.isFile()) {
return f.getCanonicalFile();
}
}
throw new FileNotFoundException("did not find: " + fileName );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy